Combine multiple images into a grid format with Java
I am trying to make a 2 x 4 grid view and i need to start arranging images from left to right. i have the paths inside a List<String>
16 Replies
⌛
This post has been reserved for your question.
Hey @alex! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
So what exactly is the problem there and how does it relate to your
List
containing paths (whatever you mean with that)?So I tried searching a similar usecase and found this
the issue is that the max capacity is 3 x 6 rn, if i have lesser images for example 5 i still want 3 x 6 display and the unfilled spots should remain vacant
so?
if the
images
array is null
at these positions, just add a null check
if the size of that array is smaller, add a bounds checkohh ok thanks let me try that in my local and see how it goes, i will update here once i have some follow up
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
@dan1st thank you very much it worked
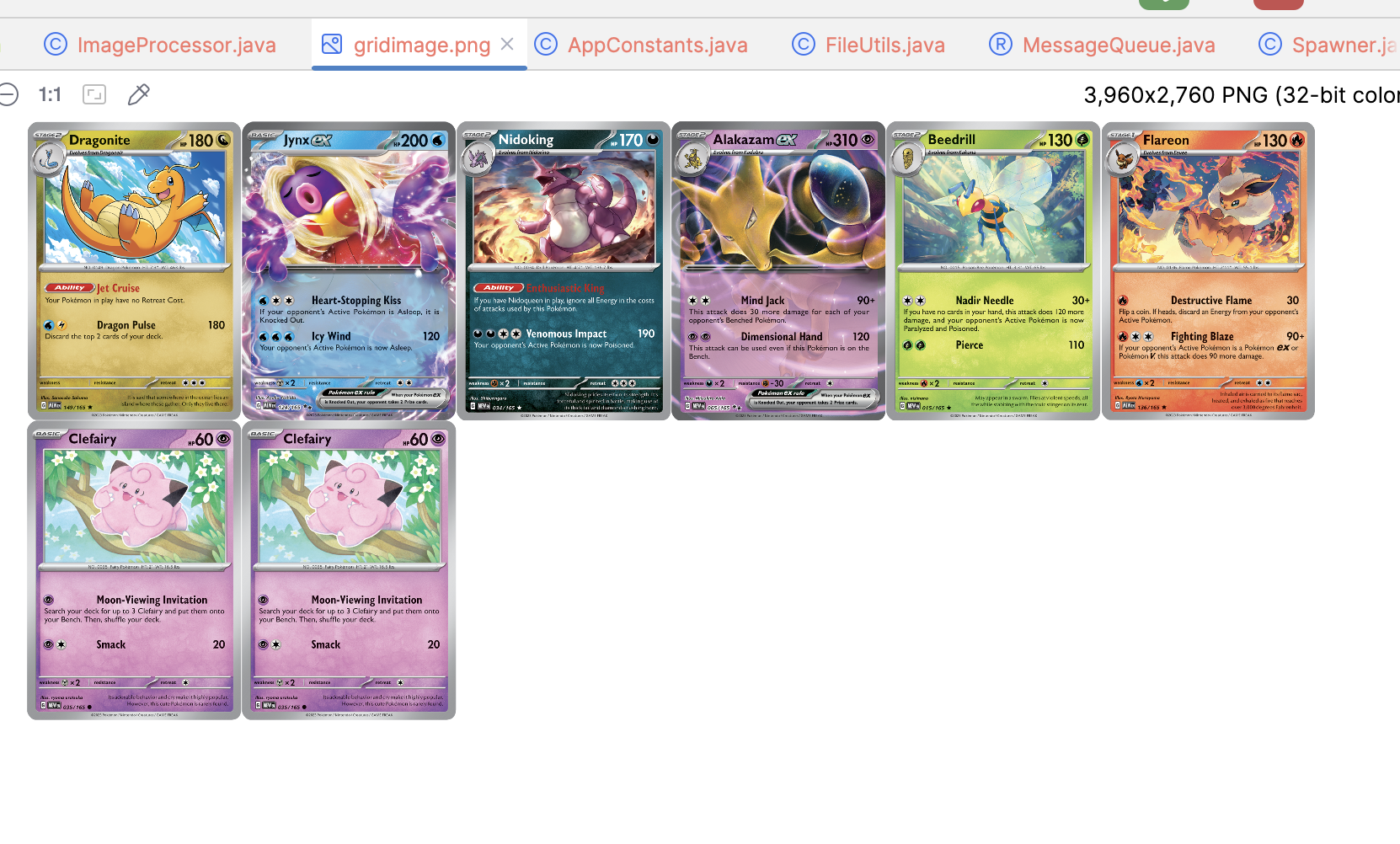
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
i have a small follow up on this
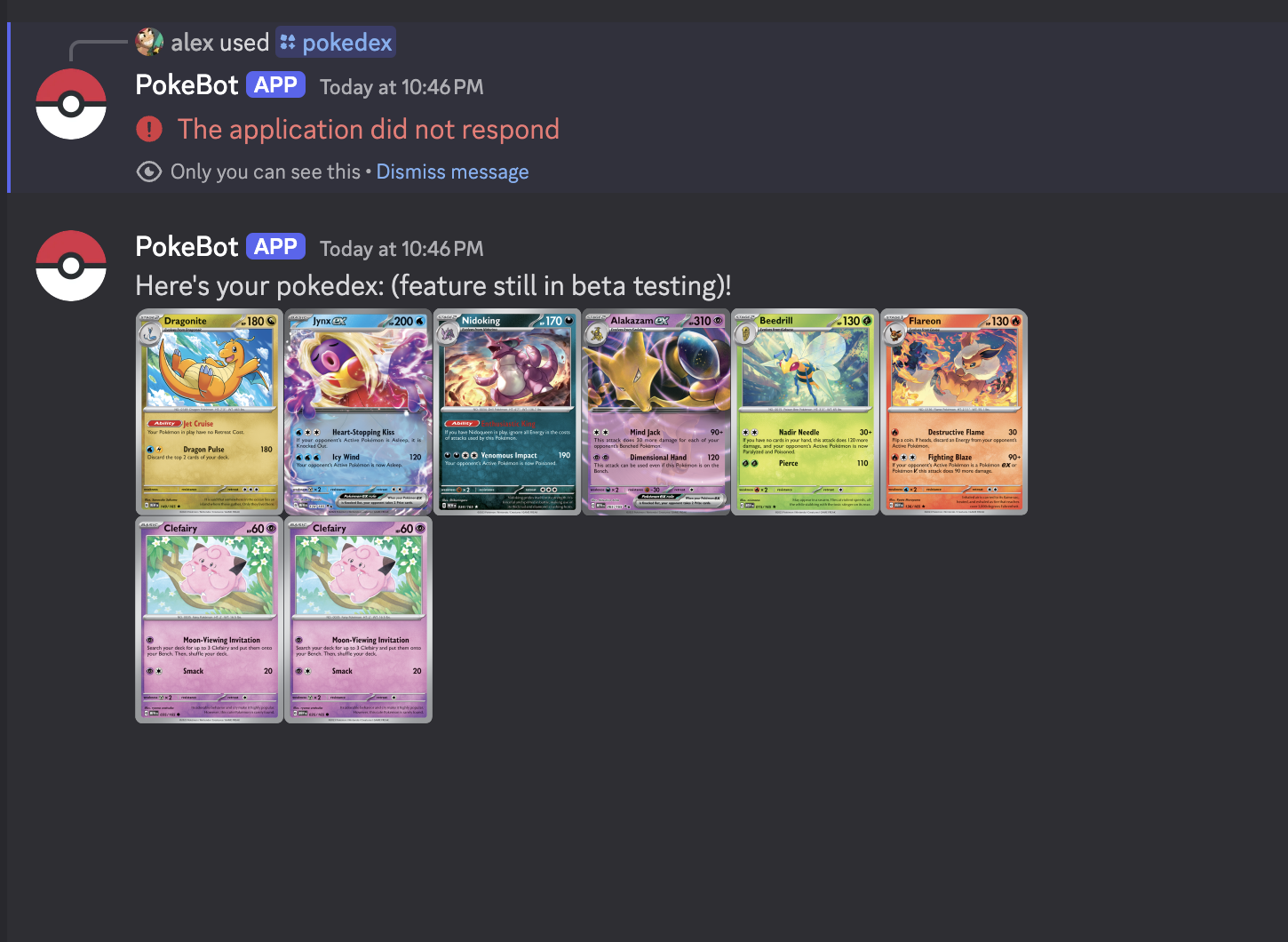
the bot takes so much time to reply back because probably it takes time to process the images, so the application hangs up, do you know a way to prevent this
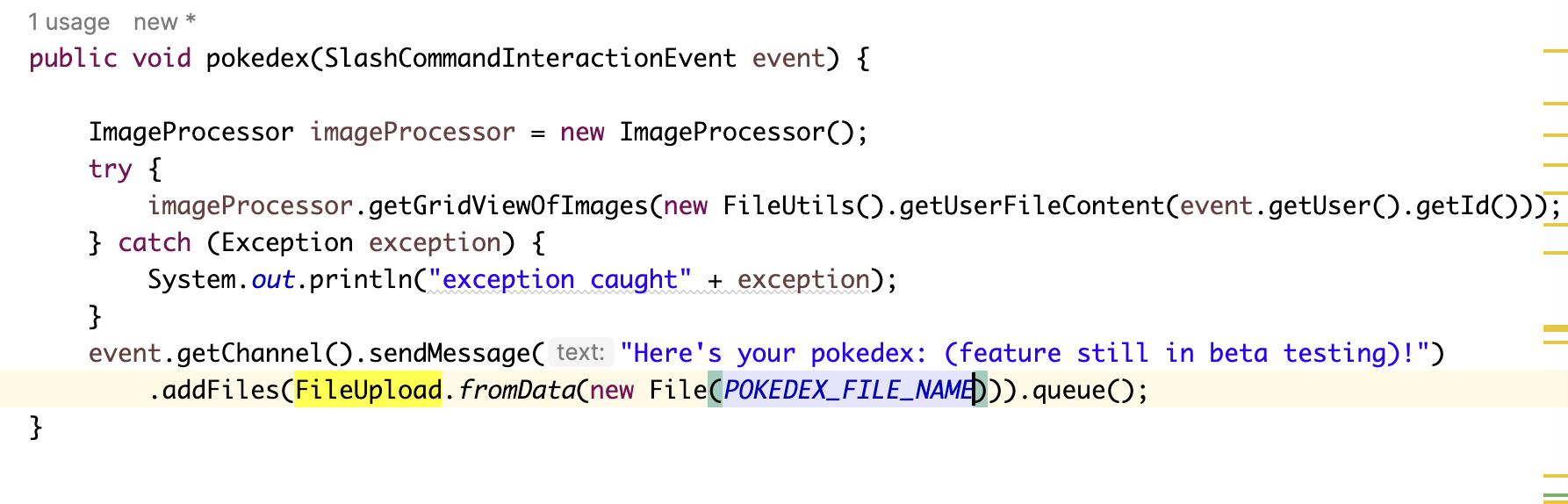
heres the code for reference
image processor generates the file and the last line just replies back the generated file
probably there should be a way to make it wait for longer time
I fixed the above by using deferReply()
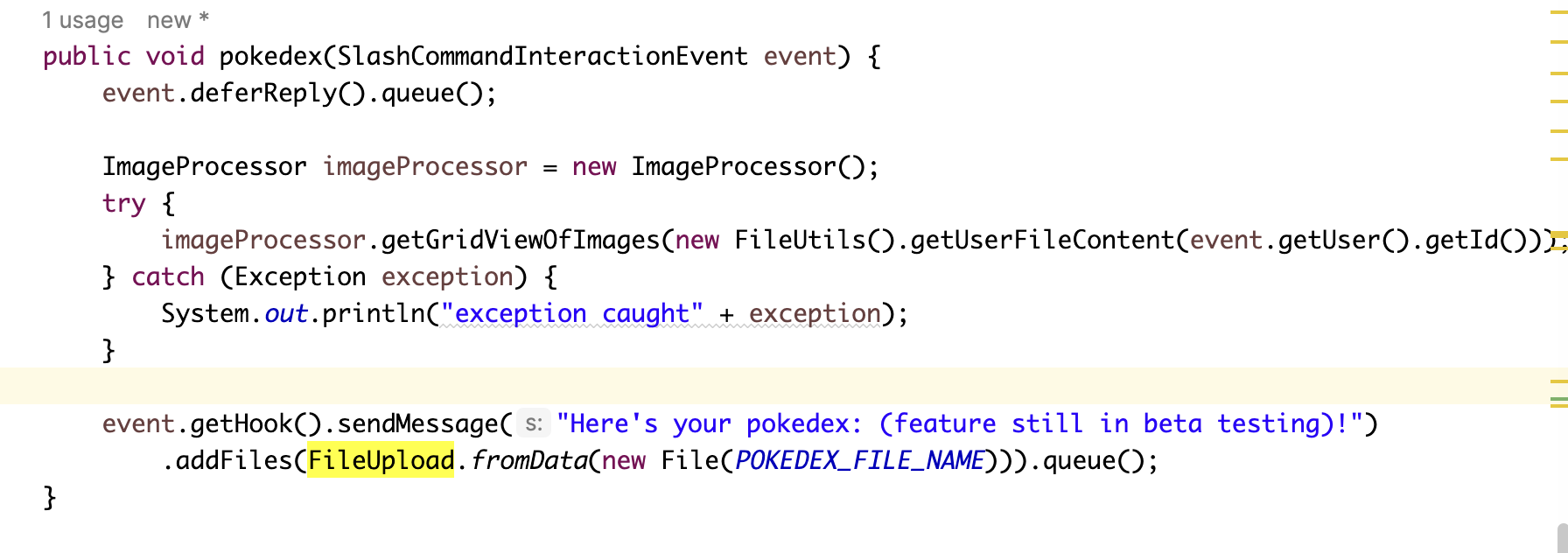
thanks!
If you are finished with your post, please close it.
If you are not, please ignore this message.
Note that you will not be able to send further messages here after this post have been closed but you will be able to create new posts.
Post Closed
This post has been closed by <@650719835310784524>.