unit testing problem with ICache
so I am getting this error
for this method
can anyone help how to fix this please?
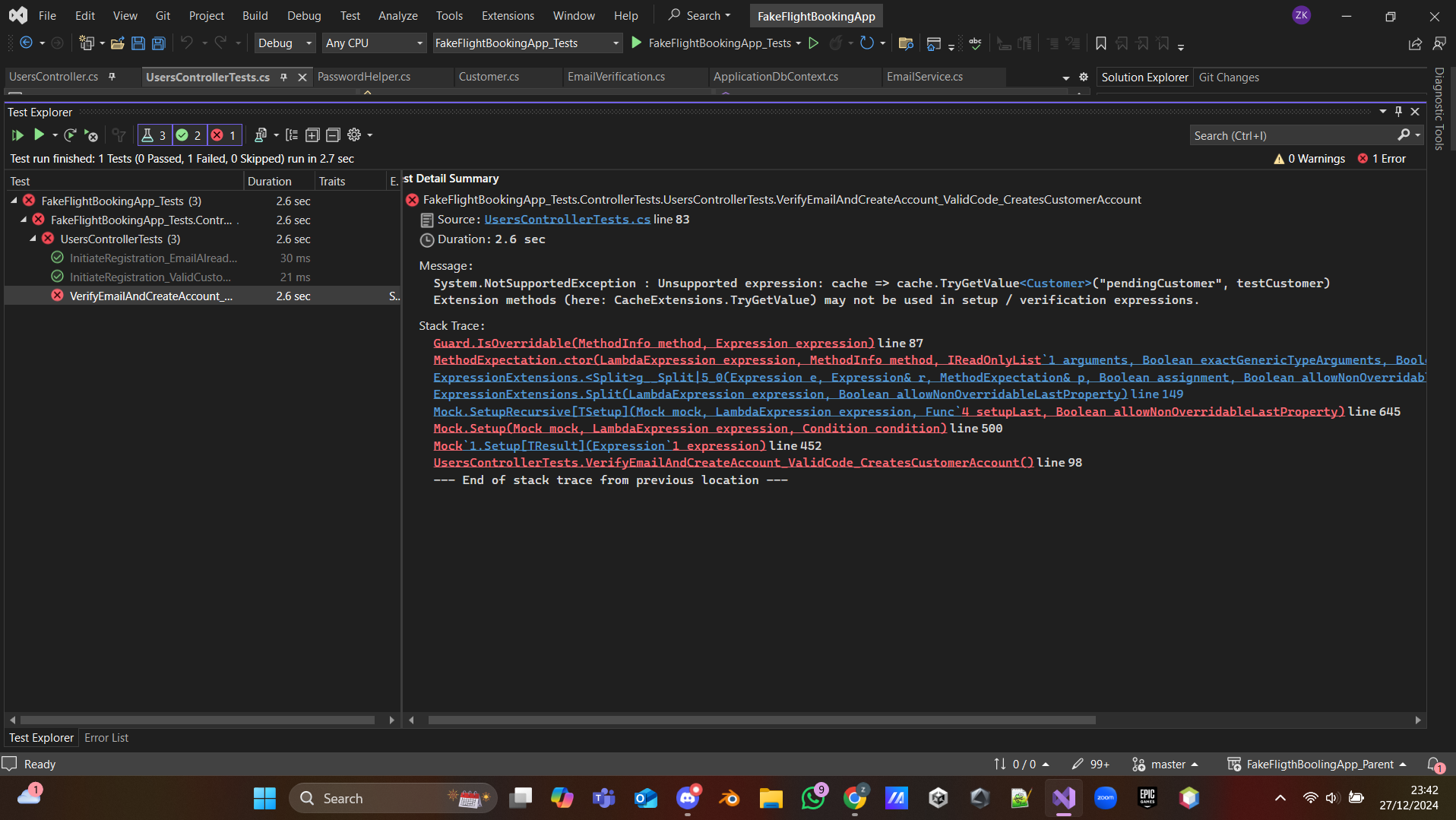
2 Replies
It.IsAny<Customer> something like this u may have to define in the mock setup
extension methods are static methods, and static methods can't be mocked. only interface or virtual methods can be intercepted/mocked
you would need to look at the implementation of CacheExtensions.TryGetValue to see what 'real' code in IMemoryCache it's relying on
and then mock those out
alternatively, couldn't you just make a real memorycache for your test? by definition it's in-memory, so there shouldn't be any issues in creating and destroying one per test...