Recommended flow for writing + deploying a basic Microservice Application (with DB)?
As per title, I am working on a basic API reliant service that will occasionally be called to store image metadata. I already have a Cloud Object Storage (COS), so I pretty much need a simple 2NF service to CRUD metadata locations for in-app image uploads.
I have looked up a few resources:
https://www.sentinelstand.com/article/guide-to-firebase-storage-download-urls-tokens
https://www.youtube.com/watch?v=mVmIJ4hvTss
I will probably use the attached architecture:
- "backend server" will be this service
- I'm not using AWS S3 so just substitute that with "generic COS"
- Metadata DB will just be any SQL/Relational DB
I am currently considering Tencent Cloud and Azure. Reasons:
- I currently have a free piggyback to use Tencent Cloud; they have a Cloud Virtual Machine.
- I know that Azure interfaces the best with dotNET, but the target for this project is individuals in China and I am unsure of accessibility in that region.
Can anyone advise?
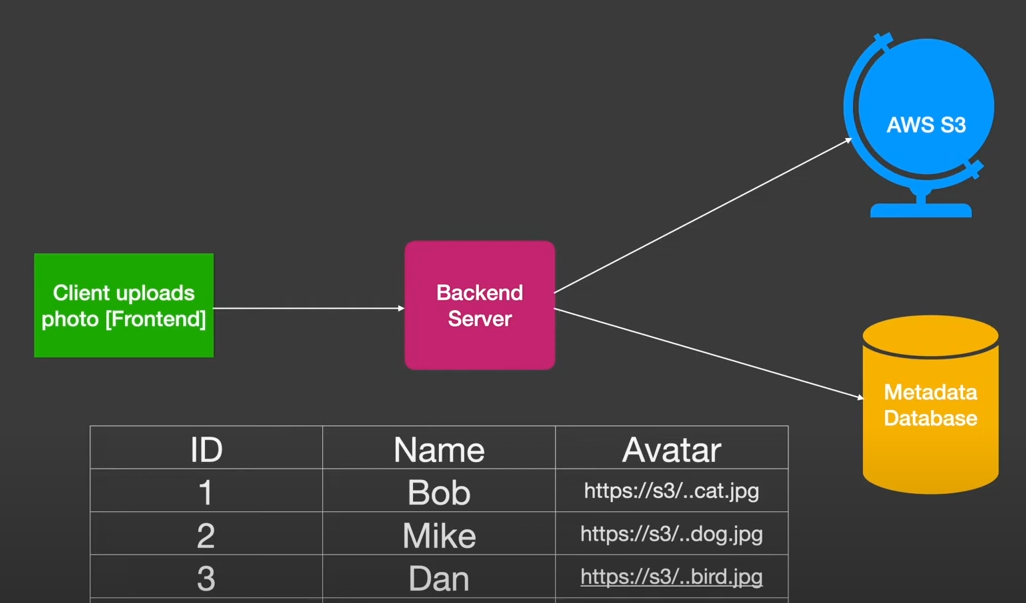
10 Replies
Not sure exactly what you are asking for here. Your suggested "architecture" seems fine
I've never deployed a C# api before, much less a dockerized one, so I have no idea what to expect/what route to take
On the Deployment side:
I checked and apparently C# builds to .dll (when I first deployed Java monolith, it was a dockerized .jar) so I guess I would need to put a .dll in Tencent Cloud Virtual Machine?
I am worried about whether Tencent Cloud Virtual Machine supports .NET or if there are hiccups along the way? I know integration for .NET-Azure is much stronger, but I'm not sure if Azure has feasible connectivity in China
I also don't know if MSSQL is well supported by Tencent or if there might be hiccups on that front
On the C# Side
I don't know what's the proper way to do APIs in C#? I know the only ORM to use is EFCore, but I'm not sure what libraries I should be using for frequent API calls
well if you are deploying to the cloud, most cloud providers have super straight forward ways to run a prebuilt container image
I checked and apparently C# builds to .dll (when I first deployed Java monolith, it was a dockerized .jar) so I guess I would need to put a .dll in Tencent Cloud Virtual Machine?No, you would dockerize the entire thing. The fact that it builds to a dll is an implementation detail - your docker base image should be the ASP runtime one that includes the .NET and ASPnet stuff required to run that dll as an application
I am worried about whether Tencent Cloud Virtual Machine supports .NET or if there are hiccups along the way? I know integration for .NET-Azure is much stronger, but I'm not sure if Azure has feasible connectivity in ChinaIrrelevant as long as they support containers. If tehy dont support containers at all, then yeah they would need .NET support.
I also don't know if MSSQL is well supported by Tencent or if there might be hiccups on that frontI would recommend using postgres regardless, but yeah, this might be a problem.
I don't know what's the proper way to do APIs in C#? I know the only ORM to use is EFCore, but I'm not sure what libraries I should be using for frequent API callswhat do you mean? are you MAKING the calls, or responding to the calls? since you are talking about making an API, Im gonna assume responding for now... in that case, ASP itself is pretty fast and performant, so as long as you follow best practices like using async IO everywhere (including for the image COS) you'll be pretty fine. If you need cutting edge performance, prefer a minimal API over controllers.
Both responding to and making calls
There is a login/auth service written in Java, this service just reuses the existing IDs to record user updates
If I use ASP 9.0, which library import do I use? I last worked with webflux in spring, I don't know what the modern equivalent in C# is
What does webflux do?
You don't really want any extra frameworks on top of ASP.NET, if you're going with minimal API though, you'd want a library to ease the process a bit.
I'm not really an expert but I was taught that WebFlux is asynchronous and non-blocking compared to traditional APIs? So you can load data on the fly as it arrives by essentially using promises, IIRC
I'm moreso wanting to know about the gold standard for web API libs in C# since webflux is apparently the gold standard for Java based on what I was taught
ASP.net has that built in
and I'd say thats true for 99% of things
idk if its "gold standard", but most asp.net projects use EF Core for database these days. Thats about it.
can you suggest some basic way to write controllers/endpoints in a C# environment?
Even in spring boot there are many ways with different annotations, some with Webflux, some without, and honestly it is quite confusing to deal with Jakarta vs Javax imports.
For dotNET I am currently referring to Nedal-Esrar's example (https://github.com/Nedal-Esrar/Travel-and-Accommodation-Booking-Platform/blob/main/src/TABP.Api/Controllers/AuthController.cs) but again he has many features and annotations, whereas some other examples do not have as many annotations.
I do not know enough to know how or what to ask about which annotations or imports are absolutely necessary.
I would really appreciate some pointers one what the bare minimum annotations/imports I would need for a simple API service would be (no auth, simply accepts Get/Post/Put/Delete, calls another service for auth, and then writes to DB or pulls from DB)
ok lets start with controllers
is the bare minimum
for an action (thats what an endpoint in a controller is called), you just need a method decorated with
[HttpGet]
(or Post, Delete, Put... you get the point). These methods should be async and the return value will be json serialized and be the http response
this is enough to get started making endpoints. The other attributes like ApiVersion
and ProducesResponseType
optional but useful if you need to support api versioning, or want to customize the generated swagger
There are some attributes like [FromQuery]
and [FromBody]
etc that are used to tell the modelbinder exactly where to get what value from. useful if you are some complicated endpoints that take both querystring params, payloads, special headers... etcThank you, I will try this