useEffect() being called before reference is assigned
im making a react roblox ts component that should tween its size when the component is called and created but the useEffect() function is creating the tween before the useRef() is assigned to the frame
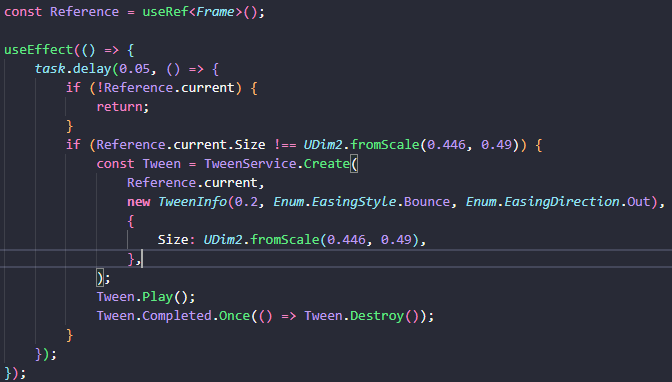
25 Replies
i tried seeing how long it would take before react sets the reference thats the only reason why there is a task.delay
dont mind that
wait
this might be a completely different issue than that
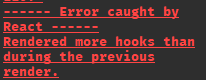
the issue is when i create the ref for some reason
i incrementally redid everything and found out this is where the problem lies for whatever reason
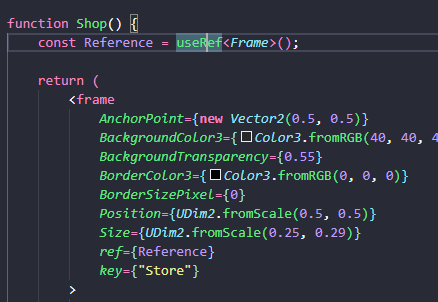
can you use something like ripple for tweening?
instead of manually writing them?
why?
that seems unrelated to the issue
it has hooks like useTween
where you can tell how to tween
and it seems like you have the issue like
can you check in your code where you do something like that
it should be something like
+ your useEffect might be missing the dependencies []
if you dont know where the error is can you try removing parts of the component to see if the part you deleted have been causing an error
i think it's better to start by removing this useEffect with animation just to see
It’s because the component I was rendering was a child of a parent
And it’s code was like
+ you might be missing a cleanup in the useEffect
So like
evil
what about it?
Children are different each render frame
And something about that react doesn’t like when using hooks instantly when rendered
that's not a problem
i think
what is Menus?
That’s what I changed and it fixed it
Map<string, Component>()
🫠
What 😭
can you make a switch component?
I changed it dw
I just rendered all of the components
oh
yeah, then prob switch will not work
Then set visibility and size/tween
btw
usage
and prob could be modified to something like
that means which menus to render you specify in ui
and there will be the set that you control "What menu to render"
could be useful i think 🤔
Sounds cool for times where there’s a much higher instance count but there’s a max of 3 menus so it should actually be okay
Definitely gonna note that for the future though thanks!
it's just a bad practice to use tweens
they suck
also https://discord.com/channels/476080952636997633/476080952636997635/1340373389453426711
Is that not un-conventional
Maybe I’m wrong
It seems like “ghetto” code
there are no conventions