PartyKit vs WebSocket server with WebSocket Hibernation
Totally new to setting up a websocket server / client and have a general architecture question.
Wondering how to best implement a web socket server and if it should still be behind my API gateway.
The gateway is built using Kono.
1. Is it possible to proxy the initial call so that it does the 101 Upgrade for websockets in the gateway and then calls the downstream websocket server using RPC?
2. I am using partykit with currently with just the generic
import { WebSocket } from "partysocket";
this works fine directly to a Websocket server direct on the worker but I have issues pushing this through the gateway to do the upgrade and everything.
Here is the excalidraw
https://link.excalidraw.com/readonly/rMHltKc25dPL0AQFvkGf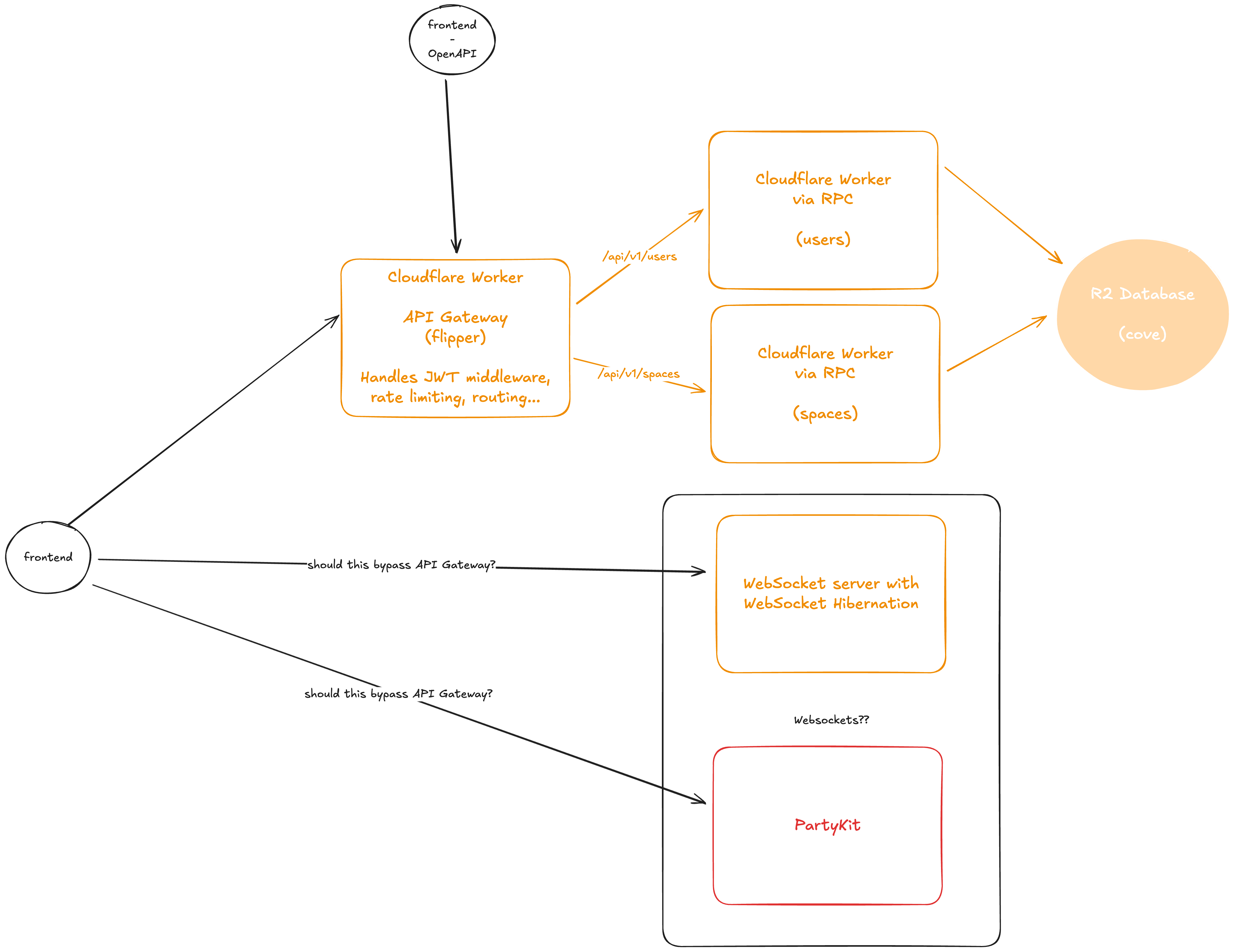
1 Reply
Maybe another way of looking at this, in this example from the docs https://developers.cloudflare.com/durable-objects/examples/websocket-hibernation-server/
Can I move this part to the Gateway, then just call an RPC?
Cloudflare Docs
Build a WebSocket server with WebSocket Hibernation · Cloudflare Du...
Build a WebSocket server using WebSocket Hibernation on Durable Objects and Workers.