How to optimize polling in frontend???
any advice will be helpful ??
5 Replies
cache? long polling? using websockets? like always it depends, you gotta give more context so we can actually help
i am using long polling and the request are hitting the server but the server is sending 404 not found but after 5-6 request server send a 200 request with all the data
so i don't if this is the problem of the frontend or api
and here is the code that i am using for polling
import { Injectable } from '@angular/core';
import {catchError, Observable, Subject, switchMap, takeUntil, tap, throwError, timeout, timer} from 'rxjs';
import {HttpClient, HttpParams} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class LongPollingService {
private baseUrl = 'https://localhost:44311/api/services';
private stopPolling$ = new Subject<void>();
private pollingInterval = 10000; // Retry interval if connection fails or times out
private connectionTimeout = 60000; // 1 minute timeout for each polling request
constructor(private http: HttpClient) { }
/**
* Start long polling for updates with GET request
* @param endpoint The API endpoint path
* @param queryParams Optional query parameters
* @returns Observable that emits server updates
*/
startPolling<T>(endpoint: string, queryParams?: Record<string, string>): Observable<T> {
// Create full URL
const url = `${this.baseUrl}/${endpoint}`;
// Reset any existing polling
this.stopPolling();
// Start polling with interval retry for connection issues
return timer(0, this.pollingInterval).pipe(
switchMap(() => this.makePollingRequest<T>(url, queryParams)),
takeUntil(this.stopPolling$)
);
}
import { Injectable } from '@angular/core';
import {catchError, Observable, Subject, switchMap, takeUntil, tap, throwError, timeout, timer} from 'rxjs';
import {HttpClient, HttpParams} from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class LongPollingService {
private baseUrl = 'https://localhost:44311/api/services';
private stopPolling$ = new Subject<void>();
private pollingInterval = 10000; // Retry interval if connection fails or times out
private connectionTimeout = 60000; // 1 minute timeout for each polling request
constructor(private http: HttpClient) { }
/**
* Start long polling for updates with GET request
* @param endpoint The API endpoint path
* @param queryParams Optional query parameters
* @returns Observable that emits server updates
*/
startPolling<T>(endpoint: string, queryParams?: Record<string, string>): Observable<T> {
// Create full URL
const url = `${this.baseUrl}/${endpoint}`;
// Reset any existing polling
this.stopPolling();
// Start polling with interval retry for connection issues
return timer(0, this.pollingInterval).pipe(
switchMap(() => this.makePollingRequest<T>(url, queryParams)),
takeUntil(this.stopPolling$)
);
}
/**
* Stop the current polling
*/
stopPolling(): void {
this.stopPolling$.next();
}
/**
* Make a single long polling request with GET
*/
private makePollingRequest<T>(url: string, queryParams?: Record<string, string>): Observable<T> {
let params = new HttpParams();
if (queryParams) {
Object.keys(queryParams).forEach(key => {
params = params.set(key, queryParams[key]);
});
}
return this.http.get<T>(url, {
params: params
}).pipe(
timeout(this.connectionTimeout),
tap((response: T) => console.log('Polling response received:', response)),
catchError((error: any) => {
console.error('Polling error:', error);
return throwError(() => error);
})
);
}
/**
* Stop the current polling
*/
stopPolling(): void {
this.stopPolling$.next();
}
/**
* Make a single long polling request with GET
*/
private makePollingRequest<T>(url: string, queryParams?: Record<string, string>): Observable<T> {
let params = new HttpParams();
if (queryParams) {
Object.keys(queryParams).forEach(key => {
params = params.set(key, queryParams[key]);
});
}
return this.http.get<T>(url, {
params: params
}).pipe(
timeout(this.connectionTimeout),
tap((response: T) => console.log('Polling response received:', response)),
catchError((error: any) => {
console.error('Polling error:', error);
return throwError(() => error);
})
);
}
/**
* Get batch workflow step response via long polling
* @param batchId The batch ID to monitor
* @param responseKey The UUID response key
* @returns Observable that emits workflow step response updates
*/
getProductBatchWorkflowStepResponse(responseKey: string, batchId?: number, ): Observable<any> {
const endpoint = 'ProductionCycle/WorkflowEvent/GetProductBatchWorkflowStepResponse';
const queryParams: any = { responseKey };
if (batchId !== undefined) {
queryParams.batchId = batchId.toString();
}
return this.startPolling(endpoint, queryParams);
}
}
/**
* Get batch workflow step response via long polling
* @param batchId The batch ID to monitor
* @param responseKey The UUID response key
* @returns Observable that emits workflow step response updates
*/
getProductBatchWorkflowStepResponse(responseKey: string, batchId?: number, ): Observable<any> {
const endpoint = 'ProductionCycle/WorkflowEvent/GetProductBatchWorkflowStepResponse';
const queryParams: any = { responseKey };
if (batchId !== undefined) {
queryParams.batchId = batchId.toString();
}
return this.startPolling(endpoint, queryParams);
}
}
here is the picture of how it takes 2-3 request before getting 200 from the api
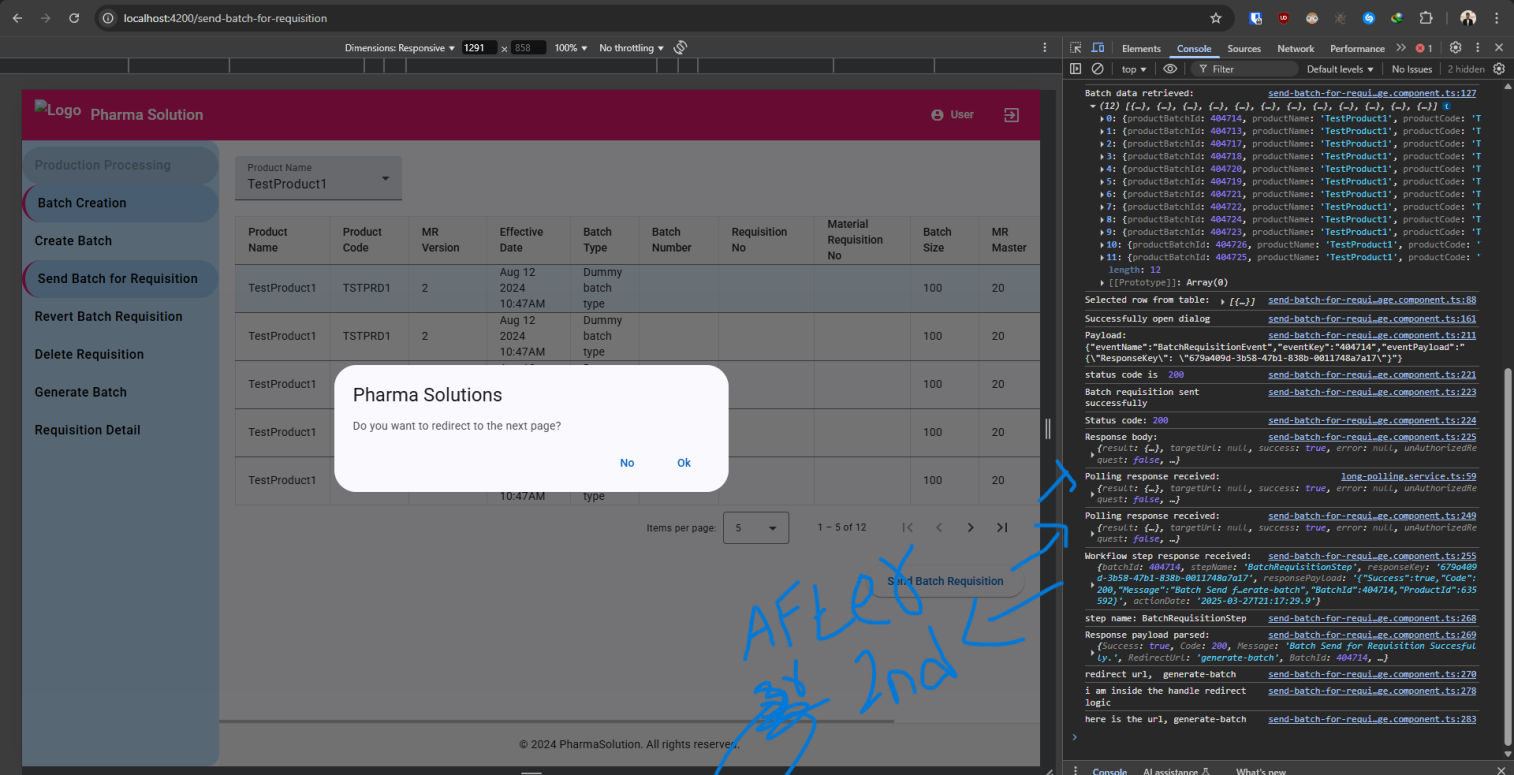
and here is the ss of the network tab
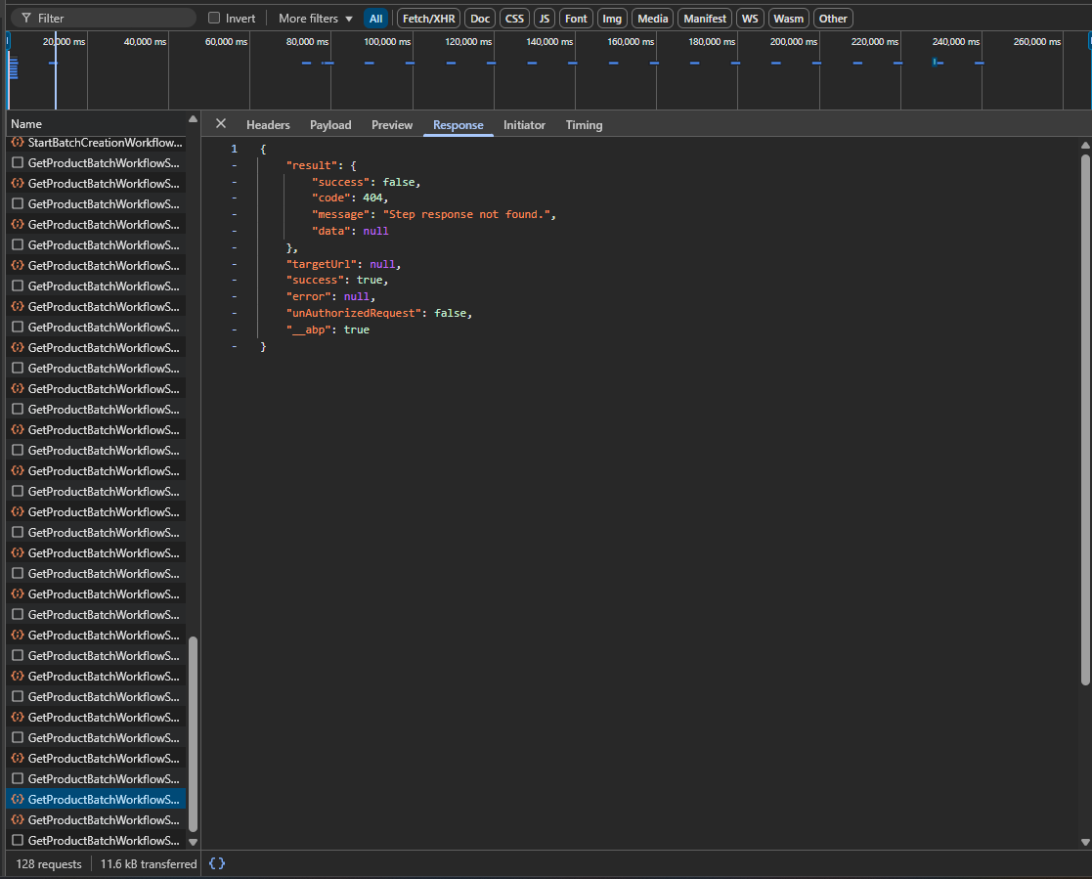
@peculiarnewbie any suggestion will be helpful. and thank you