Issue with Channel Filtering and Counting Logic
Hi everyone,
I'm facing an issue with my Discord.js code where I'm trying to implement a system that counts user messages only in specific allowed channels. However, even when the channel is not in the list of
Here's the relevant code snippet:
The problem:
Even when the message comes from a channel that isn't in the
Could someone help me figure out what might be going wrong?
Thanks in advance! 😊
I'm facing an issue with my Discord.js code where I'm trying to implement a system that counts user messages only in specific allowed channels. However, even when the channel is not in the list of
allowedChannels
, it still increments the count for the user.Here's the relevant code snippet:
The problem:
Even when the message comes from a channel that isn't in the
allowedChannels
list, the code still increments the count for the user.Could someone help me figure out what might be going wrong?
Thanks in advance! 😊
27 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPcan you console.log
allowedChannels
Allowed Channels: [ '1320870580039450686' ]
Message Channel ID: 1321080806419988531
Skipping channel: 1321080806419988531
(no skipping)
Are you sure the code is executed? Try to add a console log inside the condition
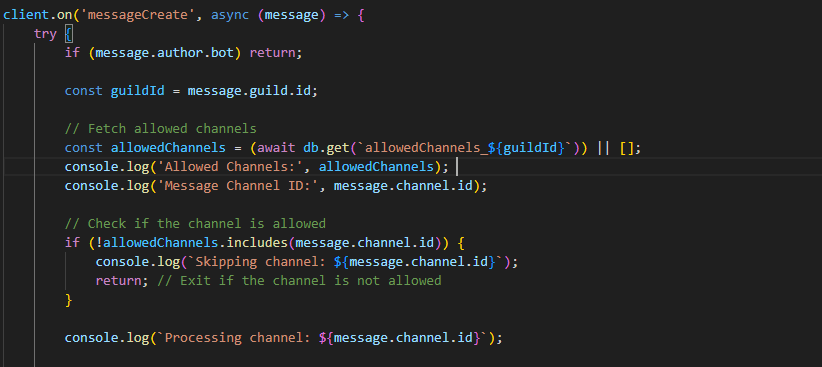
then it worked?
it skipped
no
unless you have another counting somewhere else
Issue is
!
The code will execute if it doesn't include the channelthis channel not allowed
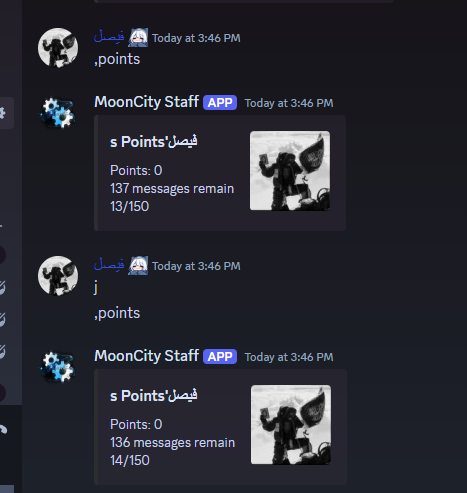
i dont think so? he wants to skip it if its not in the list
Oh, right. Well got confused from the first code
Do you see the Processing channel log?
well do you have any other pieces of code that might be running outside of that try ... catch?
i receive a lot of messages, but I'm sure it says they've been skipped
no
So it definitely not that part of the code increments the counter
There are no other parts to increase the counter
Do you have another process running? Try to turn your bot off and send the command
Pastebin
const { Client, GatewayIntentBits, SlashCommandBuilder, Collection,...
Pastebin.com is the number one paste tool since 2002. Pastebin is a website where you can store text online for a set period of time.
This is the whole code
no what he meant was do you have another instance of the bot running
no
just this one
Line 535
You do increase the count without any checks in you second
messageCreate
listener
That's why we don't recommend duplicating listeners, it's easier to duplicate codes that way*we don't
Thank you, I remembered why I separated them. I separated the listeners because I didn't want to apply the (count) conditions to the commands, and that's how I figured out the solution and the problem. Thank you all! :1980_11:
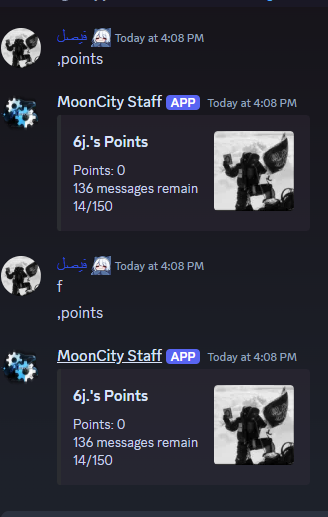