Deference and Reference in C#
Hello guys, sorry to disturb you all, can someone explain why I obtain the warning "dereferencing possibly null reference" pls.
What do we mean by "deferencing" and "referencing" something?
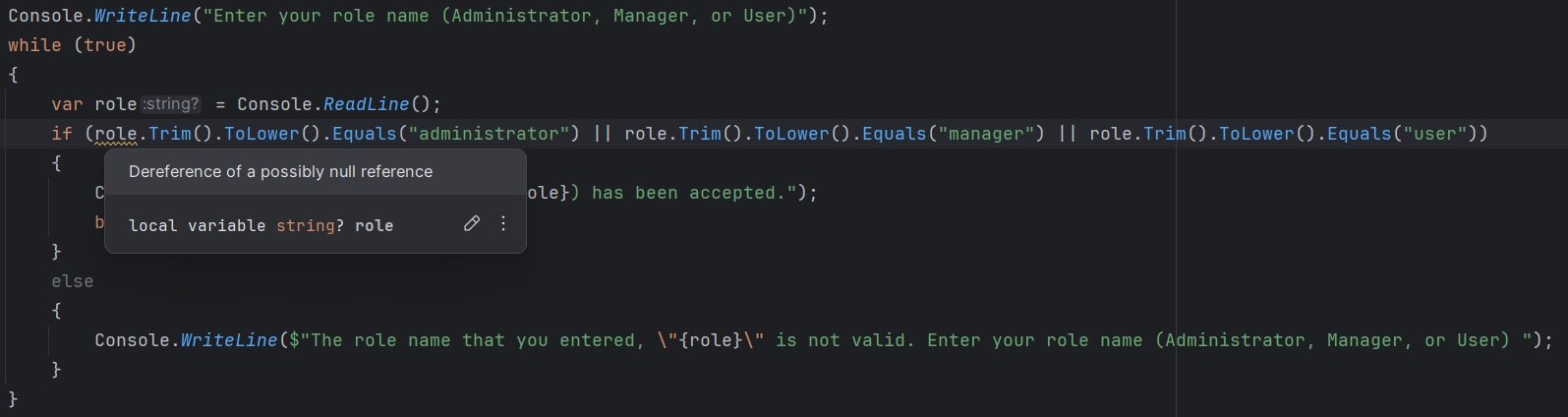
39 Replies
Dereference is when you set something to null or you forget a typed instance
what do you mean by a "typed instance" pls
here I didn't set anything to null but the var keyword assume that role can be null though using the
string?
yeah. cuz Console.ReadLine() can be null
yep I see
what do you mean by typed instance here pls
Also, what's the meaning of "dereference" here? Like we want to retrive the value of the Console.ReadLine ?
means that something exists and is of a certain type
Dereference is just basically telling you the variable can be null. and you can't reference a null variable.
because null is null, it doesn't exist
Dereferencing is Accessing something via a reference
ah, yeah I see
And doing that to null causes null ref exception
yep just read that, when I have such warning, is there a proper way of handling/ checking if the variable isn't null ?
put a ? on a nullable variable
which will basically convert to a
if (variable != null)
or something but C# does the magic for youYesnt. Depends on where you use it.
maybeNull?.Something();
yep
var x = maybeNull?.Value;
returns null if maybeNull was null
hmm here for example, I declared
role
variable, then, what should I do, writing role?
If you do, you'll get an error
Because null isn't a Boolean value
yeah, why is that
you should store
role.Trim().ToLower()
into a variable and check against that
it'll be a lot simpler to handleYeah agreed
Also,
?? false
var role = Console.ReadLine()?.Trim().To lower()
Then you can do if role == "admin"
or whatever
Don't use .Equals for no reason
Silly java habit 🙂hmm for string, it doesn't matter if we use == ? Like I know their is the reference thing
C# isn't java
It's fine to use ==
yeah, I need to understand the syntax, I will just read a bit about the
?
operator then comes back
oh okay, noted, thanks !!
is there a partiuclar scenario where .Equals is more appropriate ?If you need to specify a culture
For strings it's fine, it's pretty random if == is eq by value or ref
ok ok, thanks guys, will just read a bit about the
?
, then came backFor collections use SequenceEqual
better suggestion. switches
ah true, will try with switch statement, I always used if statements, will try to change this time
I modified the code to something like this:
I have one question though, we can't "nest" break statement right? Like I wanted one for the switch case and 1 for the while loop in the same case statement
Also, is there a way to test our fallback mechanism of "unknown" ? Like if I just press enter, it returns me an empty string but not a null reference
Make local functions that return a bool for this
Oh I see, yep, thanks !
no. change Console.ReadLine to ((string?) null) to test
var role = Console.ReadLine((string?)null)?.Trim().ToLower() ?? "Unknown";
Like that ?no literally change the function call for a null
it doesn't take a parameter afaik
the thing is, I tried to assign null to role, like after the data entry, I wrote
role = null;
but it didn't work, is there a reason for that ?role is not nullable because you have a default at the end
?? "Unknown"
change var to string? for that to work
i front of role
so it has a nullable type
hmm but var in this case doesn't act as
string?
it's string not string? because there's a fallback
ahhh
I see
remove the fallback (?? "Unknown")
yep noted, will try to change that
and it will be string?
yep noted, ty !!