Removing roll from camera matrix
I have a camera class which is just this:
and it works, but I want to eliminate the roll from the matrix somehow.
14 Replies
ā
This post has been reserved for your question.
Hey @špothicon! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
(roll as in the tilting of the camera from left to right as shown here)
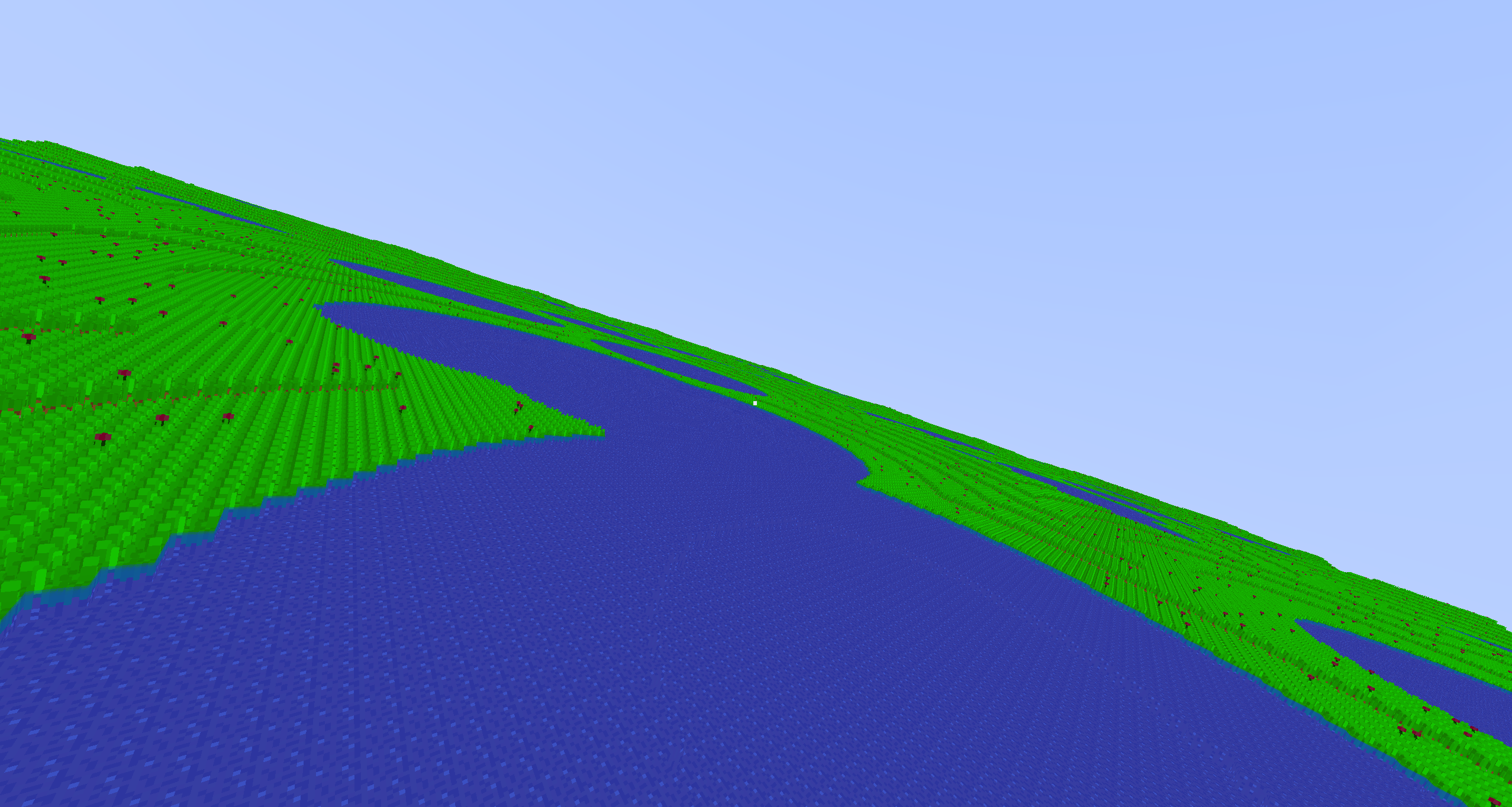
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
.
/reopen
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
open seasame
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
Then don't rotate it
Does the default constructor of Matrix4f return an identity matrix?
Yes (but not sure why that matters)
but i need to rotate the matrix so that i can well.. rotate the camera around, just need to somehow eliminate the roll aspect immediately afterwards
I don't understand
Just don't rotate on the roll axis
what do you do to not rotate on the roll axis tho
ive tried everything i can think of
its not like their seperate numbers
im getting three axis of rotation just by rotating x and y
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.
.
š¤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.