Why wont it reach return?
does anyone know why it wont return after it says "Please enter a valid discord webhook"? It looks as if everything it right to me. I deleted the thread.sleep but still nothing happens. Why wont it reach return;?
31 Replies
It reaches "Please enter a valid discord webhook" but not "return" which is right after
how are you checking this? in a debugger and stepping through line by line?
well im just using the console since this is a console app, is that a bad thing? not that experienced with coding so i dont really know how to debug professionaly and so
async void and Thread.Sleep is a really weird combination
why is it async at all? you're not awaiting anything
in async contexts you should
await Task.Delay(time);
im fairly new so just experimenting my ways forward atm. I changed async void to async task and the main to an async aswell so i can await the programs or functions, im not sure what they're called in c# sorry
yea i just changed that but thanks
i would just avoid async for now, it adds overhead and programming considerations that aren't applicable to simple console apps
so async isnt needed for sending http requests to discord?
no
i see, i was tricked by someone on the internet then i suppose
you can call
Post
instead of PostAsync
if you do want to keep using async, there are some important points
* don't use async void
unless you absolutely can't help it (basically only for event handlers)
* never call Wait()
.Result
etc., you should await
the task instead
and don't use things like Thread.Sleep because that will not work how you think it will in async methods
basically, async exists to avoid blocking threads while doing IO work like sending messages over the network, reading/writing from disk, etc.
but if that doesn't matter for your program (which it probably doesn't for a beginner console app) it just adds complexity and performance overhead to your programthanks a lot for the help, i will definitely save this to my notes 🙂
there is $nothread for a more in depth explanation of what async is doing
There Is No Thread
This is an essential truth of async in its purest form: There is no thread.
thank you
however, even though ive change everything needed to task and await it still wont return when i want it to, it only goes back when put in a random key, which i dont want it to do
i dont think theres anything wrong with the code now
that i sent
what does the code look like now?
but im triggering the code ofcourse through switch and cases
this is the code i sent earlier
while this is the function that actually triggers it
dont mind the 2 breaks, i added one just for debugging
but that didnt seem to be the problem
by random key you mean it doesn't keep running until you press a key?
Console.ReadKey()
stops your program until a key is pressedyea so basically, when i put a random number into the webhook, it says this
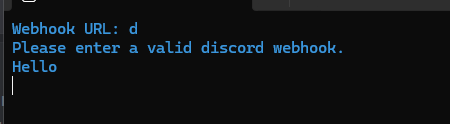
oh, that might actually be it
since it returns once i press a key
so to be more specific here, it is returning no matter what
but the line of code after that makes the program wait for a key
okay i see
also as an aside the
doit
variable is unnecessary because the code that checks it already won't run if any of those early returns are hiti know how to fix it, but i kinda come from roblox development and i am certain that you guys dont do the same things we do there. To check if the discord webhook is valid or not do i create a boolean thats set to false if not or true if yes?
booleans and how you use them are up to your program, they're just data types that can hold true or false
i was just thinking if there were a more efficient or cleaner way of doing it, like checking result or something
to check if a webhook is valid it depends exactly what you mean, is it valid if the URL matches a specific pattern or only if you can actually send a successful request to it?
this code checks if its valid or not, and then if it isnt it sets doit to false then waits a sec before returning.
i just check it if its blank or if it doesnt contain the webside url
right, so
doit
has no use here because every time you set it to false you're returning anyway
once you get to a return
any code below that won't run
so your if (doit == true)
will always evaluate to true if the code gets to that pointso you think i should ditch the if statement?
i would, it's not doing anything useful here
(also, you could just do
if (doit)
)alright
i fixed it
might not look wonderful
but it works
thanks for the help i really appreciate it