Parabola elements calculator
so, im trying to write a program what would calculate the direction of opening, vertex, y intercept, zeros, and vertex form of the equation. how would I go about doing that?
178 Replies
⌛
This post has been reserved for your question.
Hey @Royalrex25! Please useTIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here./close
or theClose Post
button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically marked as dormant after 300 minutes of inactivity.
I have the first bit done already.
this is urgent
What exactly do you want to do?
The first step would be to figure out how to do each of these things mathematically
Then it'd be a relatively simple task of translating that into Java
true lol
Once you've done that, if you get stuck writing some specific piece in Java, I think that'd be a more appropriate question for here
If we told you how to do the math we'd just be doing your homework for you
lol, but how do I get user input for specific variables?
You can use a
Scanner
, BufferedReader
or similar on System.in
thanks, how would I tie System.in to A for example? its been a while so I've forgotten
For example
thanks mate
Alternatively you could pass them as
args
, which is what I'd do given the choiceapperantly the readDouble() symbol cannot be found
nextDouble
hmm, never used that method before
it's if you want to pass input to the application before starting it
there we go, now I just need to do that two more times
I see
ok, so the code can't find symbol A from the double that is defined before that code occurs.
Variables are case sensitive
and it needs to be in scope
if you define a variable inside a
{}
block, you can only use it in that blockused uppercase for both
I defined A wthin the try area
it's better to write all the code inside the try block here tbh
Did you also do the same with the code using a?
Why? I try to keep try blocks as small as possible with only relevant bits
Also by convention, it's recommended to use camelCase (starting with a lowercase letter) for all variables and methods
Because it's
System.in
and you cannot use it once you closed itI did both with A, and now the scanner can't use A
I mean technically closing
System.in
isn't necessary but IMO it's good to get used to itdue to predefinition in main block
Can you show your code?
I'd presume he's reading his three things and being done with stdin
you don't know
here's the updated code so far
I'd move the code after the try block inside the try block
and you were redeclaring the variable i.e. you'd have two variables named
A
that fixed it
now I just need to get B and C defined from user input as well
and then I can do the rest (hopefully)
Do you know how?
use the same method as A?
yes
do I need to close the A method?
or is it fine without that?
?
I mean close the scanner for A
You should use the same scanner for all inputs
and the
try(){}
block automatically closes the scanner at the endcuz I need 3 different values for A B and C to be different
Note that you don't really need to close
System.in
(and Scanner
s on it), that's your choice - but closing is important with some other IO (e.g. files or networking)
if you run nextDouble
3 times, it will read 3 values one after each otherI see..
ahh, so how can I add a text prompt to nextDouble to make sure the use knows what each is for?
System.out.println
I assume you are able to use it?ik how to use system.out.println but I wanted to make sure there wasn't some convaluted method. and im guessing I put one before each double is scanned?
It should be fine with println
yeah, but does each one go before or after the scan.nextdouble?
Do you want to first show the user something or get data from the user?
I want to show them the prompt first so that they know what their inputing/what to do
but I also don't want all three going at once
then execute the corresponding things in that order
Why is this tagged "recursion" and "maven" lol
recursion???
my bad, should be fixed
so, how do I portray -b in java for the vertex?
?
cuz I got -75 from A = 10, B = 25
and the formula for a vertex is -b/2a
thats why im asking
I am not sure what you mean with vertex
im doing a parabola solver
What exactly are you doing and what's the issue with that?
so?
I don't think I should be getting -75 from that set
idk what you mean with solving - Do you want to find roots?
and idk the code you wrote
thats later, im trying to solve the vertex with this point
ill get the code for ya
I have no idea what you mean with solving a vertex
Do you want to evaluate the function at a specific point?
I have no idea what you mean with vertex
basically, the vertex is the point at which it intersects with it's line of symmtry
or the point at which a parabola changes direction
so if one side is facing one way, the vertex is the point at which it mirrors itself
-B/2*A
is -(B/2)*A
I think you might want -B/(2*A)
Thanks, hope this works
now im getting -1.25
I should be getting -7ish
what did I do wrong?
What exactly did you enter? What is the exact code you executed now?
And why should it be -7 instead of e.g. -1.25?
-5/4 seems like the correct value when I view a parabola with a=10, b=25
the point of which a parabola with this set of values mirrors itself is at -7 (values being A=10, B=25, C=8) , the program spit out -1.25 instead. here is my current code which seems to work just fine apart from the vertex calculation
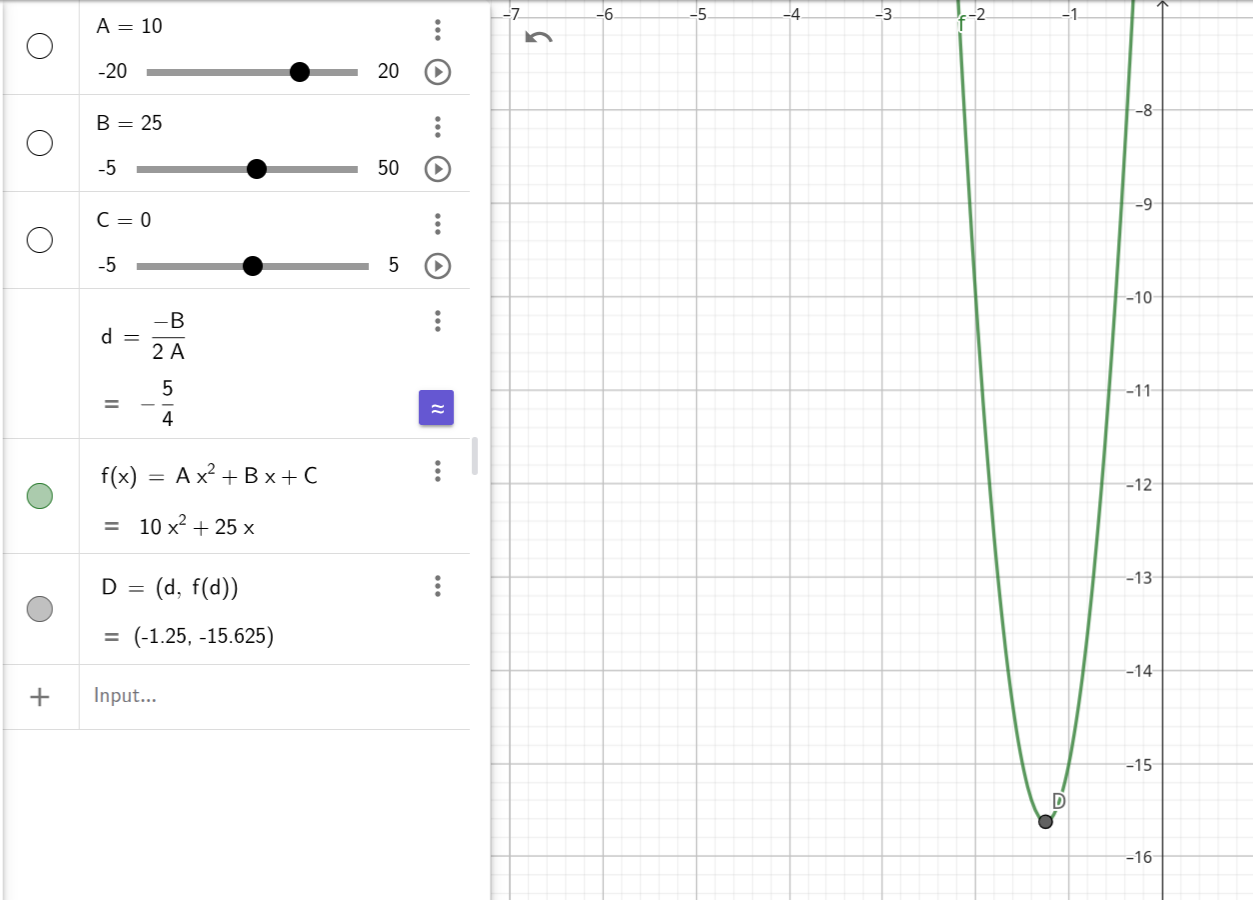
you forgot to add C being 8
That doesn't change the result
here's with C=8
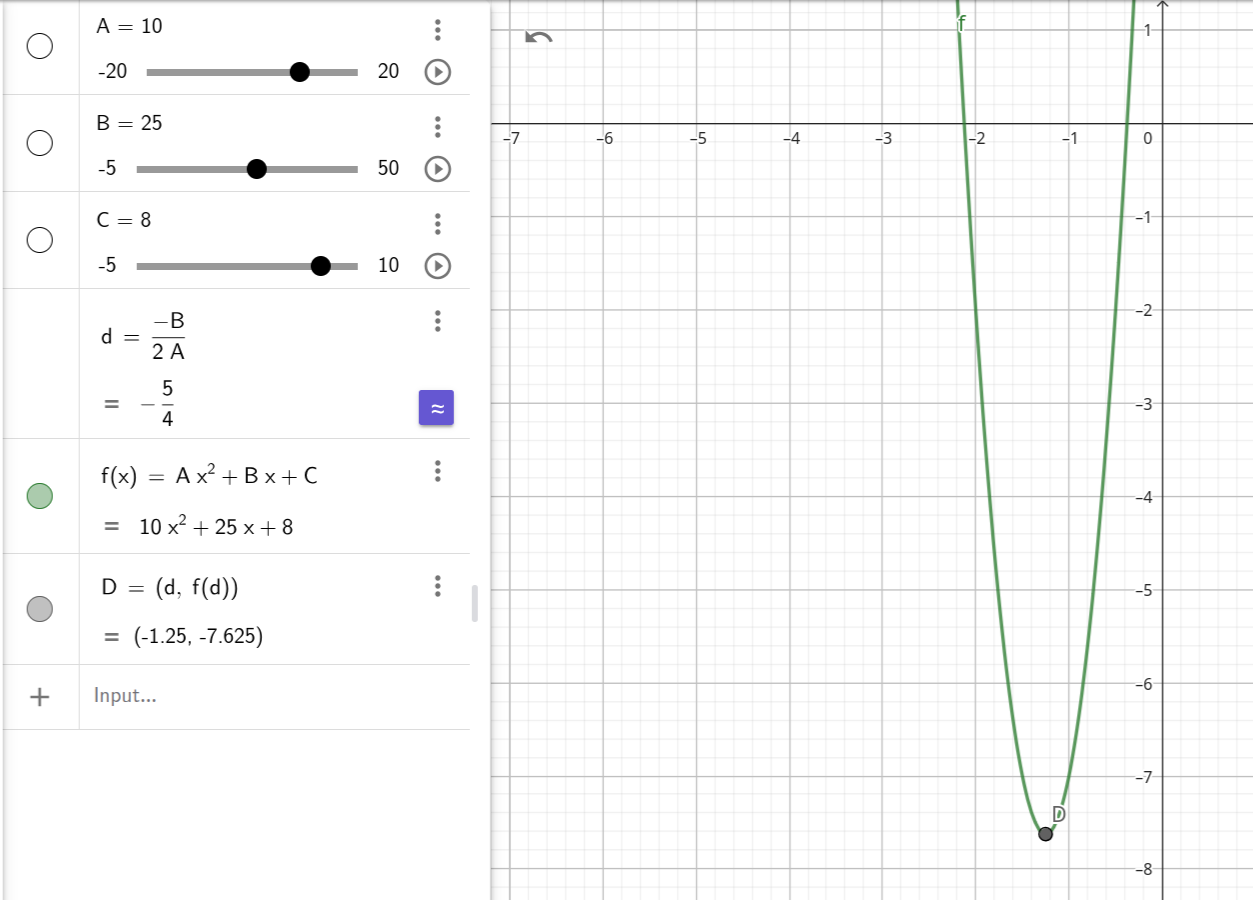
but I created it before you wrote the thing about C=8
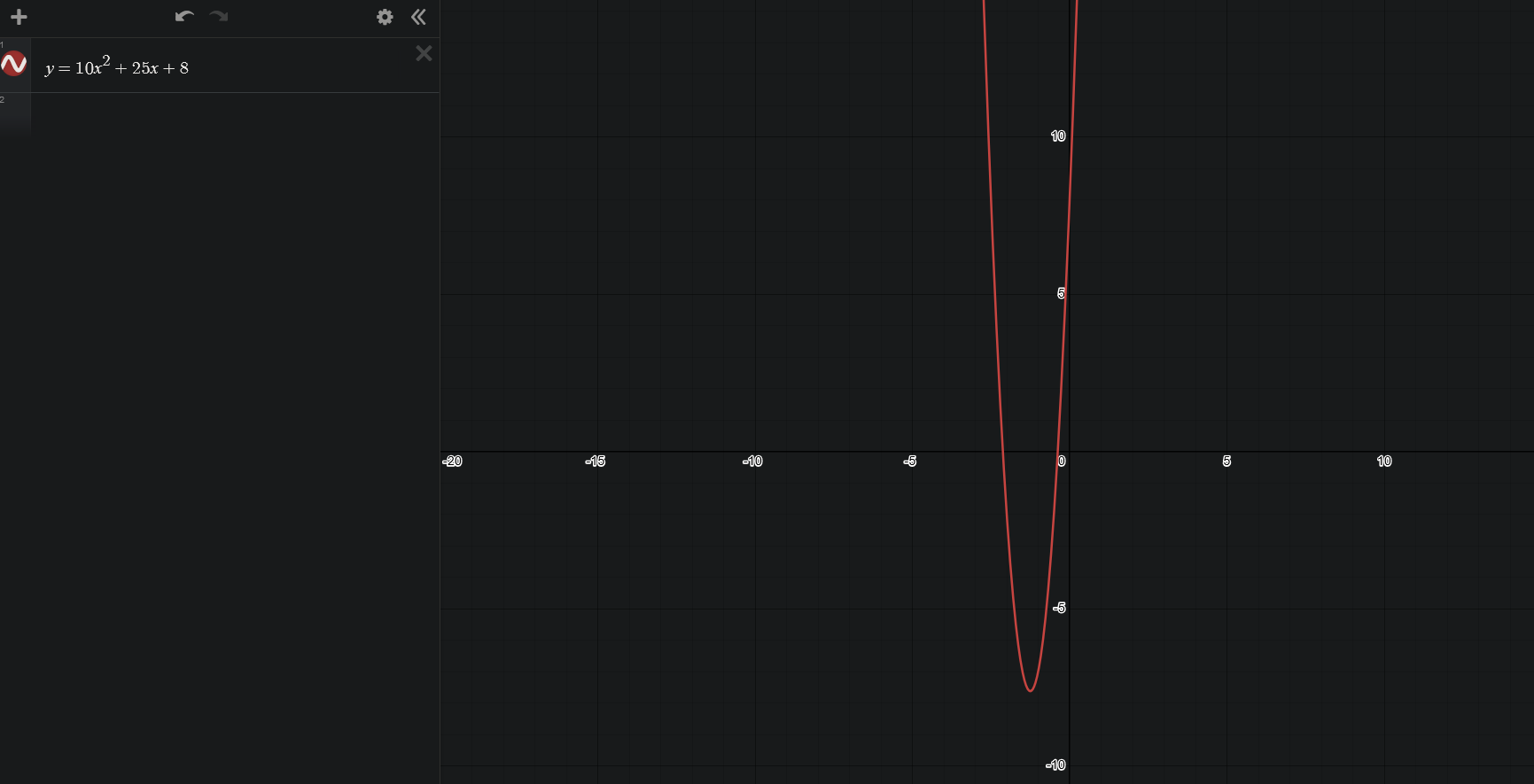
thats where it should be
that's pretty much -1.25
Do you want the y-value? If so, you'd have to calculate it by applying the function
its -7.8, how is that -1.25????
lol
now that seems a bit rude of me, sorry about that
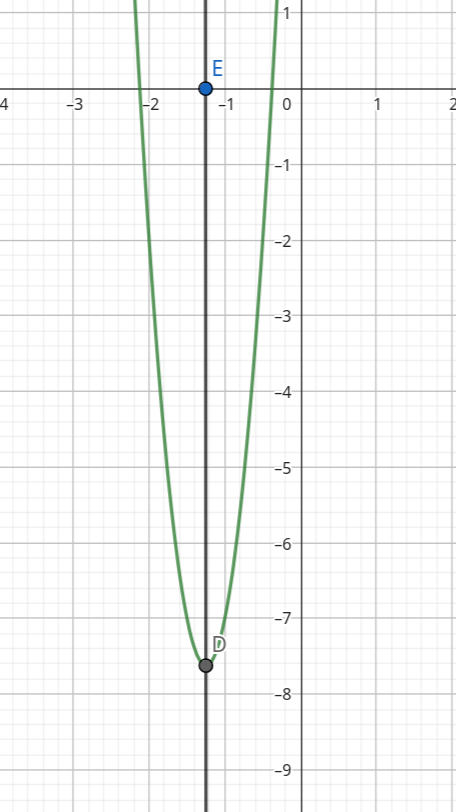
now that makes sense, Ive forgotten how to do parabola calculations, Im sorry for my oversight
no problem
wait, how do I do squared in java?
x*x
or Math.pow(x,2)
ight, and im trying to apply the xVertex value to the original equation but it keeps erroring out, I'll share the code
^2
is not squaringnow that makes sense, but what about the rest of it?
was that the only fix?
idk, what is the error you are getting?
how do I fix the "possibly lousy conversion from double to int" on the xVertex = vertexformulaX; line?
doing a proper square fixed the first issue
change the
int xVertex
to double xVertex;
int
means it's for integers i.e. no numbers with a decimal point
double
is for floating point numbersand, why am I getting a new error on the last double line before my final printing line?
because if you do string concatenation, the result is a
String
not a double
double
is for floating point numbers, not for textadding the String header to replace the double header still didn't fix the error
What's the error?
and idk what you mean with header
cuz im trying to print out the vertex form of the equation but I can't do it straight out with the println function
idk what the issue is if you don't tell me
btw
vertexFormulaY
just holds the value, not the whole formulayeah, so I'm trying to use the string VertexForm to hold the final formula without calculating it so that it can get printed out in the println fuction below it
What happens?
well, I get a giant block of errors due to the way its formatted presumably
"X" + + " - "
you have + +
therethe two main errors I get are ";" expected and that it's not a statement
I don't think that's intended
.
fixed it, but I still get the same errors
I still have to do the roots after I fix this part
I can't tell you what the issue is without seeing the errors
my guess is unmatching {}
I added the {} brackets but it give me more and new errors
such as "}" expected and ";" expected and not a statement
hmm, and I still need to do the zeros after I get this part done
heres the up to date code
if you want me to help you, you need to show me the exact errors
I did
right here
its on the String [] VertexForm line
oh
String[] VertexForm = {"y=", + A + "(" + "X" + " - " xVertex + ")" + vertexFormulaY};
is not valid
Do you want a single String
?
if yes, just do String VertexForm = "y=" + A + "(" + "X" + " - " xVertex + ")" + vertexFormulaY;
yeah, I want that string to print out the actual one when running
.
gives me the "";" expected" and "not a statement" errors
on which line?
the string VertexForm line
How did you change the line now?
I copy pasted, and replaced the old string line
ah you forgot a
+
symbol
before xVertex
that fixed it, I jsut tested the code and somehow the program got -148.25 for the vertex
idk how
you did
vertexFormulaY = A*xVertex*A+B*xVertex+C;
That's A^2 x+Bx+C
so how do I fix it?
read the line and ask yourself why it does that
ohh wait, the lack of brackets right?
no
what else could it be?
im honestly at a loss
What do you want to do with that line?
get the Yvalue of the vertex
Using the formula for a parabola.
What is the formula for a parabola?
y = Ax^2+Bx+C
And what's that line doing?
squaring A and adding the rest together?
I think
idk
thought it would work
Is A squared in this formula?
A*x is
?
ohh, wait
so I gotta add brackets then
no
what else could I do?
What is squared in the formula?
A*x is not what's squared
A is squared? sorry, its been a while
In the formula for a parabola? No, A shouldn't be squared there
Are you doing that? Yes
Is it done in the formula for parabolas? No
so x is squared then? lol
yes - in the formula
so I jsut change the 2nd A to an X?
hm?
so I do xVertexXVertex?
ranther than XVertexA?
there we go, that fix it. now all I need to do is the roots
and then im done
idk how to translate it into java code though
would you be willing to help me out with getting the steps translated?
maybe
maybe?
wdym?
why "maybe"
what would it take for you to help me translate the steps?
depends on whether I'm sleeping, whether you are showing what exactly you have an issue with, etc
well, I have been showing you what i've been struggling with
and ik you aint asleep rn lol
how would i translate the y=a(x-r)(x-s) into my program?
I think thats the only formula I need for the roots
unless im wrong
you sue
*
for the multiplications
or do you mean finding the values r
and s
?yeah, cuz I don't fully remember what r and s co-respond to
I just need a working way of getting the roots from A B and C
the roots which you can get using the abc formula?
and the X and Y values for the vertex if needed
abc formula?
yeah, how would I use the data given to find it in java?
cuz I found a formula D=B^2-4AC
idk how well it would work in java but if I could get it working, that would be great
cuz I need to display the roots after their calculated
The abc formula is
(-b +- sqrt(b^2-4ac))/2a
and this would allow me to display the roots?
this is the formula for the roots, yes
one root with
+
, one root with -
anything specific I need to do to show them?
if your task requires anything specific
$$
\frac{-b\pm\sqrt{b^2-4ac}}{2a}
$$
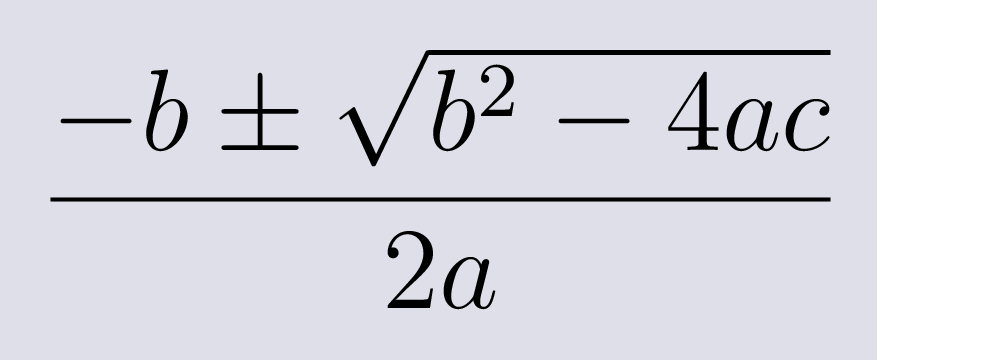
^ that formula
would needing to print both roots count?
Doesn't seem hard
and the original formula for the roots gives me "")" or ";" expected", "";" expected", and "not a statement"
because the formula isn't valid Java code
you have to translate it first
how?
What do you think?
idk lol, im terrible with java
and would I need to use two different formulas?
yes
one with
+
, one with -
ohh, thats what that meant
and you can calculate square roots in Java with
Math.sqrt(PUT_YOUR_RADICANT_HERE)
wait, you mean one with -4ac and one with +4ac right?
no
there is a +- symbol in the formula
Maybe you should first learn how quadratic equations work before implementing it
oh, wait a minute, now I remember
thanks for all the help dude, its been a few months since i've done anything with parabola's
are these the right ones?
double rootCalculation1 = (-B + Math.sqrt(B * B - 4 * A * C)) / (2 * A);
double rootCalculation2 = (-B - Math.sqrt(B * B - 4 + A * C)) / (2 * A);
looks about right
cool, thanks for all the help with this
Post Closed
This post has been closed by <@595765455436644367>.