✅ Issues with canvas
Hey guys, i'm having an issue with canvas placement:
i did this peace of code:
How come making the tiles work, and the inventory y is out of the window:
private void GenerateWorld()
{
WorldHeight = Math.Round(ActualHeight / Tile.Size);
WorldWidth = Math.Round(ActualWidth / Tile.Size);
Random random = new Random(Seed);
List<WeightedItem<TileType>> types = new List<WeightedItem<TileType>>()
{
new WeightedItem<TileType>(TileType.GrassA, 6),
new WeightedItem<TileType>(TileType.GrassB, 5),
new WeightedItem<TileType>(TileType.GrassC, 2)
};
Dispatcher.Invoke(() =>
{
for (int i = 0; i < WorldHeight; i++)
{
for (int j = 0; j < WorldWidth; j++)
{
TileType type = Utilities.GetRandomItem(types, random);
Tile tile = new Tile(Convert.ToDouble(j), Convert.ToDouble(i), type);
Display.Children.Add(tile);
}
}
LoadToolbar();
});
}
private void LoadToolbar()
{
int inventorySlots = (int)(ActualWidth / InventorySlot.Size);
Debug.WriteLine(inventorySlots);
for(int i = 0;i < inventorySlots; i++)
{
InventorySlot inventorySlot = new InventorySlot(i,WorldHeight);
Debug.WriteLine(Canvas.GetTop(inventorySlot)+","+ActualHeight);
Display.Children.Add(inventorySlot);
Debug.WriteLine($"Loaded inventory slots: {i+1}/{inventorySlots}");
}
}
private void GenerateWorld()
{
WorldHeight = Math.Round(ActualHeight / Tile.Size);
WorldWidth = Math.Round(ActualWidth / Tile.Size);
Random random = new Random(Seed);
List<WeightedItem<TileType>> types = new List<WeightedItem<TileType>>()
{
new WeightedItem<TileType>(TileType.GrassA, 6),
new WeightedItem<TileType>(TileType.GrassB, 5),
new WeightedItem<TileType>(TileType.GrassC, 2)
};
Dispatcher.Invoke(() =>
{
for (int i = 0; i < WorldHeight; i++)
{
for (int j = 0; j < WorldWidth; j++)
{
TileType type = Utilities.GetRandomItem(types, random);
Tile tile = new Tile(Convert.ToDouble(j), Convert.ToDouble(i), type);
Display.Children.Add(tile);
}
}
LoadToolbar();
});
}
private void LoadToolbar()
{
int inventorySlots = (int)(ActualWidth / InventorySlot.Size);
Debug.WriteLine(inventorySlots);
for(int i = 0;i < inventorySlots; i++)
{
InventorySlot inventorySlot = new InventorySlot(i,WorldHeight);
Debug.WriteLine(Canvas.GetTop(inventorySlot)+","+ActualHeight);
Display.Children.Add(inventorySlot);
Debug.WriteLine($"Loaded inventory slots: {i+1}/{inventorySlots}");
}
}
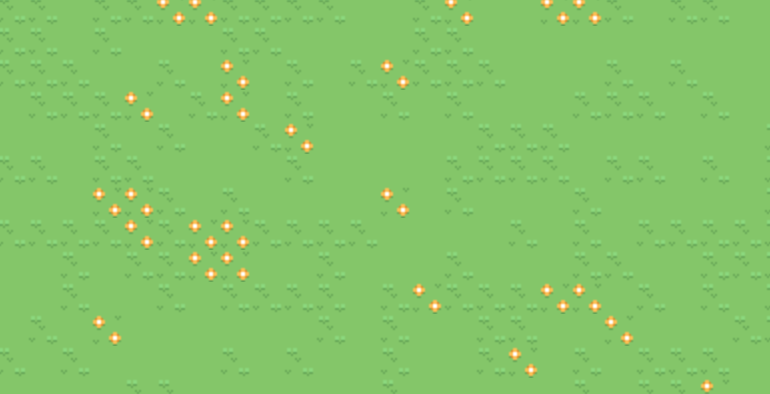
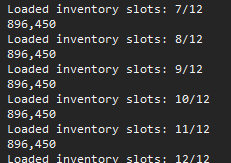
1 Reply
public class Tile : Image
{
public TileType Type { get;}
public static int Size { get; set; }=32;
public double X {
get { return Canvas.GetLeft(this) / Width; }
set { Canvas.SetLeft(this, value * Width); }
}
public double Y
{
get { return Canvas.GetTop(this) / Height; }
set { Canvas.SetTop(this, value * Height); }
}
public Tile() {
Height = Size;
Width = Size;
Type=TileType.GrassA;
}
public Tile(double x, double y,TileType type) : this()
{
X = x;
Y = y;
Type=type;
ImageBrush brush = new ImageBrush();
brush.ImageSource = new BitmapImage(new Uri(GetImagePath(Type),UriKind.RelativeOrAbsolute));
Source = brush.ImageSource;
MouseLeftButtonDown += TileClicked;
}
public static string GetImagePath(TileType type)
{
switch(type)
{
case TileType.GrassA:
return "tile_0000.png";
case TileType.GrassB:
return "tile_0001.png";
case TileType.GrassC:
return "tile_0002.png";
default:
throw new ArgumentOutOfRangeException(nameof(type), type, null);
}
}
private void TileClicked(object sender, EventArgs e)
{
switch (Type)
{
case TileType.GrassA:
return;
case TileType.GrassB:
return;
case TileType.GrassC:
return;
}
}
}
public class Tile : Image
{
public TileType Type { get;}
public static int Size { get; set; }=32;
public double X {
get { return Canvas.GetLeft(this) / Width; }
set { Canvas.SetLeft(this, value * Width); }
}
public double Y
{
get { return Canvas.GetTop(this) / Height; }
set { Canvas.SetTop(this, value * Height); }
}
public Tile() {
Height = Size;
Width = Size;
Type=TileType.GrassA;
}
public Tile(double x, double y,TileType type) : this()
{
X = x;
Y = y;
Type=type;
ImageBrush brush = new ImageBrush();
brush.ImageSource = new BitmapImage(new Uri(GetImagePath(Type),UriKind.RelativeOrAbsolute));
Source = brush.ImageSource;
MouseLeftButtonDown += TileClicked;
}
public static string GetImagePath(TileType type)
{
switch(type)
{
case TileType.GrassA:
return "tile_0000.png";
case TileType.GrassB:
return "tile_0001.png";
case TileType.GrassC:
return "tile_0002.png";
default:
throw new ArgumentOutOfRangeException(nameof(type), type, null);
}
}
private void TileClicked(object sender, EventArgs e)
{
switch (Type)
{
case TileType.GrassA:
return;
case TileType.GrassB:
return;
case TileType.GrassC:
return;
}
}
}
public class InventorySlot : Button
{
public static int Size { get; set; } = 64;
public double X
{
get { return Canvas.GetLeft(this) / Width; }
set { Canvas.SetLeft(this, value * Width); }
}
public double Y
{
get { return Canvas.GetTop(this) / Height; }
set { Canvas.SetTop(this, value * Height); }
}
public InventorySlot()
{
Height = Size;
Width = Size;
}
public InventorySlot(double x, double y) : this()
{
X = x;
Y = y;
Click += Clicked;
}
private void Clicked(object sender, EventArgs e)
{
}
}
public class InventorySlot : Button
{
public static int Size { get; set; } = 64;
public double X
{
get { return Canvas.GetLeft(this) / Width; }
set { Canvas.SetLeft(this, value * Width); }
}
public double Y
{
get { return Canvas.GetTop(this) / Height; }
set { Canvas.SetTop(this, value * Height); }
}
public InventorySlot()
{
Height = Size;
Width = Size;
}
public InventorySlot(double x, double y) : this()
{
X = x;
Y = y;
Click += Clicked;
}
private void Clicked(object sender, EventArgs e)
{
}
}