Godot UDP Client not functioning properly
I am making a Godot project where I have made a basic fighting game which will take inputs from a raspberry pi pico controller i have made which is a UDP server which sends data packets to the UDP client on Godot based on button presses for it then to be interpreted into actions in the game. one of my objects is stuck as null and i cant seem to fix it along with a whole other issue with the packet detection running every frame
53 Replies
the code right now is:
image version:
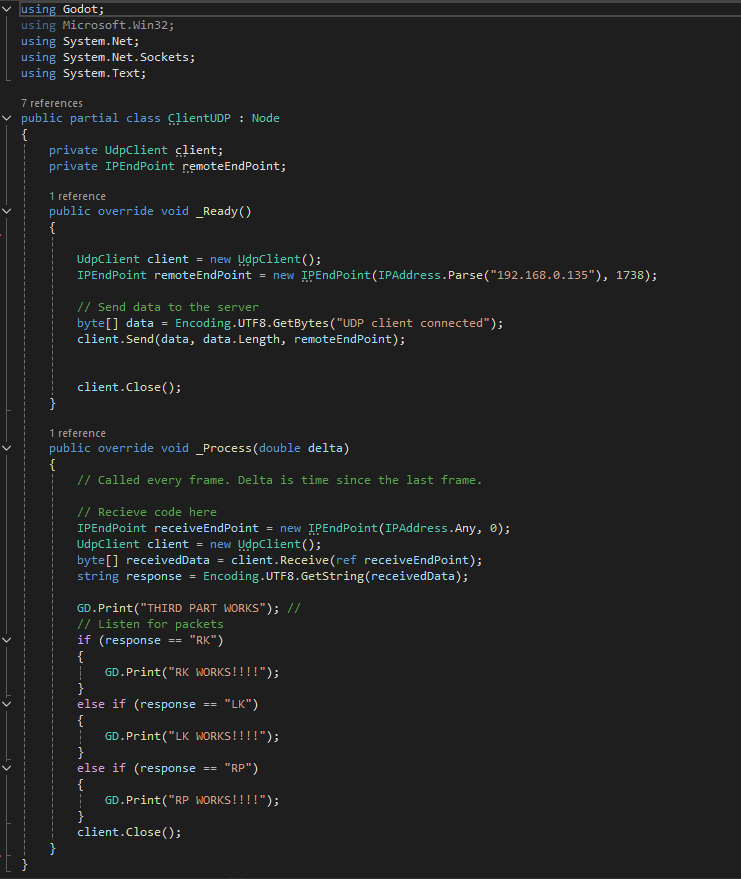
You never initialize client
Instead you create a new client every _Process and _Ready call
You should only need to instantiate one
Unknown User•6mo ago
Message Not Public
Sign In & Join Server To View
is client not initialised on the first line of _Ready?
Nope you create one and assign it to a local variable, meaning that variable only exists in _Ready
or am i missing something?
oh happy halloween
will assign the class member
client
to the value you want (UdpClient)oh i already tried this
the error still occurs, let me try again real quick
ah wait no its a different error now i forgot
E 0:00:01:0304 void System.Net.Sockets.Socket.ValidateReceiveFromEndpointAndState(System.Net.EndPoint, string): System.InvalidOperationException: You must call the Bind method before performing this operation.
<C++ Error> System.InvalidOperationException
<C++ Source> :0 @ void System.Net.Sockets.Socket.ValidateReceiveFromEndpointAndState(System.Net.EndPoint, string)
<Stack Trace> :0 @ void System.Net.Sockets.Socket.ValidateReceiveFromEndpointAndState(System.Net.EndPoint, string)
:0 @ int System.Net.Sockets.Socket.ReceiveFrom(System.Byte[], int, int, System.Net.Sockets.SocketFlags, System.Net.EndPoint&)
:0 @ System.Byte[] System.Net.Sockets.UdpClient.Receive(System.Net.IPEndPoint&)
ClientUDP.cs:33 @ void ClientUDP._Process(double)
Node.cs:2131 @ bool Godot.Node.InvokeGodotClassMethod(Godot.NativeInterop.godot_string_name&, Godot.NativeInterop.NativeVariantPtrArgs, Godot.NativeInterop.godot_variant&)
ClientUDP_ScriptMethods.generated.cs:48 @ bool ClientUDP.InvokeGodotClassMethod(Godot.NativeInterop.godot_string_name&, Godot.NativeInterop.NativeVariantPtrArgs, Godot.NativeInterop.godot_variant&)
CSharpInstanceBridge.cs:24 @ Godot.NativeInterop.godot_bool Godot.Bridge.CSharpInstanceBridge.Call(nint, Godot.NativeInterop.godot_string_name, Godot.NativeInterop.godot_variant**, int, Godot.NativeInterop.godot_variant_call_error, Godot.NativeInterop.godot_variant*)
nothing on visual studio though
This is more akin to what you want
let me try it
im not sure if flush exists on UdpClient, its convention though so, might be FlushAsync ¯\_(ツ)_/¯
no definition for flush apparently
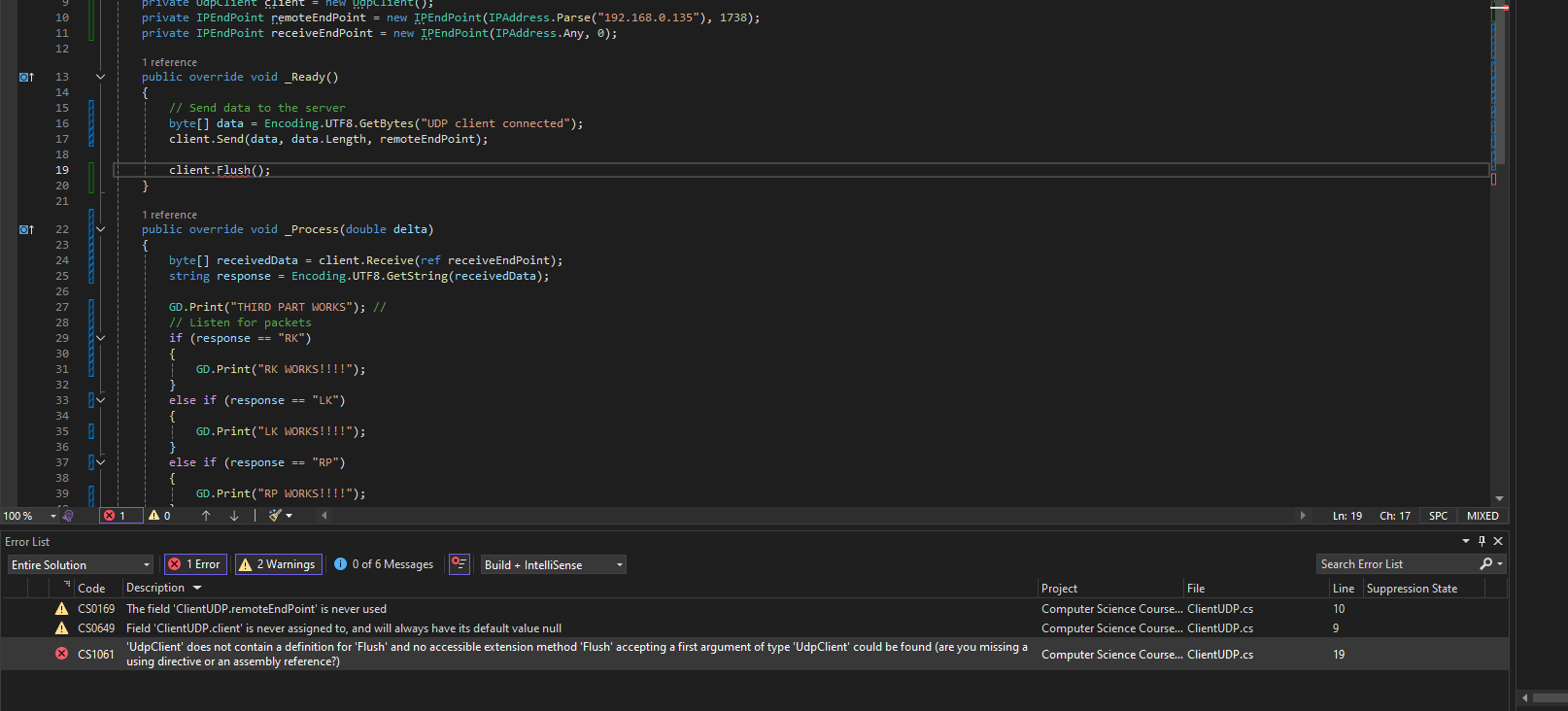
let me try async
Is there anything in intellisense?
still nothing for FlushAsync
Okay then just omit it
got it
still have 2 warnings on the bottom
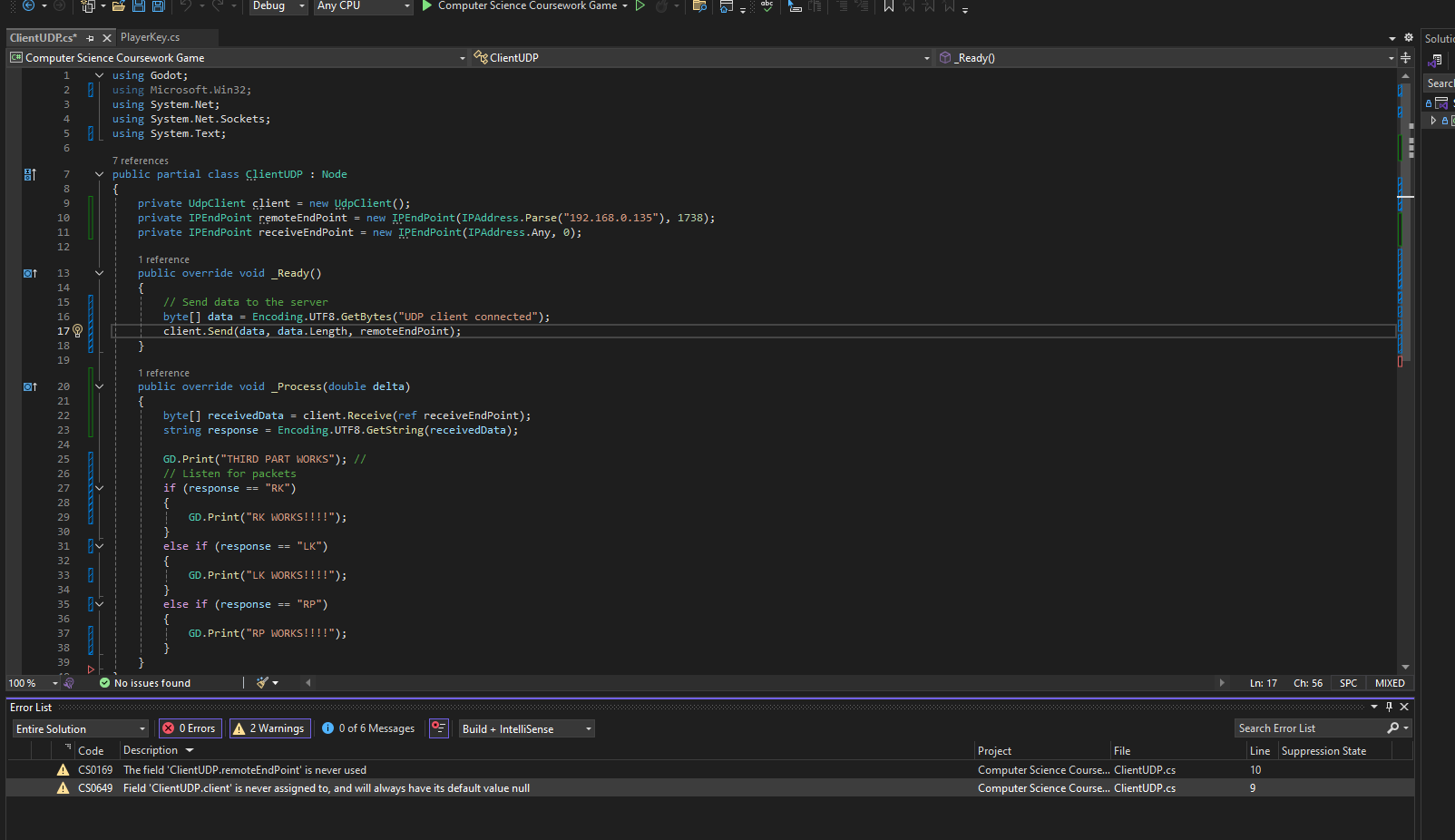
i think its just a visual studio bug at this point
i commented them out at one point and they still gave me the warnings
commenting is definitely not the solution
yeah i understand im just saying that the warnings are still given to me despite having removed the code entirely at one point
(did you save??)
yeah
its just being weird
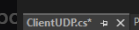
Did you?
yeah since the screenshot
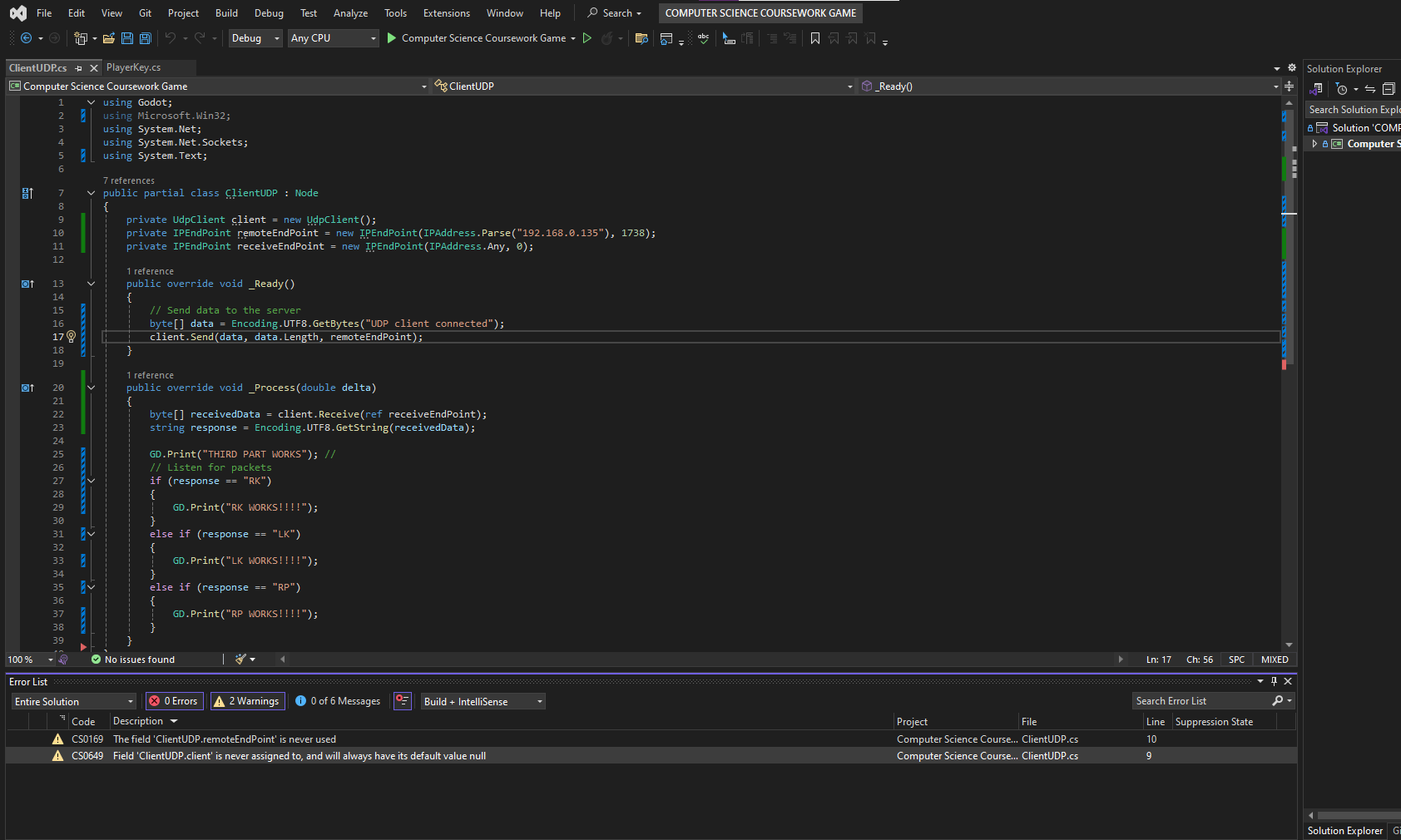
so it doesnt seem to be giving me any errors in godot
but
Those warnings seem old
it also doesnt seem to be loading into the game itself
yeah they are i think
promising results
i think i need a
client.Close(); at some point
the game wont load without it
That doesnt make sense
its like
stuck on the script
so it cant load the game
ill throw it in and see if it works
It shouldn't hang on _Ready()
You can remove the code from _Process() for now
comment it out or just straight up remove it?
comment
yeah the client.close seems not great
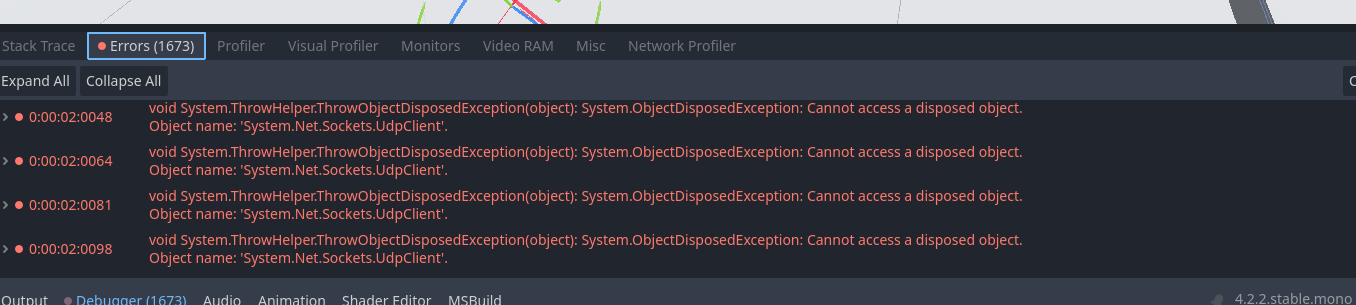
alright done
Yeah it seems like _Process() is at fault
removed the clients too
yeah i think its this one specifc line
byte[] receivedData = client.Receive(ref receiveEndPoint); this one i believe
If it blocks until it gets data, then yeah the game will get hung up
yeah
i should uh
Which is expected cuz you not using Async
i should probably start up the server huh
i forgot about that
doesnt seem to have helped
Are you sure the PI is 192.168.0.135 and its being hosted on that ip and port? If you hosting on localhost its not gonna work
yeah its on that ip and port
let me rerun some code to confirm
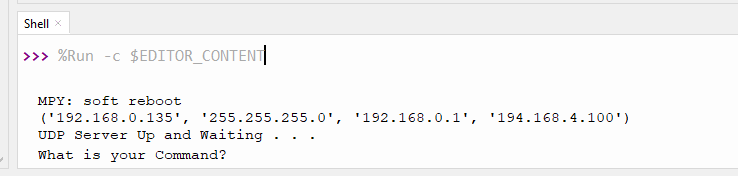
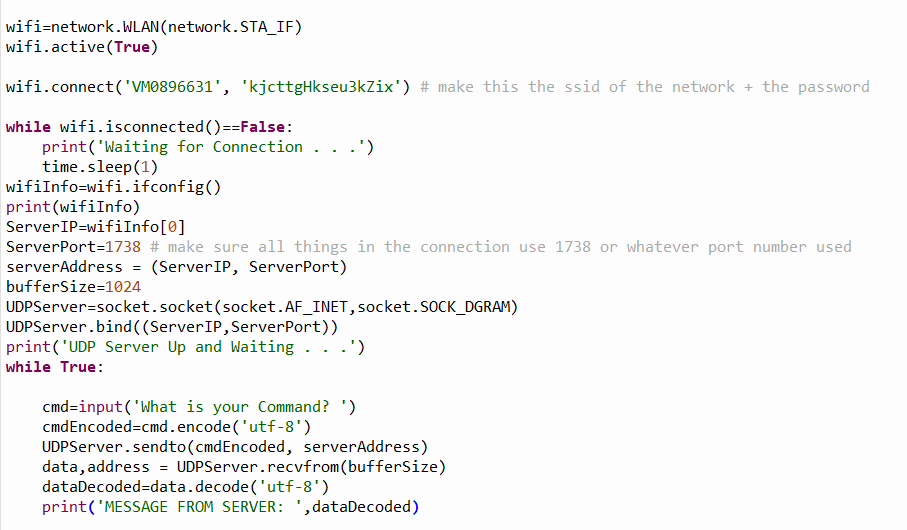
idk wtf I am looking at
oh yeah its python not c#
i shoulda clarified
basically the port is 1738 and the ip is 192.168.0.135
of the server
i cannot for the life of me figure it out