(Beginner) Instantiate objects of a class at runtime
I am learning C# by following tutorials and practicing code in Visual Studio. I will be moving on to Unity at some point, but I want a firmer understanding of C# before I do that.
I can create classes and create objects of those classes in the code. eg:
class Enemy //define an 'enemy' class
{
int xLocation;
int yLocation;
string name;
}
Enemy enemy1 = new Enemy(); // create an enemy object (in Main of course)
This creates an object that I can use in various ways. (yes, I understand constructors and initialising the fields too, I'm just keeping it short)
But what if I want to create Enemy objects at runtime. ie lets say I get an input from the user and want to 'spawn' some enemies. In my mind this means I am instantiating objects at runtime rather than in the code.
Is my understanding wrong? All my searches point me to Unity tutorials which makes me think I am misunderstanding something, I don't want to use Unity just yet. Any pointers in the right direction greatly appreciated.
10 Replies
As a bit of context on what I'm doing, I have created a 2d array that works as my gameworld. I have created a player object (the X in the middle). I can move him around with numpad.
I only needed 1 player object because there's only 1 player, so I did it in code. But in my thinking I am going to need to create some objects at runtime, not in advance. Enemies will be one example, but lots of other things too probably.
Point out the error in my thinking please 😄 😄
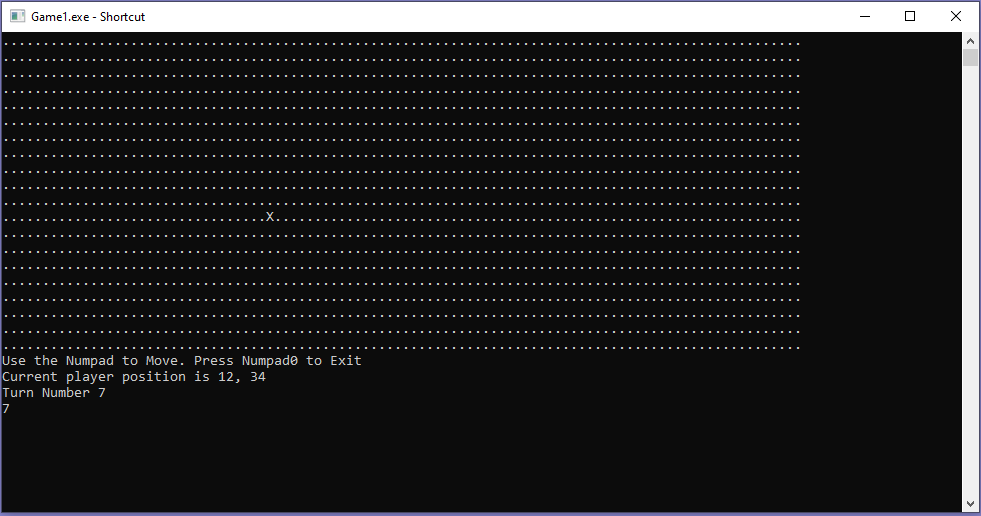
You probably want to use
Activator.CreateInstance
.
You do need to pass in the type to instantiate, and note that this activator utility attempts to find the msot suitable constructor using the parameters you passed.
However, if you do not care about arguments and you have no constructor like in your example, then the better solution might be to use generics. You can put a constraint on them which indicates you can create a new instance using no constructor or a parameterless constructorSomething like this:
https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/keywords/new-constraint
new constraint - C# reference
new constraint - C# Reference
That said, generics is a whole topic by itself
Brilliant, thank you. I'm going to do both because this is about learning rather than making a good program 🙂
I imagine I will care about arguments because when I initialise a new enemy I will want to randomise it's starting location for example.
This is the alternative I am talking about:
Note this does use reflection and does not have any direct relation to the types, in which case it might compile but throw at runtime
There is a third option which is to use dependency injection. You can make factories and/or use
ActivatorUtilities.CreateInstance
(which is not Activator.CreateInstance
) and you generally do have more control over the behaviour. This probably does mean you end up rewriting your project quite a bit, but if you do that you do end up with a very solid structure.
If you use dependency injection you could make an EnemyProvider for example, and then this implements the behaviour to create the enemy. Rather than having a generic Instantiate method for everything you have more fine grained control over how to create the enemy, and the EnemyProvider by itself would have the logic it needs to instantiate it properly into the scene, since the Dependency Injection would have provided those too through other providers and such.look up factories
it's basically the last message that the person above sent
you generally want factories over reflection
This is plenty for me to go on, which is great, thank you. I can already tell I'll be able to figure this out now that I have somewhere to start. Thank you very much!
reflection is fine as one of the fallbacks
BTW I mentioned factories combined with DI here but you can also do it without DI
It's just that generally they go hand in hand