Rendering too resource-intensive
I am using Swing to render a graph in a window; however rendering is taking too long and is too resource-intensive. What changes can I make to help with performance? Here is the class responsible for rendering my graph:

10 Replies
⌛
This post has been reserved for your question.
Hey @Danish Pirate! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
updateGraphView()
is called whenever the nodes in my graph are connected upon initalization and whenever a node is visited.How exactly do you see it taking too long?
I need to wait a couple of seconds for anything to be rendered at first. Then my window freezes
I optimized it a bit by caching
minLat, maxLat, minLon, maxLon
instead of calculating them every view update.so the rendercomponent takes too much time?
I didn't know you'd need to iterate over all nodes there
I need to redraw all the edges between the nodes every view update.
can you check what actually takes too long?
Seems to be the iterating over the nodes
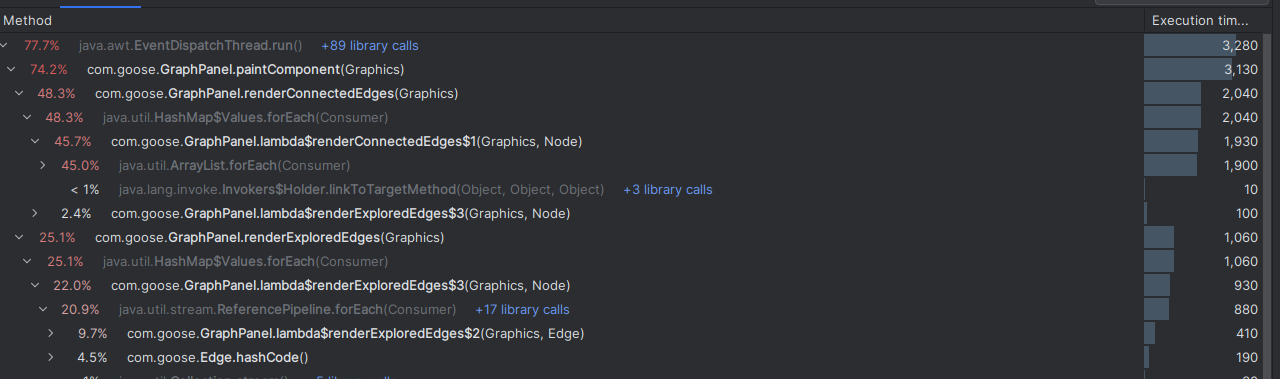
So you are iterating over all nodes/edges and computing min/max for lat/lon fir each of them
Why?
just compute these once per rendering
Post Closed
This post has been closed by <@173447330719072258>.