How to build for React + Vite + Cloudflare worker?
I am using this example provided by Cloudflare for Vite + React + Hono https://github.com/cloudflare/templates/tree/main/vite-react-template which works fine if I use it as-is.
But I need to change the static
index.html
to index.tsx
to serve the initial html by hono to add title, description, meta tags etc. based on the pathname.
So, I changed the index.html
with index.tsx
And, I also tried to change the vite config to add build from import build from "@hono/vite-build/cloudflare-workers"
but it doesn't work.
GitHub
templates/vite-react-template at main · cloudflare/templates
Templates for Cloudflare Workers. Contribute to cloudflare/templates development by creating an account on GitHub.
13 Replies
what doesn't work?
It doesn't build properly, I see blank screen on browser with this error on console
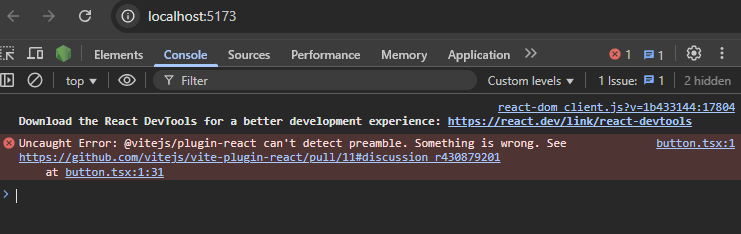
Here is the full code - https://github.com/vickyRathee/vite-react-template
GitHub
GitHub - vickyRathee/vite-react-template: Vite react template using...
Vite react template using Hono and styling with shadcn to deploy on Cloudflare workers - vickyRathee/vite-react-template
idk if this is the problem, but i don't think this should be a string: https://github.com/vickyRathee/vite-react-template/blob/cd322371ede3055f9695ace5d2da6f08def63548/_index.tsx#L15
this is working fine as string, I can see the HTML in response. So this line is not an issue!
I answered in #cloudflare, didn't see this post. You're getting that error @Vikash as mentioned in my first reply. The Vite React plugin expects an HTML file - which isn't here, because you're rendering the HTML now upon request using Hono. You can't have both
I was checking your example and it has lots of imports from
hono/jsx
, I need to use my application as is because it's a migration from Next JS and lots of shadcn components. So, anyway to just serve the index.html
as it's a SPA, so everything else should work as is in given Cloudflare exampleCorrect, I didn't dive into React specifically - though the same concept applies here as the API is more or less the same. If you're stuck with the static
index.html
file, I'd suggest looking into https://vite.dev/guide/api-plugin.html#transformindexhtmlI don't think the transformhtml will work, because I need the hono context to get the meta data from KV store to append as per pathname. For example, I have 1000 products page, each product metadata will be stored in D1/KV on cloudflare.
So the only solution is initial html render should be done by hono
In that case, get rid of the React Vite plugin & make use of the React rendererer middleware. If you need to ship JS to those pages, the article I shared should help you out
That's not importing my main script
<script type="module" src="/src/react-app/main.tsx"></script>
can you try running it from my github repo when you have some time?I don't see you use the React renderer in your repo. Here's a snippet of an app I started building yesterday:
This is resolved by using the new react router example - https://github.com/yusukebe/hono-react-router-adapter/tree/main/examples/cloudflare-workers
GitHub
hono-react-router-adapter/examples/cloudflare-workers at main · yu...
Hono <-> React Router Adapter. Contribute to yusukebe/hono-react-router-adapter development by creating an account on GitHub.