Roles & SecurityConfig
Hello so i have 3 roles
and i'm trying to write the authorities etc and here is my config
but for some reason when i hit this endpoint that requries it to be ADMIN such as this endpoint
it doesnt let me even when i'm authenticated and have a session. But when i change the securityconfig to then it works
23 Replies
ā
This post has been reserved for your question.
Hey @Itsurran! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
for testing purposes get rid of the pre authorize annotation
and use this
and check if it works
so i should remove the from controller and let this code still be in config ?
yea
if so i still get 403 in postman
try that
its either not checking that ihave a session when i hit that endpoint or something that reconize the users session aka role or something wrong with securityconfig
but i'm getting a cookie automatiaclly in postman when i authenticate
show the code for user authentication
like
how it checks if a user is authenticated
and im getting a session
when i auth
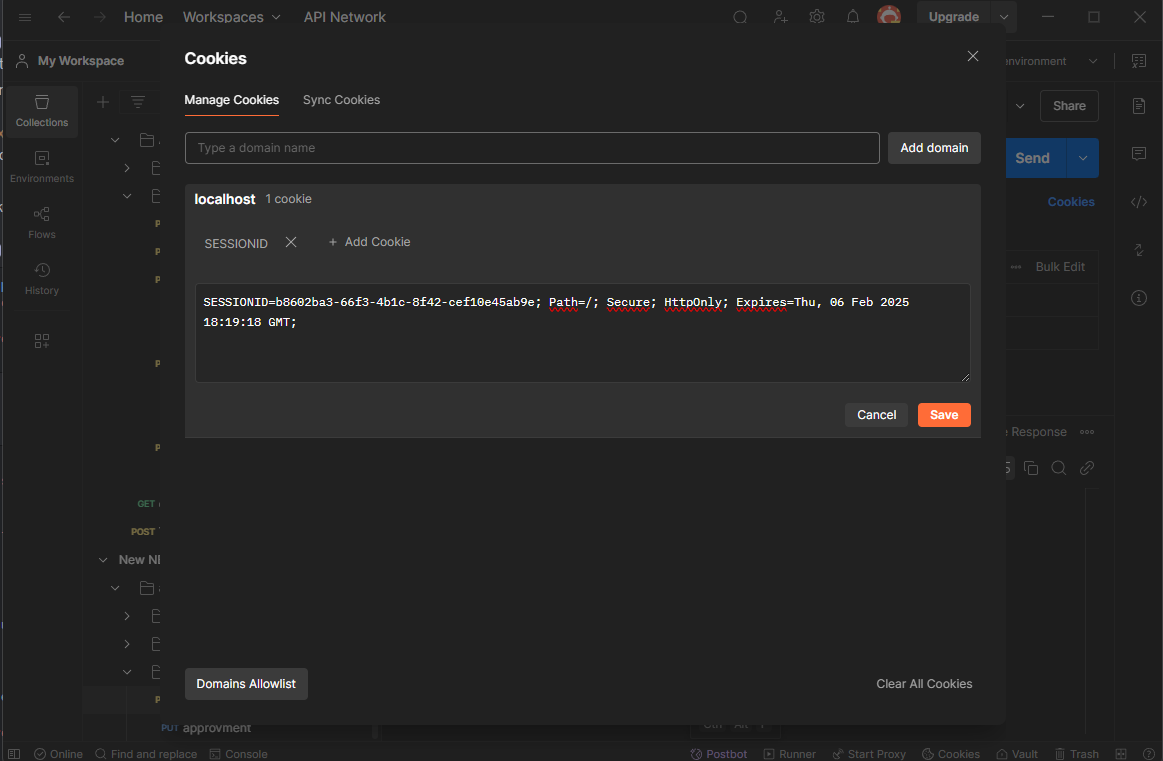
but as i said i think its either not reconizing the session aka role to send that invite post or some bullshit in config right?
ahaaa i think iknow it
l0l
i don't have anything about invite in my adminservice
lmfao
wait
so u dont have any code to check if the user from the cookie has role
or some other disaster
š±
i have a /me that gets the currentuser and by that you need a cookie
hmmm not working
think we need backup danial
can you try with a
test user details
try this
and try to login with credentials
i already have
and i can authenticate
if it works its the fault of ur cookie auth
so show how the cookie stuff works
User admin = User.builder()
.username(adminUsername)
.firstname("Admin")
.lastname("User")
.email("[email protected]")
.password(passwordEncoder.encode("Admin123!"))
.socialSecurityNumber("0000000000")
.phoneNumber("+46123456789")
.street("Default Street 123")
.city("Default City")
.role(Role.ADMIN)
.registrationStatus(RegistrationStatus.APPROVED)
.createdAt(LocalDateTime.now())
.build();
so
the cookie thing
seems to not work
HMM
do u have custom user details
No
got it working
followed Bouali Ali video on youtube where he explained everything and i just followed but did my own way
like my own roles etc
but i think the biggest problem was this
i changed it to
in my user entity
yea probably
it couldnt grab the authorities
if u just returned an empty list
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.