Help designin products model spring boot
I'm not really sure how to do it the right way I have products that have different sizes and colors and designs applied to them
35 Replies
⌛
This post has been reserved for your question.
Hey @userexit! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
This message has been formatted automatically. You can disable this using
/preferences
.
this is what I currently have @Peter Rader
Oh you mean the db-model.
yeah
Im just not sure how to do it
a product can have multiple colors
multiple sizes
different stock for each color, size combination
say I have a tshirt with a goku design on it, in color red, with size XL, XS, M
and then a tshirt with a goku design on it, in color black, with size XL, M, S, L, XXL
stuff like this
Show the entity "design"
First, rename the Product into ProductVariant.
Then make the field designs a one2many.
Create a entity Product having a many2one to ProductVariant
why would it be a one2many, the same design for example "Goku vs Gohan" can be used in a tshirt a sweater etc
A tshirt is a different variant than a sweater
Remove the price from the design
it still doesnt click in my mind ill have to draw it by hand brb
no thats actually ok some designs are harder to embroid than others so i want prices for designs then prices for products like a tshirt is going to be x amount then the total price is the price of the tshirt for example + the price of the design
Then increase the price of the productvariant.
Or dont call it price, call it "license_fee"
ok
@Peter Rader Would you use this as a product entity:
and this as product variant:
@startuml
ProductVariant::product_id --> Product::id
ProductVariant::design_id --> Design::id
ProductVariant::size_id --> Size::id
Design2Character::design_id --> Design::id
Design2Character::character_id --> Character::id
@enduml
This message has been formatted automatically. You can disable this using
/preferences
.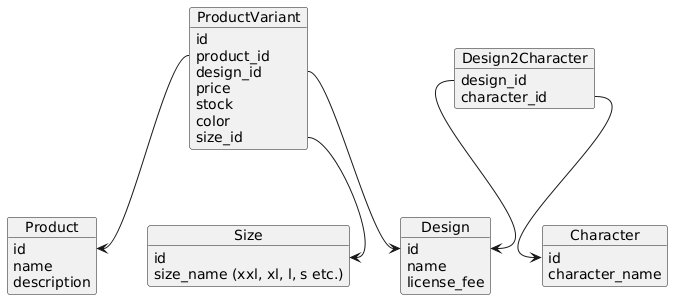
productvariant and design are connected by a many to many ?
No
the only many2many is design2character
because a different design is naturally a different product-variant.
where do you put the category of the product, in Product or in ProductVariant
as in t-shirt, sweater
etc
Hm, I forgot about it.
I would put it in Product
and you can have the same design in a tshirt and in a sweater, thats why i was suggesting the many2many with design
Depends on what you see as a category. If the Category "Wearable" should stick to the product. The category "Winter Closets" should stick to the ProductVariant because you wont wear a tanktop in winter, right?
category is just going to be T-shirt, sweater, crew neck
stuff like that
Then it must stick to the productvariant.
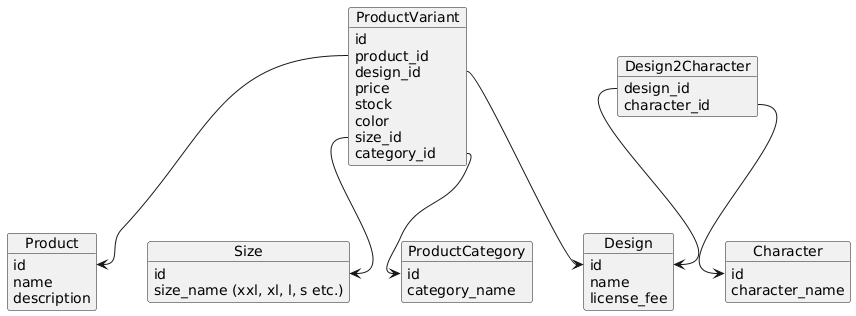
alright and now it is a many2many design and productvariant right
since I can have a tshirt with same design as a hoodie or a crew neck
2 different product variants
same design
No
You have a Sweater with 2 different designs? Can you give an example?
50cent and HelloKitty on one sweater? You are kidding!
hahahah obv not 50cent and hellokitty
but luffy and ace
so the Product thing is useless if I dont need name and description
Know neither luffy nor ace.
Are they rappers?
nah anime characters that are both blood related
say u put Eminem on the front
and Dr dre on the back
if u want the rapper version of it
hmmmmmmmmm
dont you think that could just be a string
that way you dont have to restart the app every time you wanna add a new category
same for the color
wdym with this
oh i thought you use an enum
but yea is there any need to make the category a separate entity
when you can just give it a string
yeah so they can shop by category ig
yea that makes sense then
💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.