Admin Users Count
Hi. I am currently trying to get just the count of admin users. My query looks like the following:
but the response looks like the following although the docs state that the "total" in the meta data is the count after the filters:
Any insight into this would be awesome.
6 Replies
Here is a snip of the admin dashboard I am creating
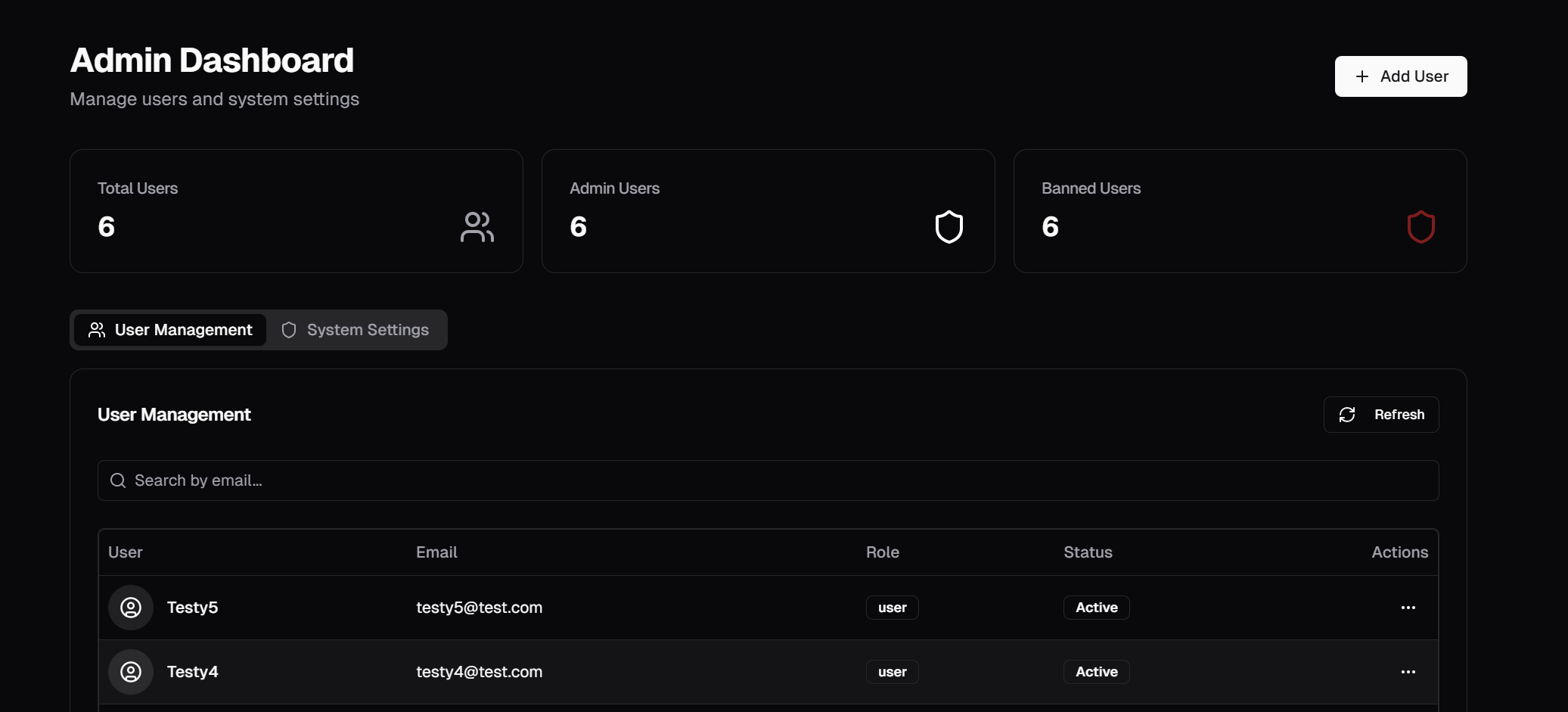
What are you expecting as the output? You are querying users with the role 'admin', as per your response you have 6 users matching this filter? You are only seeing 1 user because you set the limit to 1. Limit != Filter.
Whats up @Lick A Brick. I'm expecting the total to be equal to the number of users with the given filter criteria. I only have 1 admin user so I would expect "total":6 Right now its returning the total number of all users
Hmm... the docs seem kinda confusing to me tbh. At https://www.better-auth.com/docs/plugins/admin#list-users it mentions the parameter
filter
which accepts an array of objects, but in the example it shows filterField, filterOperator and filterValue as seperate parameters. Should't it be filter: [{ field: "role", operator: "eq", value: "admin"}]
what happens if you remove the limit: 1
does it return all 6 users or does it still only return the single admin user?Sorry. Just realized I didn’t respond. I did try this but got a type error. I looked into it further and filter isn’t a query option. I’m going to update and see if that changes anything
Can you share which adapter you are using and its configuration?