Protected routes
Hello!
I'd like to achieve a route for example
/admin
which should be only accessible for "admin" role users. If the user have "user" role, they should be redirected to the home page.
Also I'd followed the docs, but I don't know how to make the /sign-in
route inaccessible for those who are already signed in.
Later I'd like more complex role based access control for routes. How to achieve this?30 Replies
Nextjs supports all this functionality
I wanted to know how to do it.
There are 3 ways to do this, you can do it Client side with the useSession hook, through middleware, or through the route itself
What is your setup
Here's my middleware:
This one will not let you go in the /login if you're already logged in. You can just extend that by adding in the notAuthenticated array if you want to disable sign ups or any other pages.
I'm wondering why the docs are recommending sending an entire fetch request in middleware when you already have the cookie
Yea so it looks like you need to use better-fetch in middleware to get the latest verified session data, however I made a helper function that checks the Cookie directly and verifies it without needing an extra request, so if you need extremely low latency middleware for some reason
middleware.ts
This won't respect if you get "logged out" from another computer like during a reset password flow or something though, just a low latency function if you need rapid verification or less HTTP requests / queries in general and don't care too much about log out others feature
It will first try to verify the session if session_data cookie is present, and if that cookie is not present or it fails (expired session) then it will fallback to send a request to get-session if the session_token cookie is present
If you are using a static export or need to do it client side then ..
Thanks! I will try out your approach for sure
@daveycodez I actually just realized, that I don't understand your explaination
This is your middleware.ts
Or this one can also be the middleware.ts
What's this?
Also I don't really know how to create an adminroute from this. I mean I tried, but I got errors
The difference with my solution is that it uses cookies first before sending the fetch request
It's not good practice to use HTTP requests in Next.js middleware, it should be optimistic routing
Okay, I just realized what you were talking about here
I had problem with this:
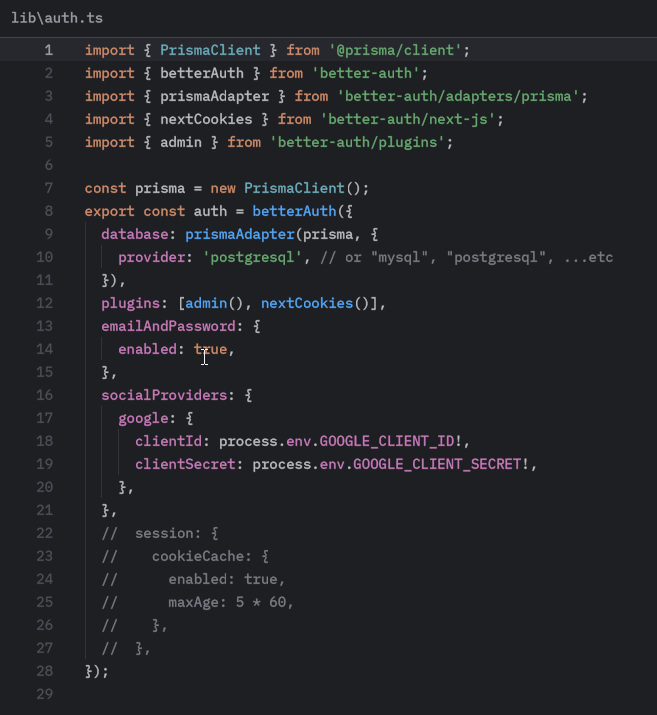
The commented part
It complitely ruined the terminate session-like stuff
That enforces the session to be alive for 5 minutes even if it gets logged out elsewhere
This is my middleware.ts for now. It gets the job done, but I don't know if I did anything that's not supposed to be
If the matcher is just those 3 paths its probably fine
Just need to be careful if you end up using middleware for everything (internationalization or something)
Well I'll have the dashboard which is the user's settings page.
I'll have the admin routes for admin users, and the sign in page is no longer accessible if the user is logged in.
I think this is all for now
Yea as long as its not sending out a fetch request on all of your routes it can be ok
Later I will have buttons that will have an action, but won't work without sessions. I think I don't need the middleware for that.
I'm doing my Uni thesis, and that's what I need it for, but I need my app to be insanely fast (at least the teacher should think that it is)
So that's why I wanted to know if there's a better way of implementing this
Because performance is what I need mostly
If you want faster performance for those 3 pages specifically you can use the cookie
"optimistic" middleware which would just check if the cookie exists at all, then the routes themselves will handle getting the current user
request.cookies.has("better-auth.session_data")
I don't find performance issues just yet but I want to implement metrics somehow to actually see if the performance of the page.
If I find issues with performance for these routes, I will implement what you adviced
Well right now if you refresh an admin page or navigate an admin page you will have this...
fetch -> /admin -> fetch ->/api/get-session-> Query database
If you do the cookie method it will just be
fetch -> /admin -> check cookie
But does the cookie method have any disadvantages?
Well middleware is mainly meant to be used for "optimistic" routing and not protection itself
the protection should live on the route itself, where you'll get the session on the route and handle it over there
the middleware just optimistically decides whether the user is logged in or not
the cookie only gets set if they are logged in, but it could be "expired"
https://www.youtube.com/watch?v=N_sUsq_y10U
This video covers how they handle authentication best practices if you were to "roll your own" auth, but shows examples of how routes protect themselves and middleware is used for optimistic routing
This is from the Next.js head of developer relations
So pretty much the middleware says -> Hey, the user isn't even logged in, so just skip loading the route.
Understandable, great to know!
Could I contact you in private for not so better-auth connected questions about NextJS?
Yea that's no prob
This is how I think I'm going to be handling middleware routing
But yea I'm going to make a GitHub issue to update the better-auth docs or something because fetch requests in middleware or Edge is high latency
I barely found anything in the docs about how to structure an optimal middleware.
Yea better-auth is platform agnostic so we're still figuring out some of the platform specific optimizations
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
I think you would still have the cookie here in your middleware, so you can just check if the cookie exists
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View
Did you guys try these auth settings to get cross-domain cookies? I was using Bearer for Capacitor localhost since it isnt hosted on the domain, but with these settings, I don't need Bearer
Unknown User•3mo ago
Message Not Public
Sign In & Join Server To View