Output Characters
Currently confused as to why there are actual "character" blocks being printed out with my String input result.
Please see screenshot for terminal log!
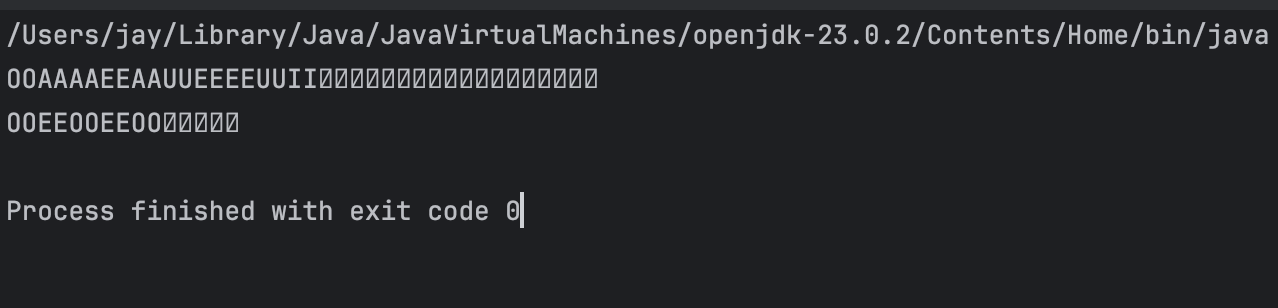
31 Replies
⌛
This post has been reserved for your question.
Hey @Jordan σ_σ! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
the char array is bigger than the data you write to it, and thus the string you return contains characters that haven't been filled (\u0000)
cmd displays these as the "missing character" symbol you see there
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
hmm i see
i wonder if i need to change phrase.length() that is passed into the char array to a specified length
i guess if you wanna do the exact char size as the desired string length
you could count the amount of vowels in the string
and then do
but thats an extra loop
yeahhhh
we wouldnt wanna do an extra loop here in this case
but then you dont know the array size you need to declare
im missing something...a key factor here about how the array size is not catching on to the size of the string thats passed through within the char array.
and this is why folks debugging is key XD
wait
cant you just use
why swap two values
i mean use two values
i mean you're right i could do that instead
because the first one just outputs the entire length
with those missing characters
hmm lemme try that actually
yea check
maybe its gonna work properly
also do you need to use an array
instead of stringbuilder
@0x150 do you know any way you can count the amount of vowels without an extra loop
so the array size is properly initialized
Can we change the size of it within the loop?
is that possible?
i dont think so
you would need to use stringbuilder
its faster than string
Indeed this is correct
and it has been resolved
cant believe i was overlooking StringBuilder...i didnt have it as my first choice right but I definitely was thinking about it but kept overlooking it and kept thinking that it wouldnt work
but after understanding that i could just pass in phrase.length() inside the StringBuilder constructor...because i didnt know it could take in an actual memory size...your conclusion worked out just fine
and i appreciate that
check it out
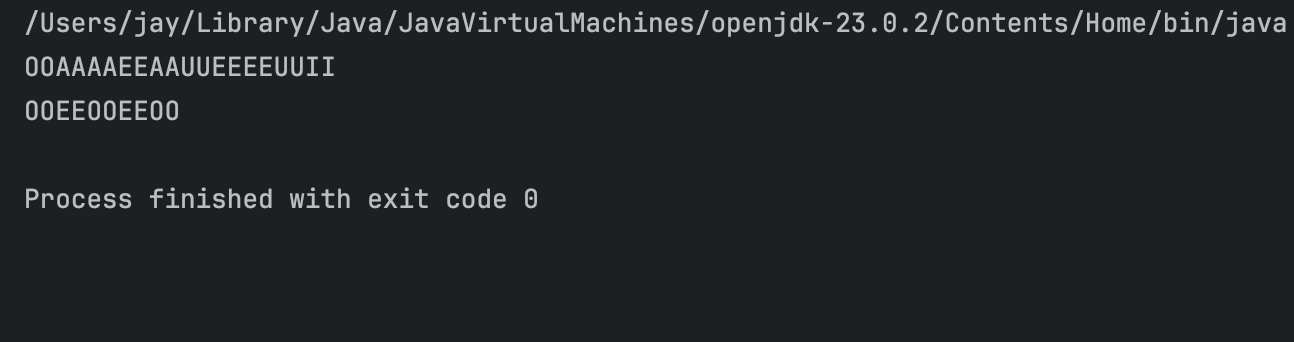
cool shit
truth be told
you could also use a list
for the
vowels
and instead of looping over all the vowels
just use
thats 1 loop less
by improving the speed of it we would use a List ye?
the more loops we can remove the faster the code would be correct?
yea the time complexity is lowered
Different topic but how do we calculate time complexity in our code? Is there a way we could do this locally to test our code to see how long each method would take?
if u wanna measure the time a method takes to finish u can do something like now
and about time complexity
GeeksforGeeks
Understanding Time Complexity with Simple Examples - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
Baeldung
Time Complexity of Java Collections | Baeldung
Learn about the time complexity for common operations on Java collections
cuz idk how to explain it simply
I appreciate this much
one more thing
.contains also runs a loop
on the vowels if it was a list
but
it stops once it finds that element
and if it doesnt it runs till the end
so in your example
to have the same functionality with a loop
it would be
not 100 percent sure about this though
additionally
to have the searching even faster in the vowels
you could use a set
because the time complexity to find it will be o(1)
instead of o(n)
so faster
but you can read about it more
i appreciate this much
if i could give a 5 star rating for customer service/assistance i would give it to you @0x150
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Post Closed
This post has been closed by <@649487890858115072>.