implement stripe payment feature for asp.net core web api and wpf
so I am trying to add a payment feature using stripe but it takes me to this page for some reason I am not sure how to fix it
here is my code
{
private readonly PaymentService _paymentService;
private readonly ApplicationDbContext _context;
public PaymentController(PaymentService paymentService)
{
_paymentService = paymentService;
}
[HttpPost("create-checkout-session")]
public ActionResult CreateCheckoutSession()
{
var session = _paymentService.CreateCheckoutSession(
"https://localhost:7186/payment/success?sessionId={CHECKOUT_SESSION_ID}",
"https://localhost:7186/payment/cancel?sessionId={CHECKOUT_SESSION_ID}", "20"
);
return Ok(new { sessionId = session.Url });
}
[HttpGet("success")]
public async Task<IActionResult> Success(string sessionId)
{
/*
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Success";
await _context.SaveChangesAsync();
}
*/
// Redirect to a success page or return success response
return Ok("Payment successful.");
}
[HttpGet("cancel")]
public async Task<IActionResult> Cancel(string sessionId)
{
/*
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Cancelled";
await _context.SaveChangesAsync();
}
*/
// Redirect to a cancel page or return cancel response
return Ok("Payment cancelled.");
}
}
{
private readonly PaymentService _paymentService;
private readonly ApplicationDbContext _context;
public PaymentController(PaymentService paymentService)
{
_paymentService = paymentService;
}
[HttpPost("create-checkout-session")]
public ActionResult CreateCheckoutSession()
{
var session = _paymentService.CreateCheckoutSession(
"https://localhost:7186/payment/success?sessionId={CHECKOUT_SESSION_ID}",
"https://localhost:7186/payment/cancel?sessionId={CHECKOUT_SESSION_ID}", "20"
);
return Ok(new { sessionId = session.Url });
}
[HttpGet("success")]
public async Task<IActionResult> Success(string sessionId)
{
/*
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Success";
await _context.SaveChangesAsync();
}
*/
// Redirect to a success page or return success response
return Ok("Payment successful.");
}
[HttpGet("cancel")]
public async Task<IActionResult> Cancel(string sessionId)
{
/*
var paymentRecord = await _context.PaymentRecords.FirstOrDefaultAsync(p => p.StripeSessionId == sessionId);
if (paymentRecord != null)
{
paymentRecord.Status = "Cancelled";
await _context.SaveChangesAsync();
}
*/
// Redirect to a cancel page or return cancel response
return Ok("Payment cancelled.");
}
}
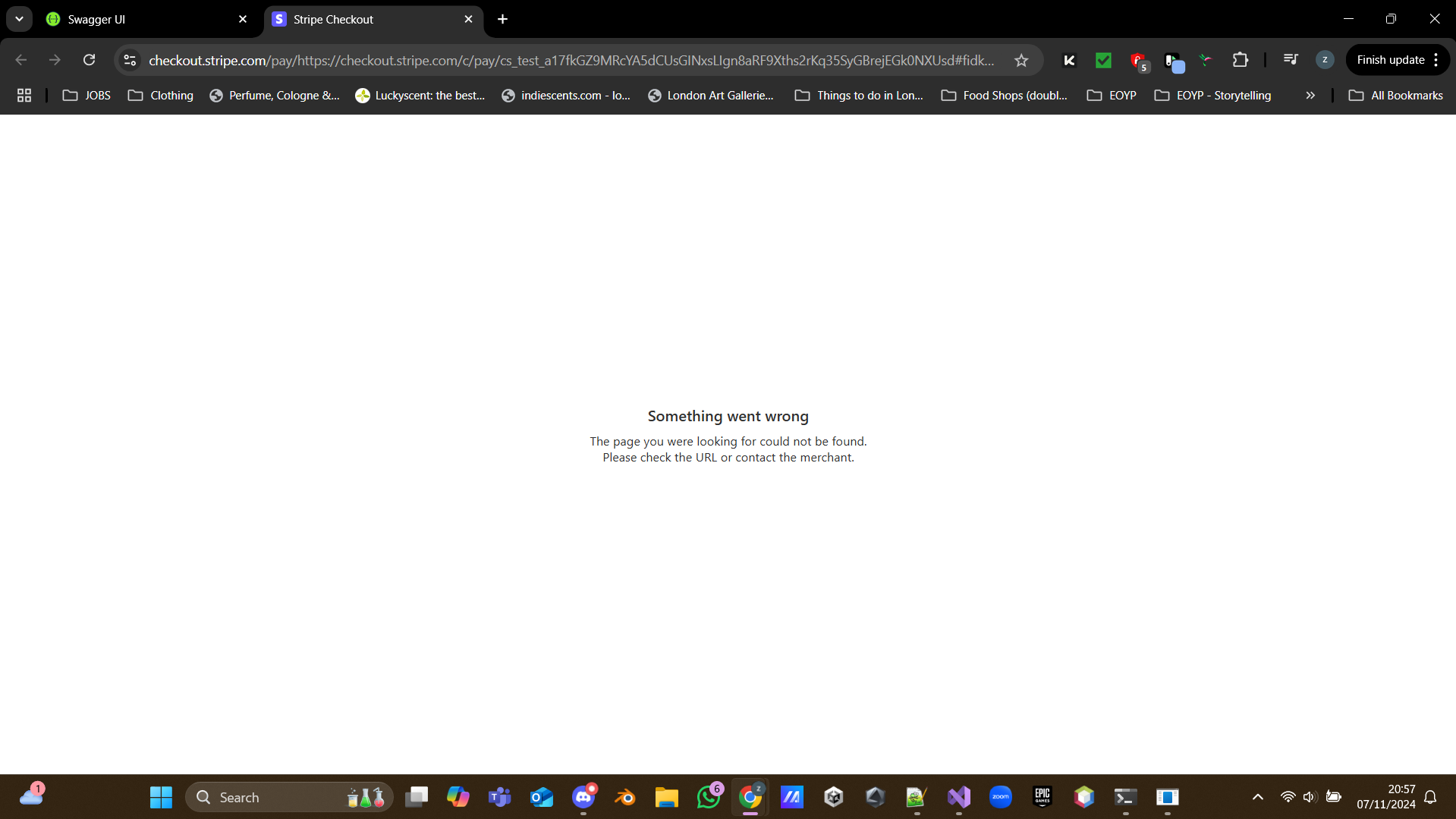
2 Replies
public class PaymentService
{
public PaymentService(IOptions<StripeOptions> stripeSettings)
{
StripeConfiguration.ApiKey = stripeSettings.Value.SecretKey;
}
public Stripe.Checkout.Session CreateCheckoutSession(string successUrl, string cancelUrl, string amount)
{
var options = new Stripe.Checkout.SessionCreateOptions
{
PaymentMethodTypes = new List<string>
{
"card",
},
LineItems = new List<SessionLineItemOptions>
{
new SessionLineItemOptions
{
PriceData = new SessionLineItemPriceDataOptions
{
UnitAmount = Convert.ToInt32(amount) * 100,
Currency = "usd",
ProductData = new SessionLineItemPriceDataProductDataOptions
{
Name = "Product Name",
Description = "Product Description"
},
},
Quantity = 1,
},
},
Mode = "payment",
SuccessUrl = successUrl,
CancelUrl = cancelUrl,
};
var service = new Stripe.Checkout.SessionService();
Stripe.Checkout.Session session = service.Create(options);
return session;
}
}
public class PaymentService
{
public PaymentService(IOptions<StripeOptions> stripeSettings)
{
StripeConfiguration.ApiKey = stripeSettings.Value.SecretKey;
}
public Stripe.Checkout.Session CreateCheckoutSession(string successUrl, string cancelUrl, string amount)
{
var options = new Stripe.Checkout.SessionCreateOptions
{
PaymentMethodTypes = new List<string>
{
"card",
},
LineItems = new List<SessionLineItemOptions>
{
new SessionLineItemOptions
{
PriceData = new SessionLineItemPriceDataOptions
{
UnitAmount = Convert.ToInt32(amount) * 100,
Currency = "usd",
ProductData = new SessionLineItemPriceDataProductDataOptions
{
Name = "Product Name",
Description = "Product Description"
},
},
Quantity = 1,
},
},
Mode = "payment",
SuccessUrl = successUrl,
CancelUrl = cancelUrl,
};
var service = new Stripe.Checkout.SessionService();
Stripe.Checkout.Session session = service.Create(options);
return session;
}
}
public PaymentPage(FlightOfferDTO flightOffer)
{
InitializeComponent();
FlightOffer = flightOffer;
// Bind data to UI
FlightDetailsTextBlock.Text = $"Flight Number: {FlightOffer.FlightNumber}";
TotalPriceTextBlock.Text = $"${FlightOffer.Price}";
}
private async void ProceedToPaymentButton_Click(object sender, RoutedEventArgs e)
{
// Replace with your API's base address and endpoint
string apiUrl = "https://localhost:7186/api/payment/create-checkout-session";
// Use HttpClient to call the API
using (HttpClient client = new HttpClient())
{
try
{
// Make a POST request to the API
HttpResponseMessage response = await client.PostAsync(apiUrl, null);
// Check if the response is successful
if (response.IsSuccessStatusCode)
{
var responseContent = await response.Content.ReadAsStringAsync();
var jsonResponse = JObject.Parse(responseContent);
// Extract the sessionId
string sessionId = jsonResponse["sessionId"].ToString();
// Redirect to Stripe Checkout
string checkoutUrl = $"https://checkout.stripe.com/pay/{sessionId}";
System.Diagnostics.Process.Start(new System.Diagnostics.ProcessStartInfo
{
FileName = checkoutUrl,
UseShellExecute = true
});
}
else
{
MessageBox.Show("Failed to create a checkout session.");
}
}
catch (Exception ex)
{
MessageBox.Show($"An error occurred: {ex.Message}");
}
}
}
public PaymentPage(FlightOfferDTO flightOffer)
{
InitializeComponent();
FlightOffer = flightOffer;
// Bind data to UI
FlightDetailsTextBlock.Text = $"Flight Number: {FlightOffer.FlightNumber}";
TotalPriceTextBlock.Text = $"${FlightOffer.Price}";
}
private async void ProceedToPaymentButton_Click(object sender, RoutedEventArgs e)
{
// Replace with your API's base address and endpoint
string apiUrl = "https://localhost:7186/api/payment/create-checkout-session";
// Use HttpClient to call the API
using (HttpClient client = new HttpClient())
{
try
{
// Make a POST request to the API
HttpResponseMessage response = await client.PostAsync(apiUrl, null);
// Check if the response is successful
if (response.IsSuccessStatusCode)
{
var responseContent = await response.Content.ReadAsStringAsync();
var jsonResponse = JObject.Parse(responseContent);
// Extract the sessionId
string sessionId = jsonResponse["sessionId"].ToString();
// Redirect to Stripe Checkout
string checkoutUrl = $"https://checkout.stripe.com/pay/{sessionId}";
System.Diagnostics.Process.Start(new System.Diagnostics.ProcessStartInfo
{
FileName = checkoutUrl,
UseShellExecute = true
});
}
else
{
MessageBox.Show("Failed to create a checkout session.");
}
}
catch (Exception ex)
{
MessageBox.Show($"An error occurred: {ex.Message}");
}
}
}
You've set the session url to the id. You can just use the url directly instead of trying to add session.payment to the start