Parameter count mismatch error when trying to change what is being displayed in a Blazor application
As it renders now, the
else if
portion of the code is working. It renders the Assessment. when clicking the edit button I get the error listed in the title. Will put the full error in a comment below this post.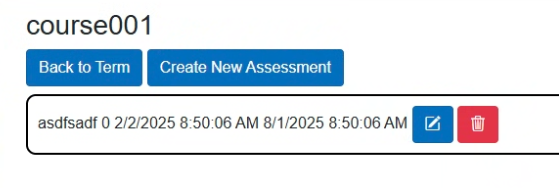
8 Replies
As far as I can tell, it should be monitoring for the changing of the state of the variable and removing the
else if
from displaying and rendering the if
portion, but it errors while still keeping the else if
displayed with the above code. I'm sure I'm misinterpreting how the rendering works but I'm not quite sure how else to handle the displaying/editing in the same lineYou're missing some
@
notations here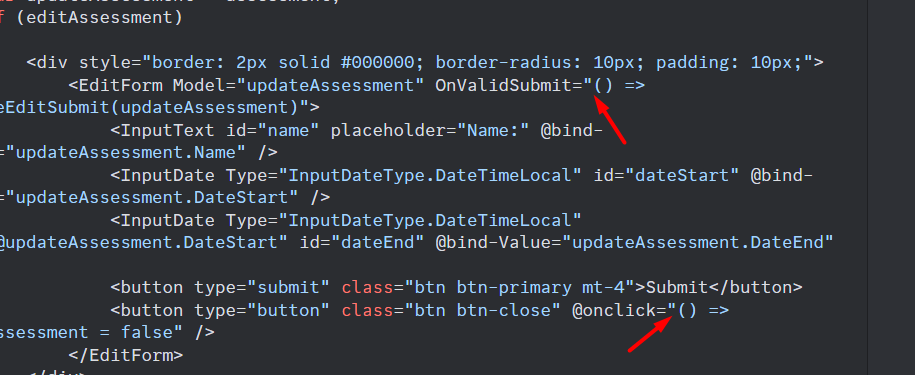
I'm not sure if this could be the issue because it's usually gracefully ignored but at least try
I tried putting
@
in both of those locations but it gets an error for that syntax. On the first, OnValidSubmit
is a Parameter of the Editform component and its syntax is like shown if i'm passing a variable (as far as I can tell), on the second, the@onclick
is to handle the EventCallback for the mouse event and set the local variable, editAssessment
to false
However, that block of code isn't even getting a chance to run when setting breakpoints
Ok, so for some additional information:
if I change
the onclick will throw an error before even attempting to run that. I have a breakpoint there and it never hits for it. It gets the parameter mismatch instantly. If I change that call to
it will run the code, but the value of editAssessment
doesn't change to true like it it set to do, and it never renders the edit block of code as shown below:
Yeah you need to add parentheses
Ok, updated and same effect. Unfortunately I’m not even reaching the point that those elements are displaying. It’s failing prior. I may need to just come up with a different approach. Push the edit to a new page and move on despite it being less than optimal.
Try this.
Yep, still no luck. Never displays the
@if (editAssessment)
block. I'm going to find a new way and move on. Maybe having an edit modal overlay instead would be easier. just a div centered in the middle of the screen being passed an object. How hard can it be lol
Yea, going the modal method was so much easier. I may revisit this later just to figure out why it wouldn't work because its a good learning, but in the vein of just carrying on with my work, modal will be the way
ty for your help, both of you