How to display api json data into a wpf page
So I am making a flight Booking app and I am using Amadeus Flight API right and I want the user to search for Flight using a Form but I get this odd error Exception: The JSON value could not be converted to System.Collections.Generic.List'1[FakeFlightBookingApp.Model.FlightOffer].Path: $ | LineNumber: 0 | BystePostionLine:1. but it does seem to make the call to the API just fine and the data is displayed in the console log as you can see in the pic. I will give some of my relevant code
29 Replies
personally, I'd have models made for the API response, then translate those into viewmodels used by your app
also you can put
cs
after your triple backticks for code blocks to give syntax highlighting
$codeTo post C# code type the following:
```cs
// code here
```
Get an example by typing
$codegif
in chat
For longer snippets, use: https://paste.mod.gg/How to deserialize JSON in C# - .NET
Learn how to use the System.Text.Json namespace to deserialize from JSON in .NET. Includes sample code.
for some examples of how to deserialize json data to a model
thx
its odd because on swagger it works fine
well you are trying to deserialize to a list, and from what I can see in that screenshot the API isn't returning a json list
var flightOffers = await response.Content.ReadFromJsonAsync<List<FlightOffer>>();
granted that screenshot isn't formatted at all so it's hard to tell exactly what it's returningyeah I had it as something else and was still thrwoing the same error asked chatgpt and was saying to make a FlightOffer class
for starters, format the returned JSON so you can actually make sense of it
deserializing to a list is pointless if the returned data isn't a list
format and post the whole thing here along with your
FlightOffer
modelsorry format what exactly
the JSON returned by that API
yeah I will do that now swagger does it automatically
:PepoSalute:
got a feeling your models aren't right but won't be able to say why until that json is readable
yeah it takes some time for the swagger to do its thing
you can also copy-paste the unformatted response into vscode, then format with vscode
here u go
yeah, you are missing an additional model to handle the overall response object
your models only cover what is nested within the
data
section of that payloadoh so I need one for meta?
no, you need one for the entire response object
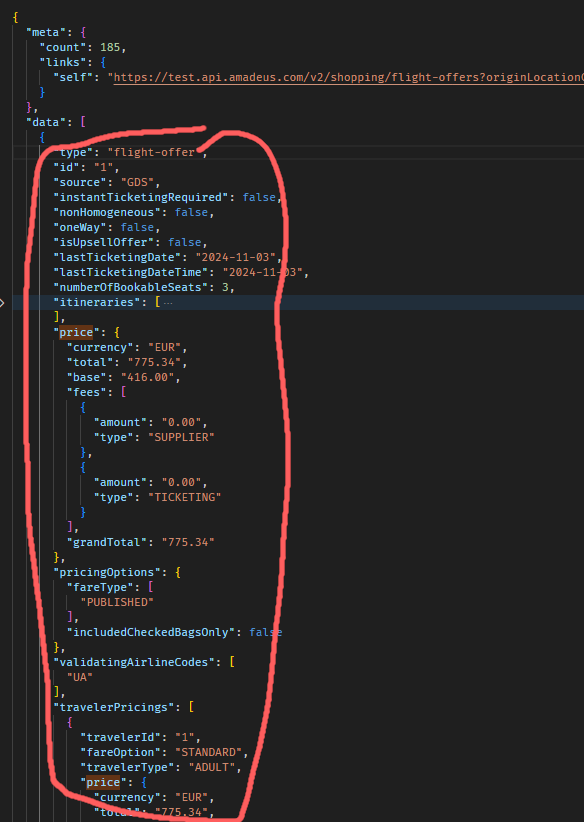
the circled part is what your models cover, which assumes your data comes in the form of
but you need to handle
so I need source, instantTicketingRequired too
etc
unsure exactly how
ReadResponseAsJsonAsync
or whatever it was named handles missing keys / properties, but you can probably ignore stuff like that
the important part is having a model with a list of flight offers inside of it
since that is exactly what "data"
is in your jsonok thank you
I get that will look into thx
gl
it works man th
tgx
thx
:PepoSalute: