✅ Random help
How do I get a random ulong out of Random.Next()? Random.NextInt64() seems to actually only give a random 63-bit number
43 Replies
Cast it...?
i meant how do i get out a fully random 64 bit number
i can obviously cast it
There are some overloads for the method, and one of them you can specify the inclusive min value, just specifies it as 0 and the max value as long.MaxValue
and then cast that to ulong?
No?
You'd need long.MinValue as the min
The 64th bit is the sign
ahhh okok
i think i can do that
i can cast that to ulong right
this would work right

Yeah, that should work. (I'd use
long.MinValue
and long.MaxValue
)I don't think so
Int64.MinValue is a negative value
you want a positive value, unsigned long
that's why i have the type cast there
maybe use Math.Abs to convert it to positive
...then what is that point
I think it'll faill, your cast
that would turn -1 into 1
which leaves me with the same 63 bits worth of numbers
well then what does Math.Abs do
NextInt64 return a non-negative long
https://learn.microsoft.com/en-us/dotnet/api/system.random.nextint64?view=net-9.0
Random.NextInt64 Method (System)
Returns a non-negative random integer.
Too slow
there is an override
*overload
this will take any int64, inclusive of negative ones
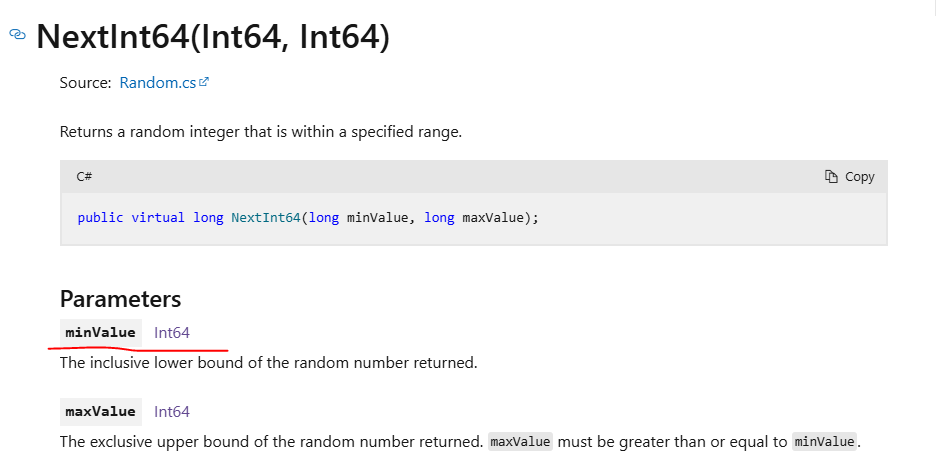
I'd possibly wrap the cast in
unchecked
ah yea
ooo ok
it seems to be working without that tho
see

ok that's settled
It will throw if you're in a
checked
context, either because someone wrapped your method in checked
, or if the assembly was compiled with checked arithmeticoh
so slap unchecked on it gotchu
ohh, now I see your point, you want to have a random value starting from 0 and ending at ulong.MaxValue, which is greater than long. The solution I said was to have only positive numbers, but not attending the whole range of ulong.
yes
Yeah, it's one of those things that normally doesn't matter, but is good practice just for the odd case where it does matter for some reason
For this case I'd say you should use RandomNumberGenerator instead of the Random class
... Why?
i need the number that comes out to be fixed
i seeded the random
(That also doesn't have a method for Int64's, FWIW)
it's ok the Random.NextInt64() works
just gotta tweak it a lil
nevermind then
I don't understand how
RandomNumberGenerator
makes it any easier to generate a random ulong ><I've never said it'd be easier 😛
I misunderstood his problem, I thought he wanted to generate a ulong value totally random, that's why I said to use the RandomNumberGenerator and not because it'd be easier, and that's also why I said "nevermind" because I realized I understood everything incorrectly when he said he had to seed the random numbers.
I don't know how you determined that he needed a cryptographically secure random number from that discussion 😛
use the
Span<byte>
overload, then reinterpret cast to ulong with Unsafe.As
@ElectricTortoiseit's ok i have an easier solution already
why not just
Unsafe.BitCast<long, ulong>
?Why use Unsafe when you can just use a normal cast?
fair
it's been established that it's positive, it's missing a bit of information
and the span overload is more flexible, if you know what you're doing
like if you're going to need 100 numbers, you can
stackalloc
a buffer for all of them and generate them all at once