✅ Why ins't this EF Core query being evaluated client-side?
Currently, I have this query:
In the query above, in the
Database.KanbanCards
sub-query I make the rest of the query client-side to transform the "a.AssociatedUsers" column into other information. This is an JSON column.20 Replies
Btw i'm getting the following exception, telling my expression ins't being evaluated on client side.
what am I doing wrong?
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
@cypherpotato only the last Select can be client side
Oh wait, you added AsE
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
Oh
Yeah that AsE needs to be in the “root” query chain.
I thought I could use AsEnumerable in nested queries. If I use it at the root, will the nested queries also be executed on the client side?
yea by the end i've rewrited the entire query to use IEnumerable instead
this was the final query
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
nope. my foreign keys are set in OnModelCreating. I don't use navigation in models
what
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
hahaa you're right
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
the JSON is configured to sent everything as camelCase btw
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
but I've always seen anonymous members closer to fields than properties
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
I am. right there
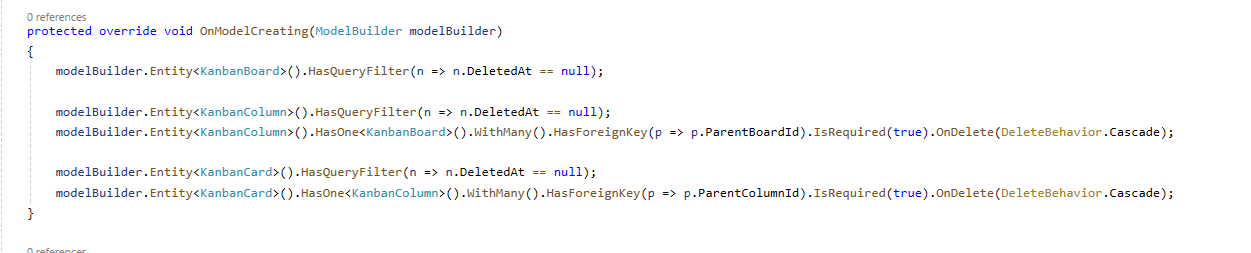
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
what no
Unknown User•2mo ago
Message Not Public
Sign In & Join Server To View
but yea, thank you guys
also i'll follow the tip of naming of anonymous objects