How to properly use "fetchReply" in Discord.js Interaction API?
While working on my Discord.js bot, I encountered the following warning:
-# (node:12684) Warning: Supplying "fetchReply" for interaction response options is deprecated. Utilize "withResponse" instead or fetch the response after using the method.
30 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!I’m using
fetchReply: true
to fetch the interaction response message, as shown in the example below and its work
It’s just a warning, but the warning is correct
I’ve tried replacing fetchReply with withResponse or fetching the reply after calling interaction.reply() as suggested in the warning, but neither approach works.
Since ur only using it to make a collector, use withResponse instead
Are you sure you aren’t using fetchReply elsewhere?
its dont work
Are you sure you aren’t using fetchReply elsewhere?
The warning shows any time you use fetchReply for the first time when running the code
sure
You don’t have any other command?
have
It’s easier just to use the project-wide search bar if on VSC
I don't understand how this could be related, but yes, I don't use it anywhere
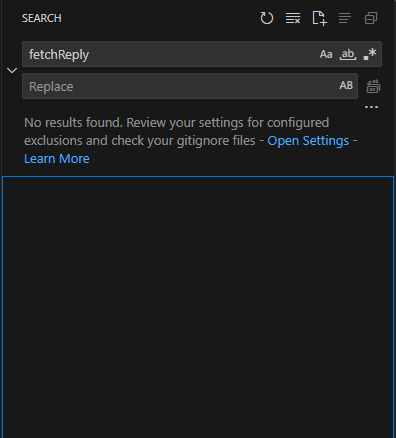
if i use withResponse: true - my collector doesn't work
if i change withResponse on fetchReply - i see id in console
Can you run the bot with the trace-deprecation flag? i.e.
node --trace-deprecation index.js
nothing

stop
sorry
it's without fetchREply
Warning went away?
strange but yes, but it doesn't matter, the problem is not in the warning but in the fact that the code does not work

Is there an error?
no
ok
when i use withResponse i get
InteractionCallbackResponse
and fetchReply return message
and if i use withResponse
i get an error with method createMessageComponentCollector
Property 'createMessageComponentCollector' does not exist on type 'InteractionCallbackResponse'Actually, just don’t enable anything
?
!
This is pointless advice
How?
That’s just how the reply method behaves
InteractionResponse is only returned when you don’t enable withResponse and withReply
Well yeah, because it's not a method on
InteractionCallbackResponse
, do <response>.resources!.message!.createMessageComponentCollector
Not that you needed fetchReply in the first place
Unless you were on a much older version that had fetchReply, but didn’t return anything w/o fetchReply
Thank you!!!
:emoji_52: