Upscaling and rotating texture
I've got a method for upscaling and rotating a texture and it essentially works already except that I'm trying to make it more efficient by making it so it only loops over the parts of the result that could potentially have pixels. (skip over known empty parts)
In my visualization here, purple is startX and orange is endX. If ths problem was solved, purple would follow the bounding box's left edge and orange would follow the bounding box's right edge.
This is really a geometry problem, not even C# specific. But every time I try to do it, I screw it up so that it cuts off part of the scaled and rotated result texture and Claude the LLM fails at this really hard.
Minimum reproduction project is here: https://github.com/BenMcLean/UpscaleAndRotate
GitHub
GitHub - BenMcLean/UpscaleAndRotate
Contribute to BenMcLean/UpscaleAndRotate development by creating an account on GitHub.
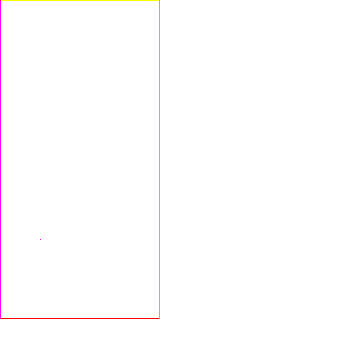
17 Replies
Rather than just fixing the code, I'd appreciate if anyone could even just describe the correct approach to solving this.
I mean, I can write code but I suck at math
i'm curious, do you have a small sample app where i can run this code to view the result?
i can't promise i'll be able to help, but i'm interested in the technique nonetheless
I guess I need to make that.
The whole thing's open source but it's part of a huge library
Are these correct when you draw them out?
Then its just a case of the
min
of that no, for the startX.i'm trying to get the correct startX for each scanline, which is only going to be the minimum corner on the one scanline that happens to intersect the corner.
also one of the corners is always going to be on x=0 as per the method description
i had started to get the corners in order to try to figure out how to get the intercepts of the lines between the corners which would be the real startX and endX
but then again i have never actually drawn those out so i probably should
Ahh per scan line
yeah. i think this should save a lot of CPU and RAM actually, especially on large images and even more when I need to run the same algorithm on Z-slices of a voxel model to make sprite stacks
Different shape but same idea i think?
I created a minimum reproduction project.
The visualization is created by running the only unit test.
https://github.com/BenMcLean/UpscaleAndRotate
GitHub
GitHub - BenMcLean/UpscaleAndRotate
Contribute to BenMcLean/UpscaleAndRotate development by creating an account on GitHub.
and the challenge is to implement the only TODO
I got my unit test lookin pretty slick.
you run the unit test and it writes the animation to "rotated.gif' inside the test project's bin folder ... or wherever else you decide to send it to on your computer by modifying that string.
you just open the gif to see the result
My
Parallelize
is like Select
except it'll run multi-threaded if the runtime allows multi-threading while acting just like regular Select
if it doesn't allow multi-threading.
Yeah and that is a really good article so thanks. I'm bookmarking that because I had planned to implement Bresenham's lines, triangles, circles and ellipses at some point only I haven't got around to it yet.
I don't actually NEED all of those per se because the point of my library is to render voxels but they'd be nice to have.
anyway the problem of the moment is getting the purple and orange lines representing startX and endX to the correct positions on each scanlineI tried feeding Claude the minimum reproduction project and asked it to implement the TODO and the result has your typical LLM glitchiness.
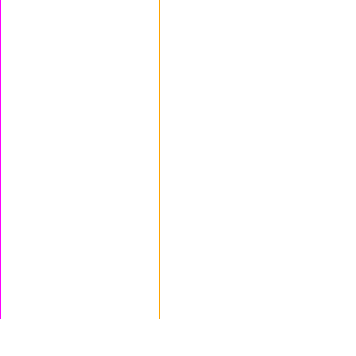
I built a test that could export five images for Claude to examine and I think it managed to solve it after just two iterations.
I'm amazed.
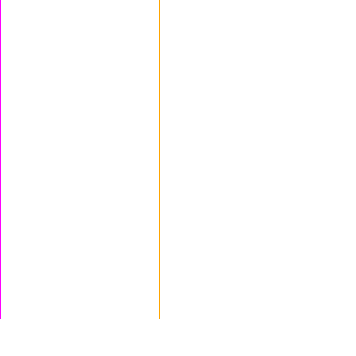
wow check out my sprite stack
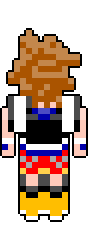