Intercept Kinde Callback
Hello, I'm wondering what is the best pattern to intercept Kinde's callback URL and do some work before letting the redirect through?
A current use case is that I have email invite setup where if user A signs up to Org X, I need to double check if user A was invited to Org X after they've signed up on Kinde via a Register Link. I've tried to add an onSuccess functino within handleAuth but I don't think it's working because console.log doesn't even show up in npm run dev.
Would appreciate some suggestions, thank you!
5 Replies
Hi there, thanks for reaching out.
This is Patrick from Kinde.
I am not sure what kind of SDK you use.
There are a few approaches you can take to intercept the callback and perform work before the final redirect: 1. Use a custom redirect URL in your environment variables
(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) : KINDE_POST_LOGIN_REDIRECT_URL=http://localhost:3000/api/auth/success 2. Then create an API route to handle the success callback where you can perform your checks : // /api/auth/success/route.ts import { getKindeServerSession } from "@kinde-oss/kinde-auth-nextjs/server"; import { NextResponse } from "next/server"; export async function GET() { const { getUser, getOrganization } = getKindeServerSession(); const user = await getUser(); const { orgCode } = await getOrganization();
// Do your invitation checks here
return NextResponse.redirect("http://localhost:3000/"); } 3. Alternatively, you can store the intended URL in local storage or cookies before redirecting to Kinde
(https://docs.kinde.com/authenticate/custom-configurations/redirect-users/) : localStorage.setItem("nextUrl", "/some-protected-route"); Then after authentication: const nextUrl = localStorage.getItem("nextUrl"); window.location.replace(nextUrl); 4. You can also use the state parameter to store additional information . The state parameter is already used for CSRF protection but can store additional data: { "BlueFox0101" : { nextUrl: '/some-protected-route', } } For your specific use case of checking invites after registration, I would recommend approach #1 with a custom success redirect endpoint, as it gives you the most control over the flow and allows you to perform server-side checks before the final redirect. I offered solutions using the Next.js SDK. If you need further assistance, please let me know.
There are a few approaches you can take to intercept the callback and perform work before the final redirect: 1. Use a custom redirect URL in your environment variables
(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) : KINDE_POST_LOGIN_REDIRECT_URL=http://localhost:3000/api/auth/success 2. Then create an API route to handle the success callback where you can perform your checks : // /api/auth/success/route.ts import { getKindeServerSession } from "@kinde-oss/kinde-auth-nextjs/server"; import { NextResponse } from "next/server"; export async function GET() { const { getUser, getOrganization } = getKindeServerSession(); const user = await getUser(); const { orgCode } = await getOrganization();
// Do your invitation checks here
return NextResponse.redirect("http://localhost:3000/"); } 3. Alternatively, you can store the intended URL in local storage or cookies before redirecting to Kinde
(https://docs.kinde.com/authenticate/custom-configurations/redirect-users/) : localStorage.setItem("nextUrl", "/some-protected-route"); Then after authentication: const nextUrl = localStorage.getItem("nextUrl"); window.location.replace(nextUrl); 4. You can also use the state parameter to store additional information . The state parameter is already used for CSRF protection but can store additional data: { "BlueFox0101" : { nextUrl: '/some-protected-route', } } For your specific use case of checking invites after registration, I would recommend approach #1 with a custom success redirect endpoint, as it gives you the most control over the flow and allows you to perform server-side checks before the final redirect. I offered solutions using the Next.js SDK. If you need further assistance, please let me know.
Hey Patrick, thank you so much for responding so quickly! I'm using Next and relatively new to development, so if we setup a custom redirectURL, do we still need the src/app/api/auth/[kindeAuth]/route.ts ? I guess that's where I'm very confused.
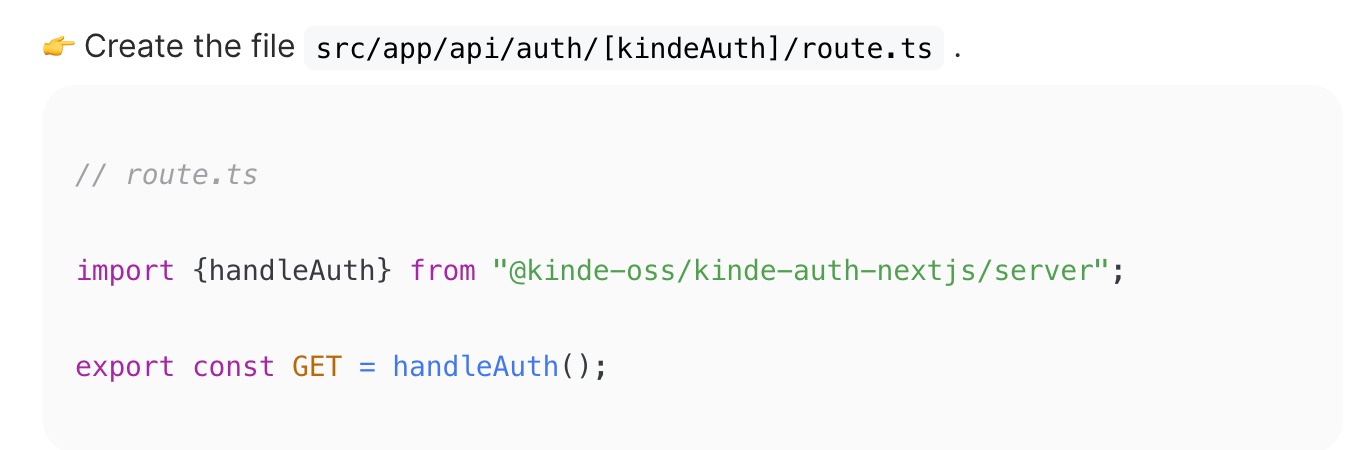
Yes, you still need the
(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) . It needs to be at
(https://docs.kinde.com/developer-tools/sdks/backend/nextjs-sdk/#authentication) : -
(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) . Before that can happen, the OAuth flow needs to complete via the
[kindeAuth]
route file. Here's why:
1. The [kindeAuth]
route is essential for handling the OAuth flow with Kinde(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) . It needs to be at
/app/api/auth/[kindeAuth]/route.ts
and should contain:
import { handleAuth } from "@kinde-oss/kinde-auth-nextjs/server";
export const GET = handleAuth();
2. This route handles several key Kinde Auth endpoints in your Next.js app(https://docs.kinde.com/developer-tools/sdks/backend/nextjs-sdk/#authentication) : -
/api/auth/login
- /api/auth/logout
- /api/auth/register
- /api/auth/kinde_callback
3. The custom redirect URL you set in KINDE_POST_LOGIN_REDIRECT_URL
only determines where users go after the authentication is complete(https://kinde.com/blog/engineering/nextjs-with-drizzle-and-kinde-auth) . Before that can happen, the OAuth flow needs to complete via the
kinde_callback
endpoint that's handled by the [kindeAuth]
route.
So in summary:
- Keep the [kindeAuth]
route for handling the OAuth flow
- Use custom redirect URLs for what happens after successful authentication
- Both pieces work together - the OAuth handling needs to complete before your custom redirect can occuroooooooooo I see, yea that is where I'm getting stuck. I didn't know that so this is exactly what I want it to do. Essenetially the Login URI is a middle route after OAuth where you can do processing right. Before you render your page/app
I'm doing crazy stuff like:
export const GET = handleAuth({
async onSuccess(request: NextRequest, response: NextResponse) {
console.log('AUTH CALLBACK TRIGGERED!!!!');
try {
const url = new URL(request.url);
const pathname = url.pathname;
if (pathname.endsWith('/kinde_callback')) {
console.log('Intercept Kinde Callback:', request.url);
const kinde = getKindeServerSession();
const { getUser } = kinde;
const kindeUser = await getUser();
-----------
No wonder this does not work LOL
Sounds great.
I'm happy to help you.