Jwt token
I am not able to extract the header from my spring boot app controller
Spring boot backend
React frontend
61 Replies
ā
This post has been reserved for your question.
Hey @Danix! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
um
you are checking if your header has authorization
but the server makes a header named Authorization
yeh it have
or did you fix that
You mean i have to check for the Authorization not for the authorization ?
yes
try that
and see if it helps
let me'
ok
no still the same
show the code
how u changed it
do you have a cors config class
yeh
in my spring boot package
did you expose the authorization header
can you show it
yeh
add this
and check if it works
yeh
sure
nahh still the same
interesting
can you try to add another random header
and try to read it
like
idk
"abc123"
and try to read it in your front end
hm let me
you can also print all the header attributes
see what it says
still undefined for the new header aswell
does this print any headers
no
quite interesting
yeh\
hmmmmmmmmmm
for testing purposes
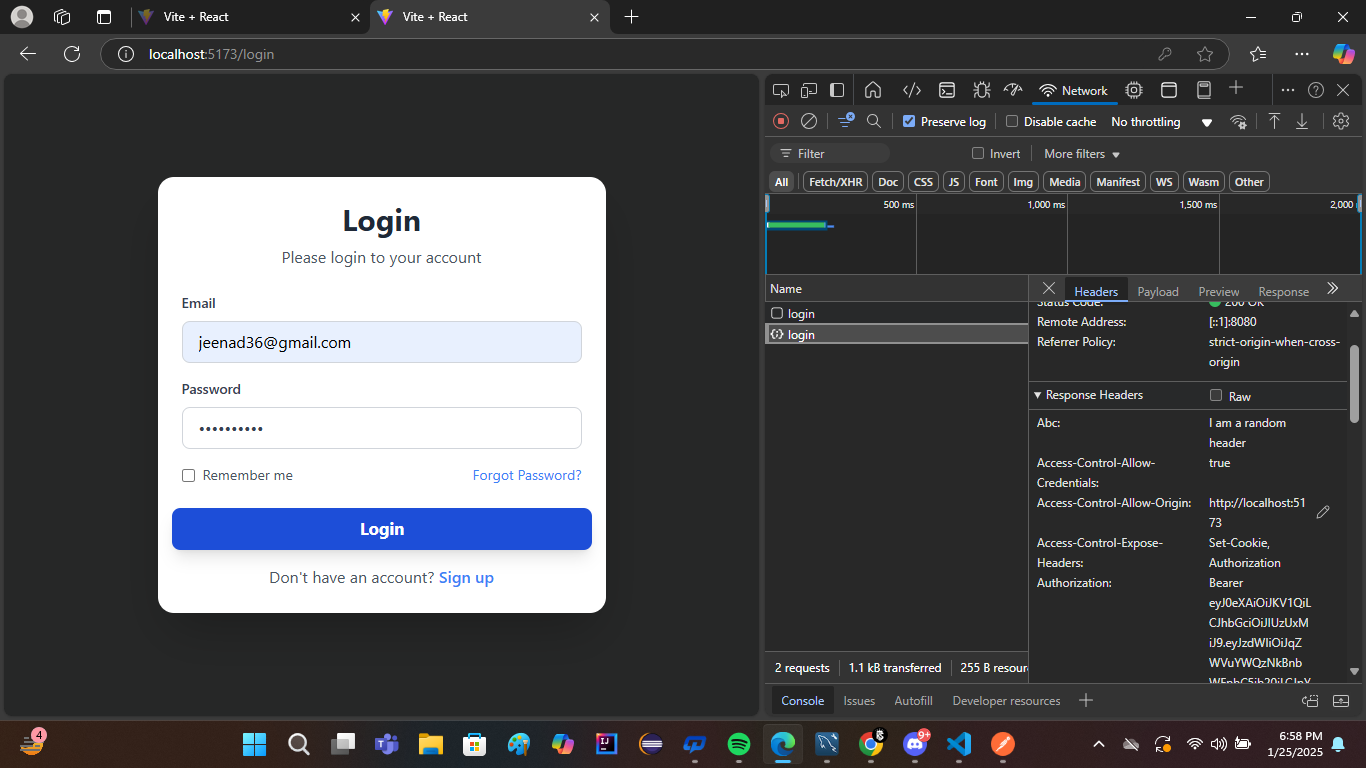
do add allowed origin and your app port
instead of the
Abc is not considered as a header here !
*
š±
what
show the code
that u use
to check if there is abc
header
yea and show frontend
hmmmmmmmm
can you try this config
yeh sure
did it help btw
i am trying it
o
so your app runs at 5173
put 5173 here instead of 3000
i did but i think i need to restart the server
yes
ok so now i got abc header but not the authorization one!
we are progressing
amazing
but the thing is the abc header isn't in the headers list !
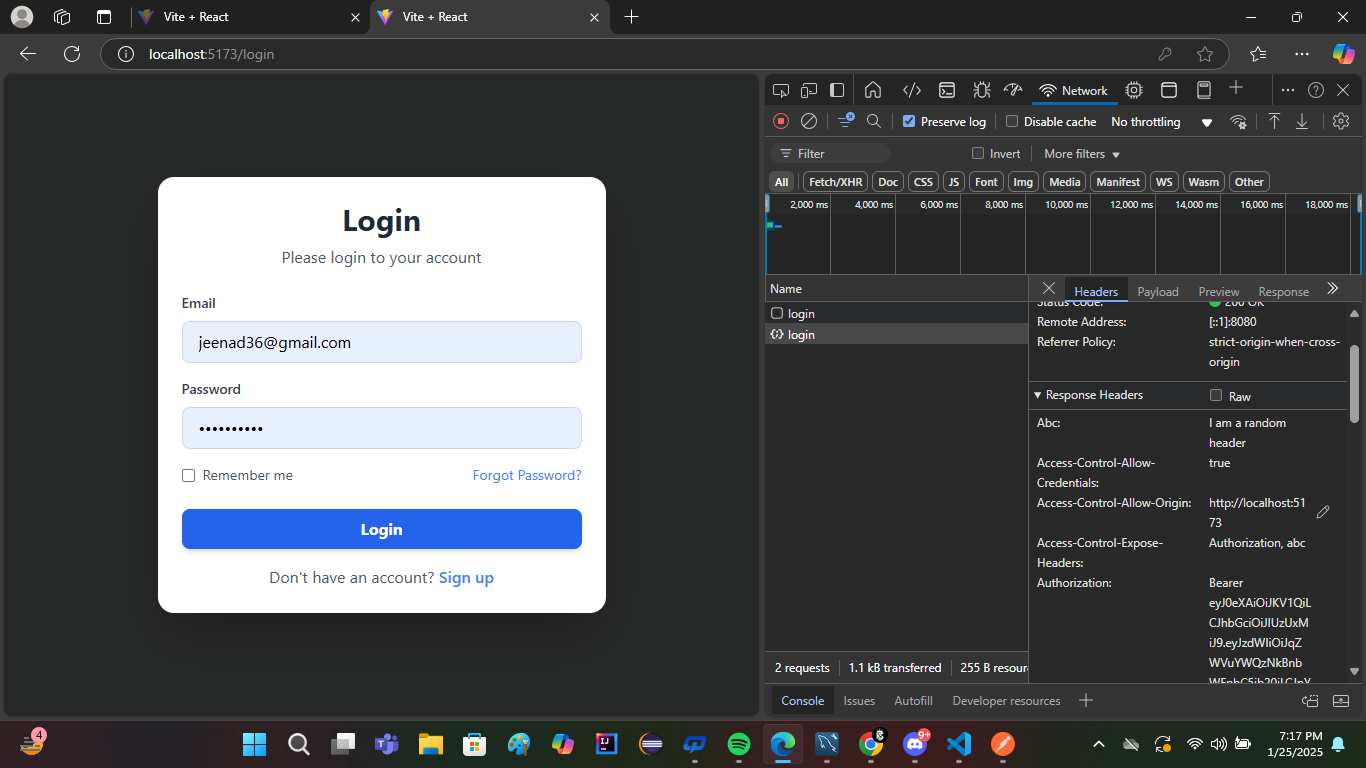
it is
well it kind of is
yeh
i can see the header abc in the headers panel in postman
if i will change the header named from authorization to just auth so that might work ?
hm
try it
and make sure to swap the name in the server
in the exposed headers
nahh still the same undefined shit !
truly a disaster
ok show both codes again
the one with corsfilter and frontend
let me show u my github
so i might be better
ok
lol
what if you
try to do the auth attribute now
wait nevermind
I have a secret api tokejn in my app.properties and git is not allowing me to push the code so what can i do to commit it ?
oh
dont push it then
what if you
rename the abc header
to auth
check if you can read it then
and try to pass to that header the jwt token
yeh let me
with the name of auth i am getting it !
1 sec
I am able to get it via post man
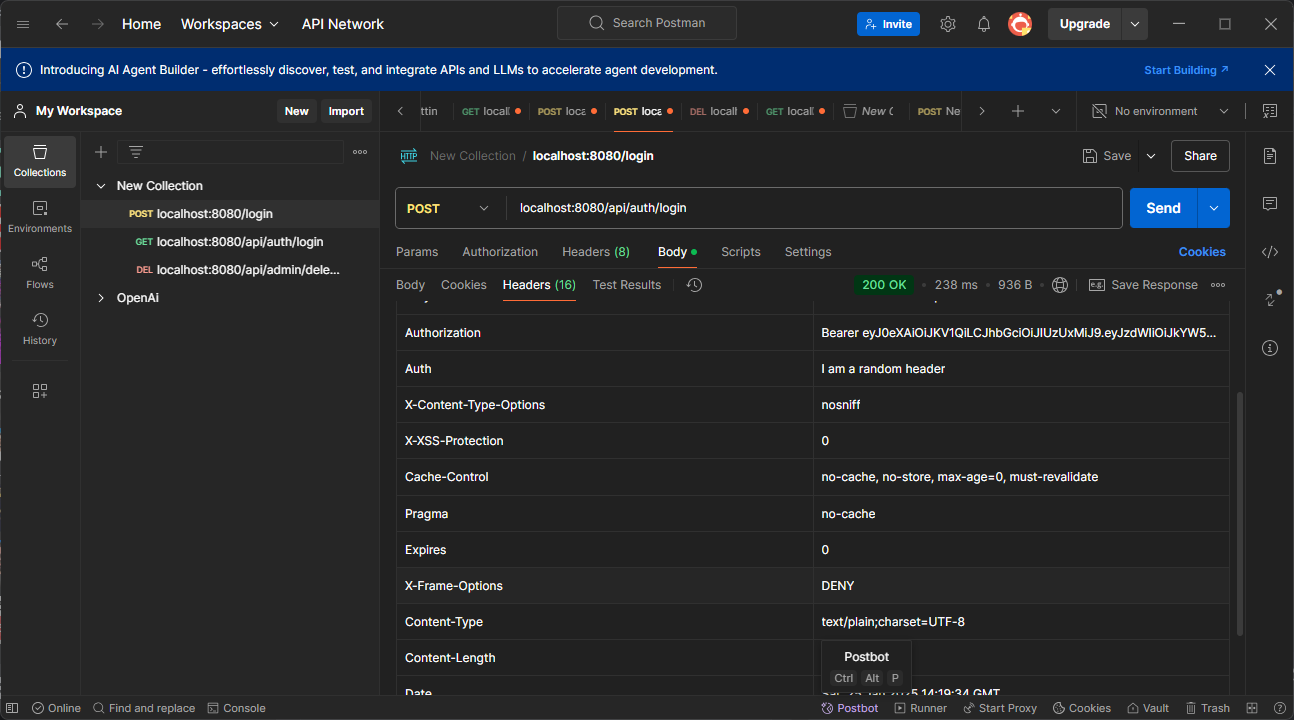
bro we got it
:boohoo:
niceeeeeeeeeeeeeee
real
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.