Not triggering on entitlement create
Does anyone know why the code here wouldn't work to create a user and make a subscription on entitlement create?
19 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPDoes the event not emit?
No
IDK how to get someone to test it for me without them having to pay for it
You can create a test entitlement
:method: EntitlementManager#createTest()
[email protected]
Creates a test entitlement
:property: ClientApplication#entitlements [email protected]
The entitlement manager for this applicationmy bot has no commands yet, thats for a interaction endpoint on https api
I can trigger it from Insomnia tho
? You don't need to have a command for creating entitlements. Test entitlement will be free for developers of the app so you can consume it without having to pay for it
Yes but that is an emit for a comand is it not?
I'm not sure what you are talking about
Are you saying the event doesn't emit because you have no way to test it or the event doesn't emit at all when an entitlement is created?
I created on using the payment flow from discord, as it is in my team, and nothing was handled by the app
Do you by any chance have enabled webhook events for Entitlement Create?
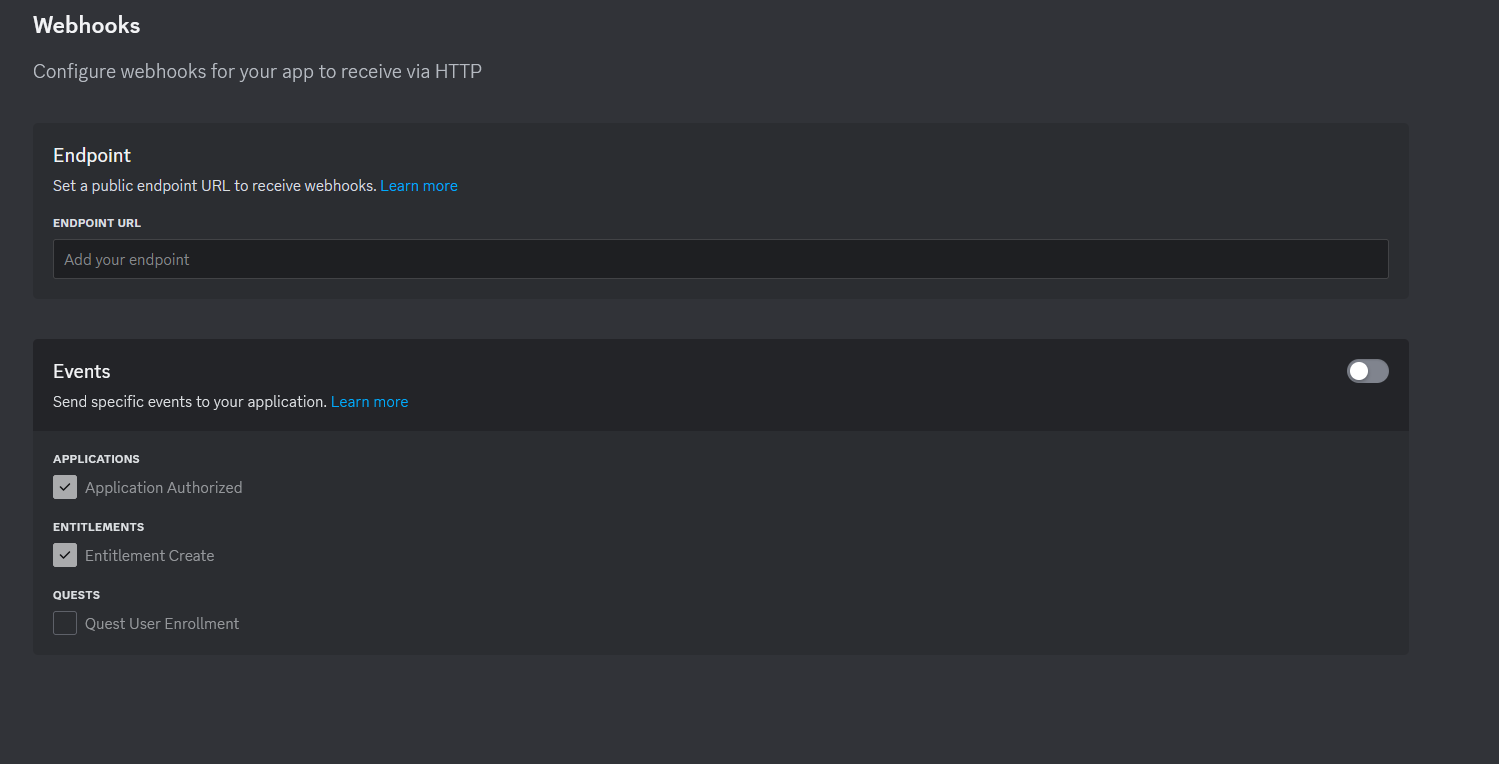
Its off but yeah
I'm not sure if that affects it.. you can try disabling it specifically and then see
What do I need to do to be able to send an entitlement create to my app? Authorization header?
I've cancelled the entitlement in the settings and it has not sent an upate or delete to the bot events.
Huh? Again I have no idea what you are talking about. Maybe you should explain what you are doing and what you expect to happen?
^ followup to this
When a user creates or cancels an app subscription, it should send the EntitlementCreate, EntitlementUpdate or EntitlementDeleted event to the bot. The bot then finds the corresponding user and subscription, creates if not there on create, and deletes if its deleted. and updates if its been updated.
Yeah I get that, but what makes you think it doesn't emit? How are you testing it? Or even, have you made sure that your event handler is properly configured? Is it just this event not emitting or all?
I found out its how I was handling the DB. I was using a DB connection that runs on the edge in vercel, needed to modify for non-edge style hosting. And there is a weird issue with the one that is being run on my account that I tested. It doesnt emit when i activate or cancel it.
If you want to test it I can add you to the team for now?