AI Worker form Workflow throws Network connection lost Name: AiError
Simple calls to other models work, but I am trying to process an uploaded video from R2 and hit this error.
Code for workflow
import {
WorkflowEntrypoint,
type WorkflowEvent,
type WorkflowStep,
} from "cloudflare:workers";
import { NonRetryableError } from "cloudflare:workflows";
import type { FlipperBinding } from "@repo/types";
export type WorkflowVideoParams = {
key: string;
};
export class WorkflowVideo extends WorkflowEntrypoint<
FlipperBinding,
WorkflowVideoParams
> {
async run(event: WorkflowEvent<WorkflowVideoParams>, step: WorkflowStep) {
// Can access bindings on `this.env`
// Can access params on `event.payload`
let video: R2ObjectBody | null;
await step.do("getting video from R2", async () => {
console.log("getting video from R2");
video = await this.env.COVE_STORAGE.get(event.payload.key);
if (!video) {
throw new Error("Video not found in storage.");
}
});
await step.do("ai video", async () => {
if (!video) {
throw new Error(
"Not processing video, as it was not found in storage.",
);
}
try {
console.log(`processing ${video.key} video with ai...`);
const blob = await video.arrayBuffer();
const input = {
audio: [...new Uint8Array(blob)],
};
const response = await this.env.AI.run(
"@cf/openai/whisper",
input,
);
console.log(response);
} catch (e) {
console.error(e);
throw new NonRetryableError("Failed to process video with ai.");
}
});
}
}
import {
WorkflowEntrypoint,
type WorkflowEvent,
type WorkflowStep,
} from "cloudflare:workers";
import { NonRetryableError } from "cloudflare:workflows";
import type { FlipperBinding } from "@repo/types";
export type WorkflowVideoParams = {
key: string;
};
export class WorkflowVideo extends WorkflowEntrypoint<
FlipperBinding,
WorkflowVideoParams
> {
async run(event: WorkflowEvent<WorkflowVideoParams>, step: WorkflowStep) {
// Can access bindings on `this.env`
// Can access params on `event.payload`
let video: R2ObjectBody | null;
await step.do("getting video from R2", async () => {
console.log("getting video from R2");
video = await this.env.COVE_STORAGE.get(event.payload.key);
if (!video) {
throw new Error("Video not found in storage.");
}
});
await step.do("ai video", async () => {
if (!video) {
throw new Error(
"Not processing video, as it was not found in storage.",
);
}
try {
console.log(`processing ${video.key} video with ai...`);
const blob = await video.arrayBuffer();
const input = {
audio: [...new Uint8Array(blob)],
};
const response = await this.env.AI.run(
"@cf/openai/whisper",
input,
);
console.log(response);
} catch (e) {
console.error(e);
throw new NonRetryableError("Failed to process video with ai.");
}
});
}
}
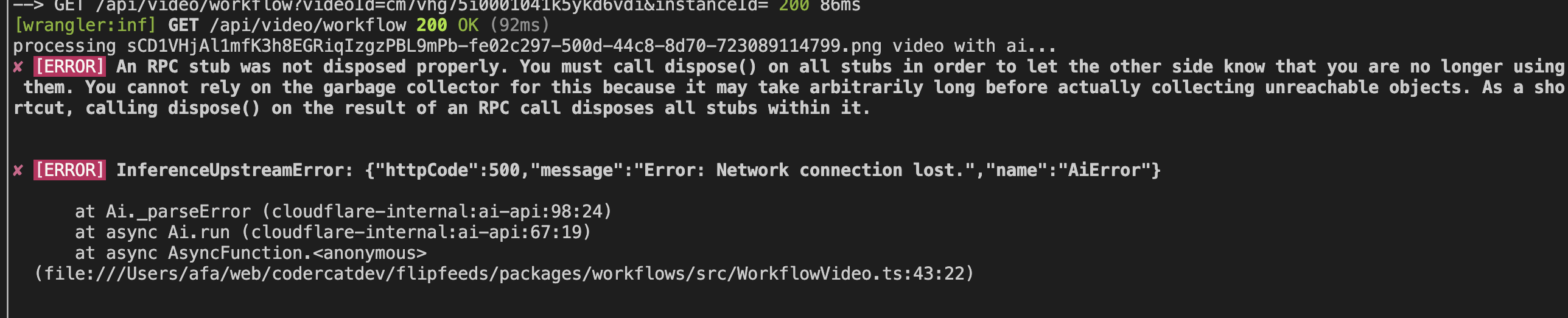
0 Replies