Puzzled about Nullability in EFCore
"Non-nullable property 'Owner' must contain a non-null value when exiting constructor. Consider adding the 'required' modifier or declaring the property as nullable."
I get this warning constantly, and sometimes it causes EFcore to error out when I try to apply migrations.
Say for example I have a
Post
entity that may or may not have an uploaded cover UpCover
How can I account for this on both sides of the relationship?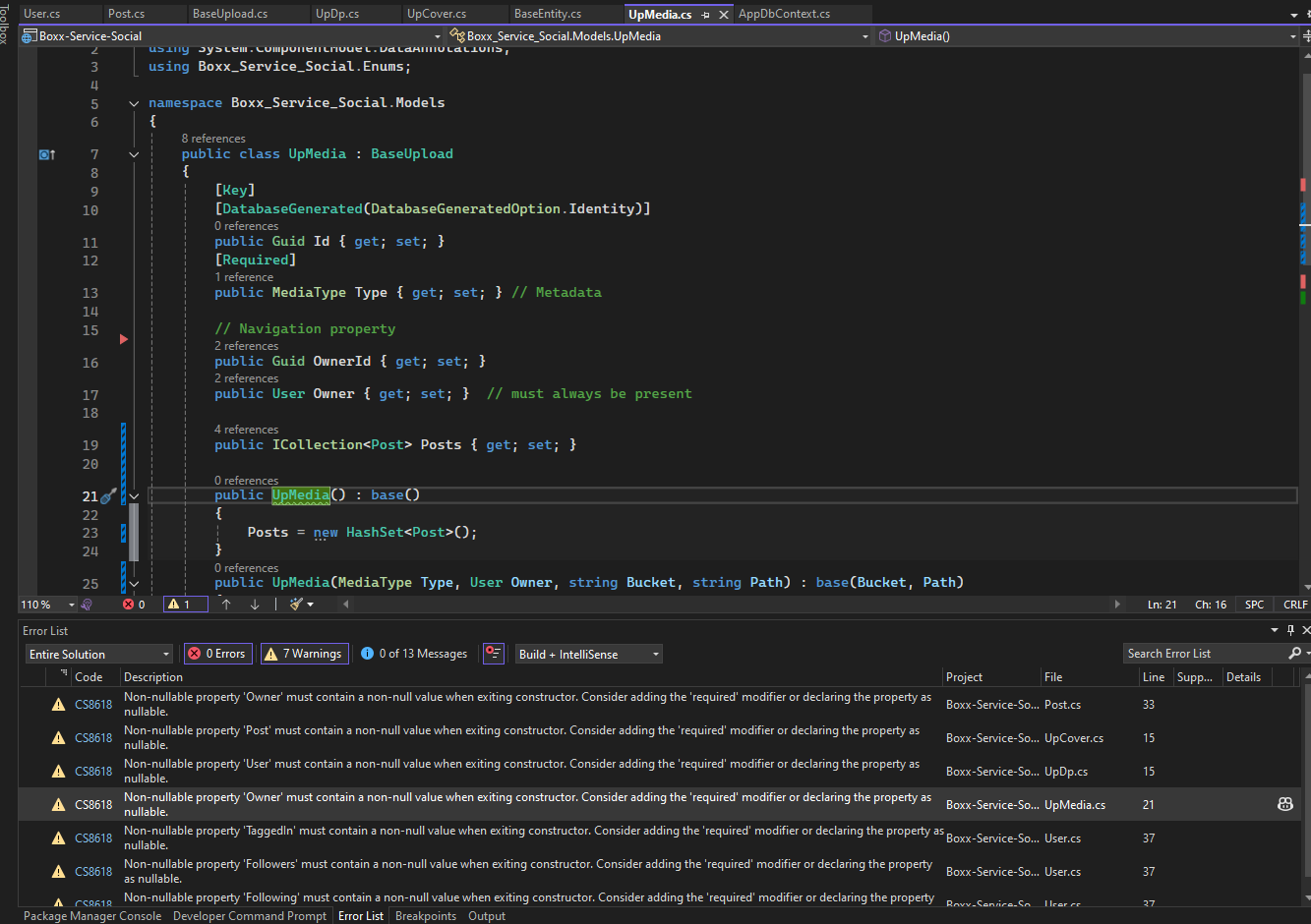
28 Replies
UpCover.cs:
AppDbContext
Is making the nav property nullable on one side only a correct way to resolve this?
yes
i follow these rules
* all navigation properties from a dependent to a principal are nullable (e.g.
Cover
is always nullable, even if the relationship is required)
* all navigation properties from a principal to its dependents is a non-nullable List<T>
with a default value of []
all navigation properties from a principal to its dependents is a non-nullable List<T> with a default value of []
This is only for one-to-many, no? Many-to-many would be different?so a post must have an owner, so is setting required modifier here correct?
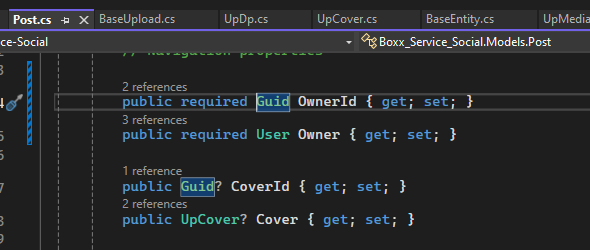
I personally like to explicitly type my many to many relationships, so for me if
A
and B
have a many-to-many relationship through the join table AB
then I have
and then configure them with HasMany(a => a.Bs).WithMany(b => b.As).UsingEntity<AB>()
using the fluent api
EF doesn't care about required
. If the property is a non-nullable guid, it'll be required in the database
required
requires you to supply a value when initializing an instance of the classI did this for a different many-to-many, is this correct?
also if I set nullable, should I set it on both the object and the Id or just the object
not sure, i use explicit join types
object is always nullable
what are explicit join types
having a class
AB
to represent the join table between A
and B
so just Guid needed to become Guid?
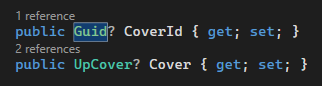
yes
you manually create the intermediary table?
yes
I think what @surwren has is the same just her way is telling ef that the table name is to be PostMedia.
Just letting ef create the table for the relationship of many to many
the difference is that if you explicitly create a type and
DbSet
for it then you can query the join table directly and also add more columns on to it if you need tocan you show an example of the proper way to do a manual table?
I have something similar in UserSubscriptions but idk if it is correct:
idk if this is right
looks like it could work. does it?
this is the general form of what i do
It doesn't throw any errors, I haven't tried adding to it yet
but you said you do manually this way
which is very different from the syntax I just posted
I'm confused
not really, in your case
A
and B
are just the same entityare you referring to the custom relationship for
Subscribers
or Followers
because Followers is kind of like your example
whereas subscribers is what I would call a custom table (UserSubscription)A
and B
are both User
yes
for
Followers
it uses similar syntax as you do, except without the []
creation bc it uses a hashset
for UserSubscription
it is an entirely custom table
can you elaborate on which style you are referring to when you say custom tablei'm referring to what i described and posted pseudocode for
it's not going to be 100% analagous to your case because your case doesn't have two different entities being joined
what you posted is an example of an explicit join entity, yes
can you explain how you do this
not sure, i use explicit join types
having a class AB to represent the join table between A and B
the difference is that if you explicitly create a type and DbSet for it then you can query the join table directly and also add more columns on to it if you need to
with the pseudocode you postednot right now, advent of code day 17 is about to drop lol
but i'll get back to you
I feel really stupid about this. How did you do explicit type creation or more columns in the the pseudocode you posted here
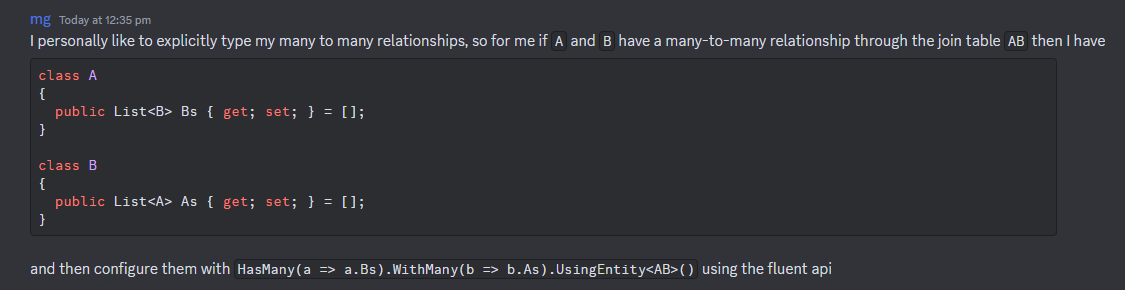
@surwren see the section Many to Many with Class for Join Entity. May answer you current question.
https://learn.microsoft.com/en-us/ef/core/modeling/relationships/many-to-many?source=recommendations
Many-to-many relationships - EF Core
How to configure many-to-many relationships between entity types when using Entity Framework Core
what i didn't include (but probably should have, sorry) was the definition of
AB