How To Type A Clone Function?
I'm working on my very first Svelte app, and, because I can't use JSX, I'm currently trying to get Wunderbaum working to do a tree grid. (It would be really nice if it used the actual CSS grid layout to display itself rather than fixed width columns in pixels, but it don't, and it doesn't bother me enough to write an alternative. ๐ธ)
Anywho, my program is getting a
FileSystemDirectoryHandle
from window.showDirectoryPicker()
, and recursively spidering that directory into a Wunderbaum tree for review before generating an IPFS CAR archive from whatever files the user selects.
The problem is Wunderbaum mutates the input data structure in such a way I can't use it to generate the CAR file. So, one approach I was considering was to copy the input before sending it to Wunderbaum. The problem is it contains FileSystemFileHandle
s for all the files in the tree, & those can't be cloned, so I came up with:
Which I would call as cloneWithout(root, ['handle'])
to omit the handle
property from being cloned.
As is so often the case, TypeScript is unhappy with my code. It doesn't seem to like to return unknown
, nor does it appreciate that the object I'm passing in is unknown
.
Should I be using any
s? I try to avoid them because ESLint bitches so much. ๐ธ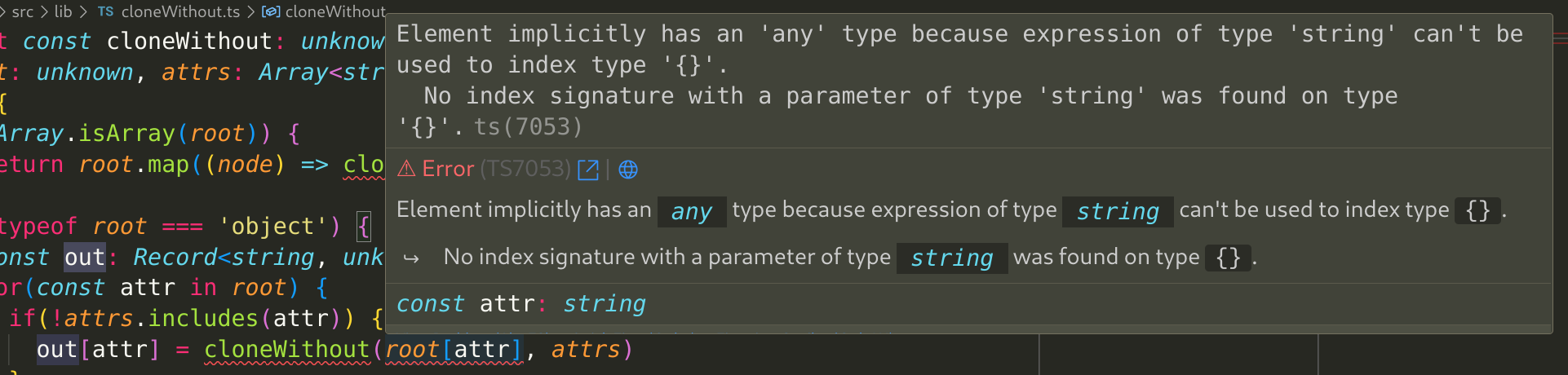
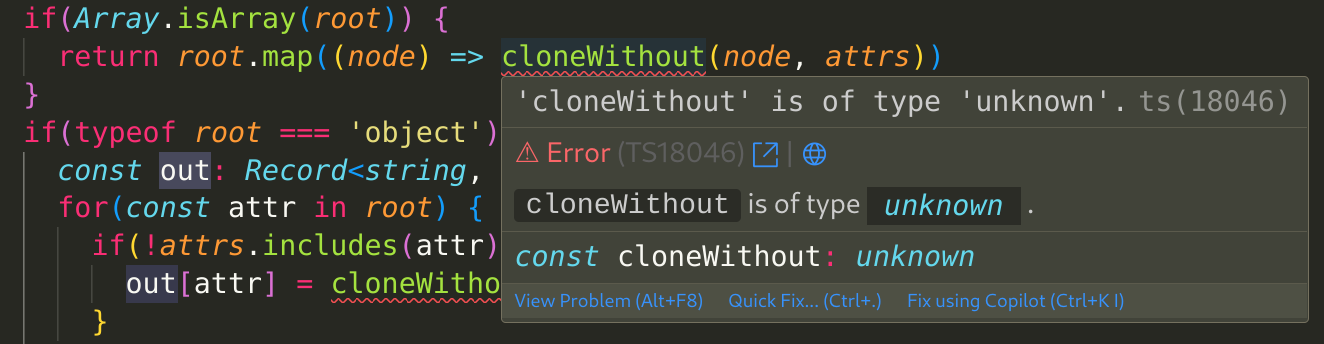
1 Reply
well, this horrible thing seems to make the compiler happy: