Slash Command Not working
PING COMMAND
Interaction Create
Event Handler
module.exports.run = async (interaction) => {
const pingEmbed = new EmbedBuilder()
.setAuthor({ name:`RebootMC`, iconURL: interaction.client.user.displayAvatarURL()})
.setColor(`#fcd200`)
.setFooter({ text: `RebootMC. All rights reserved.`, iconURL: interaction.client.user.displayAvatarURL()})
.setTimestamp();
return interaction.deferReply({ embeds: [pingEmbed], ephemeral: true });
}
module.exports.help = {
name: 'ping',
desc: 'Outputs the average of the last 5 websocket pings sent to the Discord API.',
usage: 'ping',
category: 'general'
};
module.exports.run = async (interaction) => {
const pingEmbed = new EmbedBuilder()
.setAuthor({ name:`RebootMC`, iconURL: interaction.client.user.displayAvatarURL()})
.setColor(`#fcd200`)
.setFooter({ text: `RebootMC. All rights reserved.`, iconURL: interaction.client.user.displayAvatarURL()})
.setTimestamp();
return interaction.deferReply({ embeds: [pingEmbed], ephemeral: true });
}
module.exports.help = {
name: 'ping',
desc: 'Outputs the average of the last 5 websocket pings sent to the Discord API.',
usage: 'ping',
category: 'general'
};
client.on(Events.InteractionCreate, async interaction => {
if (!interaction.isChatInputCommand()) return;
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`No command matching ${interaction.commandName} was found.`);
return;
}
try {
await command.run(interaction);
} catch (error) {
console.error(error);
if (interaction.replied || interaction.deferred) {
await interaction.followUp({ content: 'There was an error while executing this command!', ephemeral: true });
} else {
await interaction.reply({ content: 'There was an error while executing this command!', ephemeral: true });
}
}
});
client.on(Events.InteractionCreate, async interaction => {
if (!interaction.isChatInputCommand()) return;
const command = interaction.client.commands.get(interaction.commandName);
if (!command) {
console.error(`No command matching ${interaction.commandName} was found.`);
return;
}
try {
await command.run(interaction);
} catch (error) {
console.error(error);
if (interaction.replied || interaction.deferred) {
await interaction.followUp({ content: 'There was an error while executing this command!', ephemeral: true });
} else {
await interaction.reply({ content: 'There was an error while executing this command!', ephemeral: true });
}
}
});
readdir("./events/", (err, files) => {
if (err) return console.error(err);
// Filter for JavaScript files and count them
let evnFiles = files.filter(f => f.split(".").pop() === 'js');
const totalFiles = evnFiles.length; // Number of .js files
console.log(`\nLoading ${totalFiles} Event Files Now...`);
if (totalFiles === 0) return console.log('\x1b[33m ⚠ - No event files found!\x1b[0m');
// Load each event file
evnFiles.forEach((f, i) => {
const props = require(`../events/${f}`);
let eventName = f.split(".")[0];
console.log(`${i + 1}: ${f} loaded!`);
client.on(eventName, props.bind(null, client));
});
});
readdir("./events/", (err, files) => {
if (err) return console.error(err);
// Filter for JavaScript files and count them
let evnFiles = files.filter(f => f.split(".").pop() === 'js');
const totalFiles = evnFiles.length; // Number of .js files
console.log(`\nLoading ${totalFiles} Event Files Now...`);
if (totalFiles === 0) return console.log('\x1b[33m ⚠ - No event files found!\x1b[0m');
// Load each event file
evnFiles.forEach((f, i) => {
const props = require(`../events/${f}`);
let eventName = f.split(".")[0];
console.log(`${i + 1}: ${f} loaded!`);
client.on(eventName, props.bind(null, client));
});
});
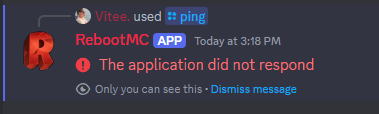
6 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OP[email protected] & Node v18.16.0
What do you mean?
The handlers handle the listeners like ready, guildaddmember
yeah, so why are you listening to
interactionCreate
directly instead of using your handler
anyway...
- Show your command handler
- verify client.commands
contain the said command (do you get the No matching command found... error on console?)
- verify interactionCreate is being fired at all?const { Collection } = require("discord.js");
const { readdirSync, readdir} = require("fs")
module.exports = (client) => {
client.commands = new Collection();
readdirSync('./commands').forEach(dir => {
readdir(`./commands/${dir}`, (err, files) => {
if (err) return console.log(err);
// Filter for JavaScript files and count them
let cmdFiles = files.filter(f => f.split(".").pop() === "js");
const totalFiles = cmdFiles.length; // Number of .js files
console.log(`\nLoading ${totalFiles} Slash Commands...`);
if (cmdFiles.length === 0) return console.log("\x1b[33m ⚠ - No event files found!\x1b[0m");
// Load each slash command file
cmdFiles.forEach((f, i) => {
let props = require(`../commands/${dir}/${f}`);
console.log(`${i + 1}: ${f} loaded`)
client.commands.set(props.help.name, props);
if (props.help.aliases) props.help.aliases.forEach(a => client.aliases.set(a, props.help.name));
})
})
})
};
const { Collection } = require("discord.js");
const { readdirSync, readdir} = require("fs")
module.exports = (client) => {
client.commands = new Collection();
readdirSync('./commands').forEach(dir => {
readdir(`./commands/${dir}`, (err, files) => {
if (err) return console.log(err);
// Filter for JavaScript files and count them
let cmdFiles = files.filter(f => f.split(".").pop() === "js");
const totalFiles = cmdFiles.length; // Number of .js files
console.log(`\nLoading ${totalFiles} Slash Commands...`);
if (cmdFiles.length === 0) return console.log("\x1b[33m ⚠ - No event files found!\x1b[0m");
// Load each slash command file
cmdFiles.forEach((f, i) => {
let props = require(`../commands/${dir}/${f}`);
console.log(`${i + 1}: ${f} loaded`)
client.commands.set(props.help.name, props);
if (props.help.aliases) props.help.aliases.forEach(a => client.aliases.set(a, props.help.name));
})
})
})
};
Collection(1) [Map] {
'ping' => {
run: [AsyncFunction (anonymous)],
help: {
name: 'ping',
desc: 'Outputs the average of the last 5 websocket pings sent to the Discord API.',
usage: 'ping',
category: 'general'
}
}
}
Collection(1) [Map] {
'ping' => {
run: [AsyncFunction (anonymous)],
help: {
name: 'ping',
desc: 'Outputs the average of the last 5 websocket pings sent to the Discord API.',
usage: 'ping',
category: 'general'
}
}
}
ChatInputCommandInteraction {
type: 2,
id: '1307683438828195883',
applicationId: '1307551436233769010',
channelId: '1223239487967072266',
guildId: '664302680231116834',
user: User {
id: '1118905277232713828',
bot: false,
system: false,
flags: UserFlagsBitField { bitfield: 64 },
username: 'vitee.',
globalName: 'Vitee.',
discriminator: '0',
avatar: 'a_d753db6f8cb00ba222b857d7ba51f660',
banner: undefined,
accentColor: undefined,
avatarDecoration: null,
avatarDecorationData: null
},
member: GuildMember {
guild: Guild {
id: '664302680231116834',
name: 'KyvastMC',
icon: '20c84dc8c0dd9ecf8cfb8a1b7d5e7b77',
features: [Array],
commands: [GuildApplicationCommandManager],
members: [GuildMemberManager],
channels: [GuildChannelManager],
bans: [GuildBanManager],
roles: [RoleManager],
presences: PresenceManager {},
voiceStates: [VoiceStateManager],
stageInstances: [StageInstanceManager],
invites: [GuildInviteManager],
scheduledEvents: [GuildScheduledEventManager],
autoModerationRules: [AutoModerationRuleManager],
available: true,
shardId: 0,
splash: null,
banner: null,
description: null,
verificationLevel: 2,
vanityURLCode: null,
nsfwLevel: 0,
premiumSubscriptionCount: 0,
discoverySplash: null,
memberCount: 8,
large: false,
premiumProgressBarEnabled: true,
applicationId: null,
afkTimeout: 300,
afkChannelId: null,
systemChannelId: null,
premiumTier: 0,
widgetEnabled: null,
widgetChannelId: null,
explicitContentFilter: 2,
mfaLevel: 0,
joinedTimestamp: 1731818691129,
defaultMessageNotifications: 1,
systemChannelFlags: [SystemChannelFlagsBitField],
maximumMembers: 500000,
maximumPresences: null,
maxVideoChannelUsers: 25,
maxStageVideoChannelUsers: 50,
approximateMemberCount: null,
approximatePresenceCount: null,
vanityURLUses: null,
rulesChannelId: '1223213309046689913',
publicUpdatesChannelId: '1223213309046689913',
preferredLocale: 'en-US',
safetyAlertsChannelId: null,
ownerId: '1118905277232713828',
emojis: [GuildEmojiManager],
stickers: [GuildStickerManager]
},
joinedTimestamp: 1711370627789,
premiumSinceTimestamp: null,
nickname: null,
pending: false,
communicationDisabledUntilTimestamp: null,
user: User {
id: '1118905277232713828',
bot: false,
system: false,
flags: [UserFlagsBitField],
username: 'vitee.',
globalName: 'Vitee.',
discriminator: '0',
avatar: 'a_d753db6f8cb00ba222b857d7ba51f660',
banner: undefined,
accentColor: undefined,
avatarDecoration: null,
avatarDecorationData: null
},
avatar: null,
flags: GuildMemberFlagsBitField { bitfield: 0 }
},
version: 1,
appPermissions: PermissionsBitField { bitfield: 2251799813685247n },
memberPermissions: PermissionsBitField { bitfield: 2251799813685247n },
locale: 'en-US',
guildLocale: 'en-US',
ChatInputCommandInteraction {
type: 2,
id: '1307683438828195883',
applicationId: '1307551436233769010',
channelId: '1223239487967072266',
guildId: '664302680231116834',
user: User {
id: '1118905277232713828',
bot: false,
system: false,
flags: UserFlagsBitField { bitfield: 64 },
username: 'vitee.',
globalName: 'Vitee.',
discriminator: '0',
avatar: 'a_d753db6f8cb00ba222b857d7ba51f660',
banner: undefined,
accentColor: undefined,
avatarDecoration: null,
avatarDecorationData: null
},
member: GuildMember {
guild: Guild {
id: '664302680231116834',
name: 'KyvastMC',
icon: '20c84dc8c0dd9ecf8cfb8a1b7d5e7b77',
features: [Array],
commands: [GuildApplicationCommandManager],
members: [GuildMemberManager],
channels: [GuildChannelManager],
bans: [GuildBanManager],
roles: [RoleManager],
presences: PresenceManager {},
voiceStates: [VoiceStateManager],
stageInstances: [StageInstanceManager],
invites: [GuildInviteManager],
scheduledEvents: [GuildScheduledEventManager],
autoModerationRules: [AutoModerationRuleManager],
available: true,
shardId: 0,
splash: null,
banner: null,
description: null,
verificationLevel: 2,
vanityURLCode: null,
nsfwLevel: 0,
premiumSubscriptionCount: 0,
discoverySplash: null,
memberCount: 8,
large: false,
premiumProgressBarEnabled: true,
applicationId: null,
afkTimeout: 300,
afkChannelId: null,
systemChannelId: null,
premiumTier: 0,
widgetEnabled: null,
widgetChannelId: null,
explicitContentFilter: 2,
mfaLevel: 0,
joinedTimestamp: 1731818691129,
defaultMessageNotifications: 1,
systemChannelFlags: [SystemChannelFlagsBitField],
maximumMembers: 500000,
maximumPresences: null,
maxVideoChannelUsers: 25,
maxStageVideoChannelUsers: 50,
approximateMemberCount: null,
approximatePresenceCount: null,
vanityURLUses: null,
rulesChannelId: '1223213309046689913',
publicUpdatesChannelId: '1223213309046689913',
preferredLocale: 'en-US',
safetyAlertsChannelId: null,
ownerId: '1118905277232713828',
emojis: [GuildEmojiManager],
stickers: [GuildStickerManager]
},
joinedTimestamp: 1711370627789,
premiumSinceTimestamp: null,
nickname: null,
pending: false,
communicationDisabledUntilTimestamp: null,
user: User {
id: '1118905277232713828',
bot: false,
system: false,
flags: [UserFlagsBitField],
username: 'vitee.',
globalName: 'Vitee.',
discriminator: '0',
avatar: 'a_d753db6f8cb00ba222b857d7ba51f660',
banner: undefined,
accentColor: undefined,
avatarDecoration: null,
avatarDecorationData: null
},
avatar: null,
flags: GuildMemberFlagsBitField { bitfield: 0 }
},
version: 1,
appPermissions: PermissionsBitField { bitfield: 2251799813685247n },
memberPermissions: PermissionsBitField { bitfield: 2251799813685247n },
locale: 'en-US',
guildLocale: 'en-US',
DiscordAPIError[10062]: Unknown interaction
at handleErrors (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:727:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:831:23)
at async _REST.request (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:1272:22)
at async ChatInputCommandInteraction.reply (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:115:5)
at async Client.<anonymous> (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\events\interactionCreate.js:16:13) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: { message: 'Unknown interaction', code: 10062 },
code: 10062,
status: 404,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1307686566281347124/aW50ZXJhY3Rpb246MTMwNzY4NjU2NjI4MTM0NzEyNDpKVUw3cWp3YUZEc3VuajZpVVVmUmlvQXFPcmxIeWxVenBMSHpIa2hqMnVkTFhGQVpkRW5WdlBlV1hOeDR2VWlQQkNYZkhaaDl6dmVVNmd0bzhoM2hXMW5LeUQ0S09sRVVzVmozWVRzdG1aZnpKNXplR3hJNGZ2TXFNZXRnUmRHUA/callback'
}
node:events:491
throw er; // Unhandled 'error' event
^
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:727:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:831:23)
at async _REST.request (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:1272:22)
at async ChatInputCommandInteraction.reply (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:115:5)
at async Client.<anonymous> (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\events\interactionCreate.js:22:17)
Emitted 'error' event on Client instance at:
at emitUnhandledRejectionOrErr (node:events:394:10)
at process.processTicksAndRejections (node:internal/process/task_queues:84:21) {
requestBody: {
files: [],
json: {
type: 4,
data: {
content: 'There was an error while executing this command!',
tts: false,
nonce: undefined,
enforce_nonce: false,
embeds: undefined,
components: undefined,
username: undefined,
avatar_url: undefined,
allowed_mentions: undefined,
flags: 64,
message_reference: undefined,
attachments: undefined,
sticker_ids: undefined,
thread_name: undefined,
applied_tags: undefined,
poll: undefined
}
}
},
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1307686566281347124/aW50ZXJhY3Rpb246MTMwNzY4NjU2NjI4MTM0NzEyNDpKVUw3cWp3YUZEc3VuajZpVVVmUmlvQXFPcmxIeWxVenBMSHpIa2hqMnVkTFhGQVpkRW5WdlBlV1hOeDR2VWlQQkNYZkhaaDl6dmVVNmd0bzhoM2hXMW5LeUQ0S09sRVVzVmozWVRzdG1aZnpKNXplR3hJNGZ2TXFNZXRnUmRHUA/callback'
}
DiscordAPIError[10062]: Unknown interaction
at handleErrors (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:727:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:831:23)
at async _REST.request (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:1272:22)
at async ChatInputCommandInteraction.reply (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:115:5)
at async Client.<anonymous> (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\events\interactionCreate.js:16:13) {
requestBody: { files: [], json: { type: 4, data: [Object] } },
rawError: { message: 'Unknown interaction', code: 10062 },
code: 10062,
status: 404,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1307686566281347124/aW50ZXJhY3Rpb246MTMwNzY4NjU2NjI4MTM0NzEyNDpKVUw3cWp3YUZEc3VuajZpVVVmUmlvQXFPcmxIeWxVenBMSHpIa2hqMnVkTFhGQVpkRW5WdlBlV1hOeDR2VWlQQkNYZkhaaDl6dmVVNmd0bzhoM2hXMW5LeUQ0S09sRVVzVmozWVRzdG1aZnpKNXplR3hJNGZ2TXFNZXRnUmRHUA/callback'
}
node:events:491
throw er; // Unhandled 'error' event
^
DiscordAPIError[40060]: Interaction has already been acknowledged.
at handleErrors (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:727:13)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
at async BurstHandler.runRequest (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:831:23)
at async _REST.request (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\@discordjs\rest\dist\index.js:1272:22)
at async ChatInputCommandInteraction.reply (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\node_modules\discord.js\src\structures\interfaces\InteractionResponses.js:115:5)
at async Client.<anonymous> (C:\Users\DrSpa\OneDrive\Desktop\RebootMC Bot\events\interactionCreate.js:22:17)
Emitted 'error' event on Client instance at:
at emitUnhandledRejectionOrErr (node:events:394:10)
at process.processTicksAndRejections (node:internal/process/task_queues:84:21) {
requestBody: {
files: [],
json: {
type: 4,
data: {
content: 'There was an error while executing this command!',
tts: false,
nonce: undefined,
enforce_nonce: false,
embeds: undefined,
components: undefined,
username: undefined,
avatar_url: undefined,
allowed_mentions: undefined,
flags: 64,
message_reference: undefined,
attachments: undefined,
sticker_ids: undefined,
thread_name: undefined,
applied_tags: undefined,
poll: undefined
}
}
},
rawError: {
message: 'Interaction has already been acknowledged.',
code: 40060
},
code: 40060,
status: 400,
method: 'POST',
url: 'https://discord.com/api/v10/interactions/1307686566281347124/aW50ZXJhY3Rpb246MTMwNzY4NjU2NjI4MTM0NzEyNDpKVUw3cWp3YUZEc3VuajZpVVVmUmlvQXFPcmxIeWxVenBMSHpIa2hqMnVkTFhGQVpkRW5WdlBlV1hOeDR2VWlQQkNYZkhaaDl6dmVVNmd0bzhoM2hXMW5LeUQ0S09sRVVzVmozWVRzdG1aZnpKNXplR3hJNGZ2TXFNZXRnUmRHUA/callback'
}
but allways the first reaction i get this
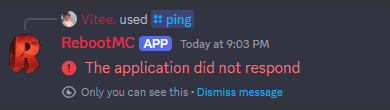
then it works
so maybe the erorr later is from this
So put the interactionCreate in the command handler?
sorry event handler
why
thx man. that really fixed the problem. really appreciate the help