could you explain what this code is doing in terms of implementing the problem in the picture?
like what is the logic is

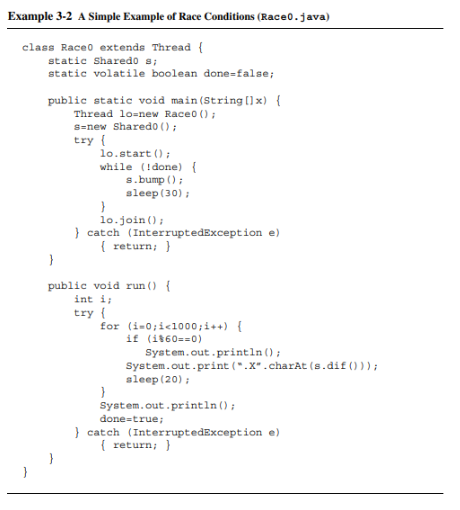
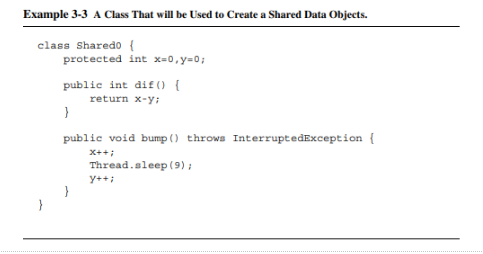
5 Replies
⌛
This post has been reserved for your question.
Hey @SidKid! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
^thats all one code, discord was saying it is too long to be in one block
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
there is no read difference between brokenIncrement and betterIncrement - both aren't thread safe
and well the code is trying to increment numbers a given amount of time but due to it not being thread safe, counter1 and counter3 might be too low
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.