✅ A dictionary that I made isn't working, but only in one method. It works fine elsewhere.
Image 1 is the code for adding the objects to the dictionary
public static Dictionary<int, ItemData> Items = new Dictionary<int, ItemData>();
is how I have the dictionary set up and that works just fine.
In image 2 when I try to run a task that checks the dictionary with the key 1 it should return the object ItemData
with the values 1,"Oak Wood","A simple material for crafting.",0,0
but it returns null and the value for the dictionary is Count = 0
Image 3 is using the same .TryGetValue
key in a different method and it's working how it should and returning the right data.
I have tried hard coding the ItemData
values in the dictionary directly and that didn't change anything so it has to be a problem with the asnyc task is my best guess but google is giving me nothing and I have genuinely no clue where to start doing anything else to fix this.
That's the code for object. This problem only arose when I removed a bool value and added the int ArmourType
.
Image 4 is the code for the async task causing the problem and the line used to run the task is Task.Run(() => Data.Skill(4, 10000, 1, 1, 3, 10, 15)).Wait();
If anything else is needed to help I can provide it.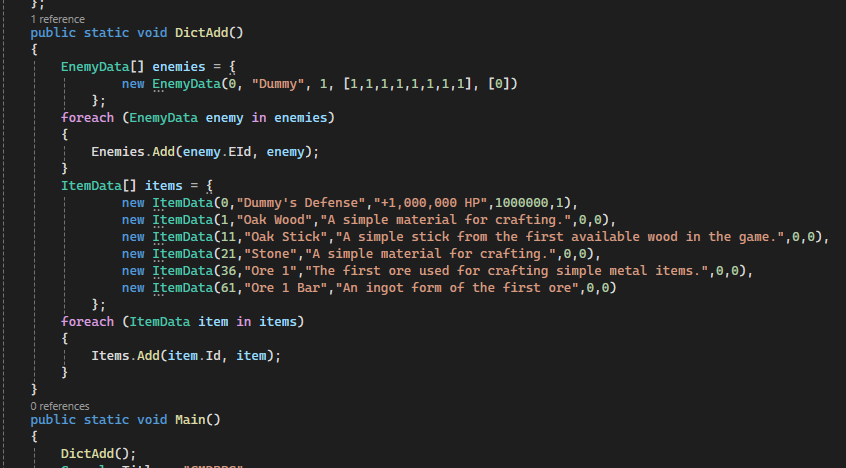
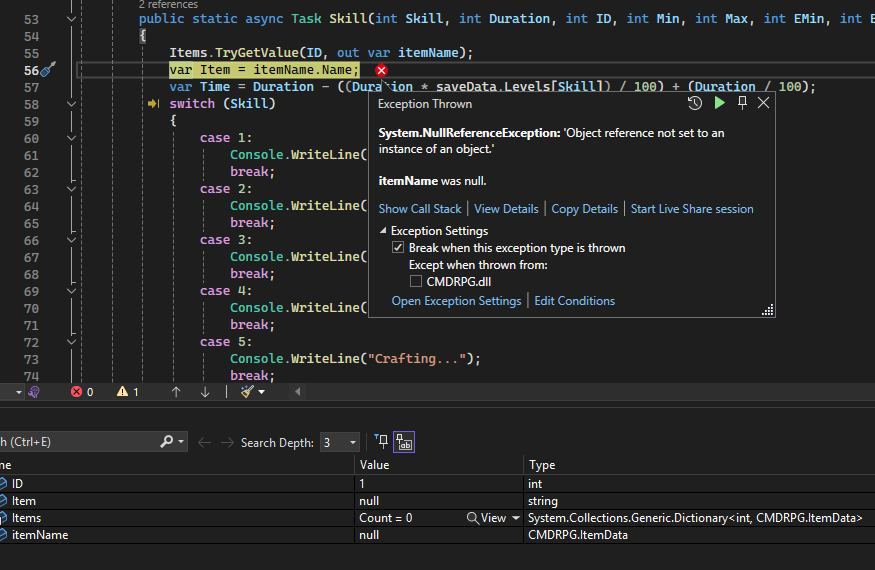
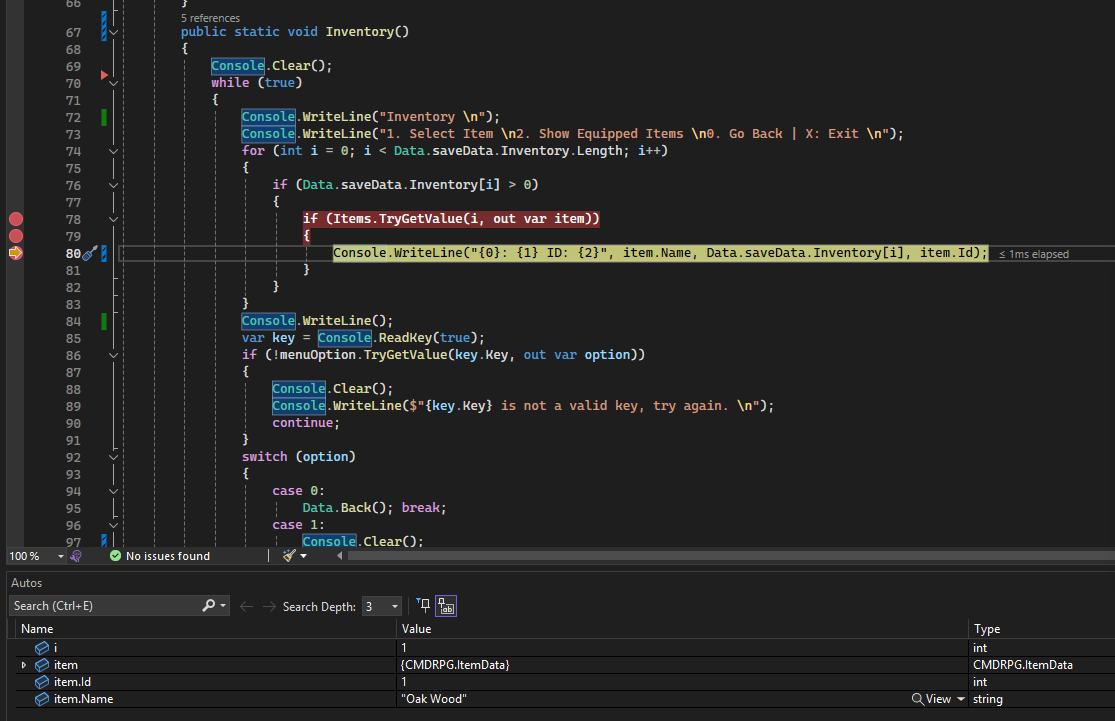
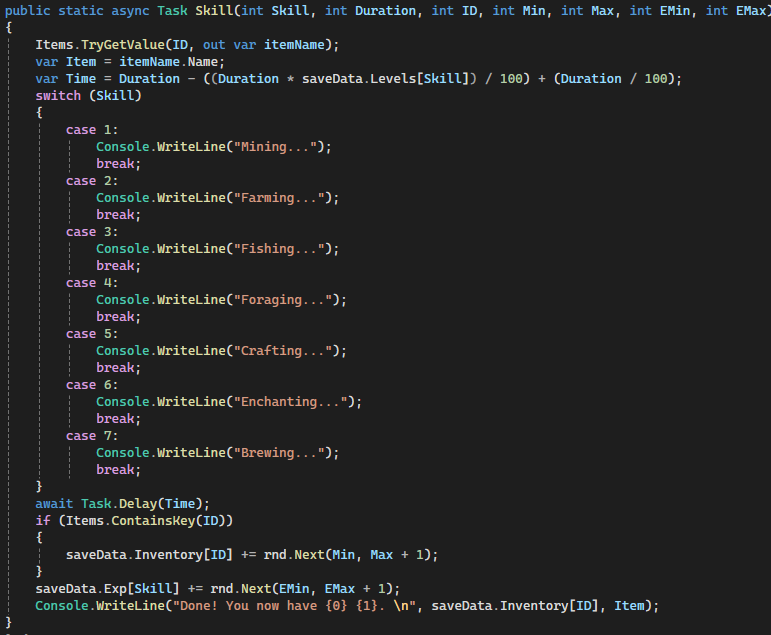
101 Replies
Dictionaries arent threadsafe
if you want thread safety use ConcurrentDictionary
also dont
.Wait
tasks
the whole point of tasks is to not do that
why are you using a task?I'm just confused because it used to be (int, string, string, int, bool) and worked fine but when I changed the object to (int, string, string, int, int) is when it stopped working.
I didn't know how else to do what I needed to do.
what im trying to ask is what are you trying to do
Oh, it's a video game in the command line and the only way I could figure out how to save the information about the menu you're in while doing Task (in game, not the coding sense) was to make it a seperate thread.
have you learned classes yet?
Yes
I think
I have more than just that 1 at the very least
oh yeah i just saw the class you have declared lol
whats up with me today
anyway
Lol, all good
that
(int, string, string, int, bool)
thing should probably be class
let me check out your codei just switched to my pc
actually first of all
$pasteIf your code is too long, you can post to https://paste.mod.gg/, save, and copy the link into chat for others to see your shared code!
Yeah that's what I meant. The class for the
ItemData
object used to be (int, string, string, int, bool)
but I changed it to (int, string, string, int, int)
and then the task broke.so what you have now is a dictionary of
ItemData
?
and when you change ItemData
your code breaks?The only thing I haven't tried is changing the
ItemData
class back to (int, string, string, int, bool)
can you show the code where you add your item to the dict?
just to be clear, do you mean by that an actual tuple, or that the
ItemData
class has those fieldsyou could initialize the dict wit those
The item class has those fields
also your main isnt async
And it does, it just doesn't work in the task
The main doesn't call the task
if you have async code your main should be async
I didn't know that
Main does fairly little in my program tho
rule of thumb is await amything async, async all the way up
you can just do that for now if all you need is delays
until you learn async properly
or you can just use Thread.Sleep
anyway
where is it called then
the thing that takes the item from the dict
A different method called
Tutorial()
That's in a different classis your code on github by any chance
Yes
GitHub
GitHub - SammyOwO/CMDRPG: A simple RPG game I'm making in the comma...
A simple RPG game I'm making in the command line. Windows only hence CMDRPG and not UnixRPG lol - SammyOwO/CMDRPG
That's already the latest version
I pushed when I posted this
It's a lot worse than you could ever expect
so in general we prefer that the
Program
class and Main
to only do startup
and have the game handled in its own class
rather than have all that in Program.cs
Main only does startup
also functions should only do one thing
generally
if you have a function thats longer than 20 lines its probably too long
lines arent a good mesasure of this, just a rule of thumb
Program.cs has the stuff that I need the whole program to access like that async task
what is a good measure is asking yourself "is this doing only one thing?"
I have a method that calls a task and if you follow the line of nested loops and if else and switch case statements it's 21 long
if something requires something else, pass it around
Yeah, but that's why it's a dictionary
So it can be accessed where it's needed
just to give you an idea, main should probably just be
I mean I guess I can make main 4 lines of code in my case
dictionaries are for "give me a value for this key"
access is a whole other thing
Well I mean that's all I need
anyway ive gone off on a tangent here
All good
where are you using that dict
For the Inventory menu and for that asnyc task
Menu.Inventory()
and Data.Skill()
Data.cs also holds the classes for the objects
As well as the outline for the save file.
SaveFile
EnemyData
and ItemData
another rule of thumb is one file per class
right so
i think you should refactor your code first before continuing
I feel like it'd be dumb to put those 3 in a seperate file tho bc it's only used by the
Data
class in Data.csbecause debugging this is very hard
and the more you go on the harder it will be in the future
my guess is its a thread safety issue, but i cant follow your code to make sure
I mean I would refactor it if I knew any other way to write the code. I wrote everything relating to the inventory method yesterday
start by breaking up methods
one method that does one thing
let me show you an example
what does
menuOption.TryGetValue
do?
get a value from the save data?That just makes it so pressing D1 or NumPad1 return the int 1
or D2 and NumPad2 returns 2 etc
That's literally just to make menu options work properly whether you use the num pad or the number row
It also includes X and I
X to quit and I for inventory
right so that for example should be handled by an
Input
class or something like that
because its not related to menusHonestly
It works
I'm just gonna leave it
but its messy
and it makes debugging hard
this is a good opportunity to learn refactoring
you have a bug thats hard to diagnose
I mean I know what refactoring means and why it's important
thats your motivation
But I genuinely can't write my code any other way
im explaining to you right now
^
Yeah, but I wouldn't know how to do that
I know how to do that with dictionaries
And it would take a solid 10 hours to change
what i meant is move that code there
thats how you learn
another example,
this shouldnt just be a random string, there should be a list of places that you display
this would be handled by your
Input
class
e.g. Input.GetKey()
What
and the
Places
function should be named something like GoToPlace
and passed the placeThat's the way it every menu is
I didn't even write that code
I just copied and pasted it bc it worked
where did you get it from?
yeah no dont do that
you can copy paste code that works only if you fully understand what it does and why it works
I mean it's before literally every single menu option in this program
and if youre new you shouldnt even do that, you should write it yourself
yeah and im telling you how you should refactor that
Yeah, I understand
Basically anything outside of the tutorial function does nothing right now except the inventory
an example of a method that does more than 1 thing,
Menu.Places()
. it does
1. display places that the user can go to
2. read and process input
3. go to said place
these should be 3 different methodsI don't know how I could break it up
start by refactoring everything that has to do with your bug
put those 3 things into their own methods
It's just the task method
And I can't find anything wrong
Nothing about it changed
I only changed the
ItemData
class to have an int at the end rather than a boolwhat i meant is, everything from
Main
to wherever your bug isYeah I couldn't find any issues with any of it
It just broke
thats most probably just a coincidince
i know, im recommending you refactor that
so that you could find the issue
I tried hard coding the values directly into the dictionary and teh same thing happened in the task
alright
those are your options
So it's not a problem with putting the values in the dictionary
1. refactor your code, make it easier to debug and more readable, then fix your issue
2. try to figure out whats going on using the debugger
3. try guessing what the issue is and changing random things until it works
I know where the problem starts. I just have 0 clue why it happens
like
^
I used the debugger to the best of my ability and I tried everything I could think of that related to the code
so that leaves you with option 1
The problem there is
The only thing that needs ot be refactored is the async task
And I don't even know how to re write that
no, far from that
like i said, break up methods into things that only do one thing
Everything else works though
youre asking the same questions in a cycle
it works until it doesnt
which is now
if you want to make it easier, or even possible, to debug
The only things that don't work are stuff I didn't write
then you need to refactor
besides that menu thing
That works rlly well
But I didn't write the async task
rewrite them yourself then :)
I have no clue where to even start
I would just rewrite the exact same thing
do that then
because youll understand it this time
delete the code and write it yourself
I mean I understand it
But that's because I used it
I don't understand why it broke though
listen thats all the advice i can give you
i already told you why its important to write the code yoruself
Oh my god
I found the problem
ah so you went with option 4
It was from when I refactored my code
look at the code until you randomly find the bug
I had 2 seperate
Items
dictionaries
And one just isn't being used at all
How do I mark solved
?i hope you take my advice and refactor
$close
If you have no further questions, please use /close to mark the forum thread as answered
I would love to, but I can't.
sure you can