per pixel collision
Hi, I was just curious if anyone might know how to impliment per pixel collision into a project? I'm making a game for my A level Computer Science course - it's c# Monogame. The per pixel code is in each class that needs it, but how would I go about implimenting it in the game itself, and furthermore, should I put the code in a globals class instead? Thanks for any help in advance. I've attached screenshots of my code and where it needs to be used.
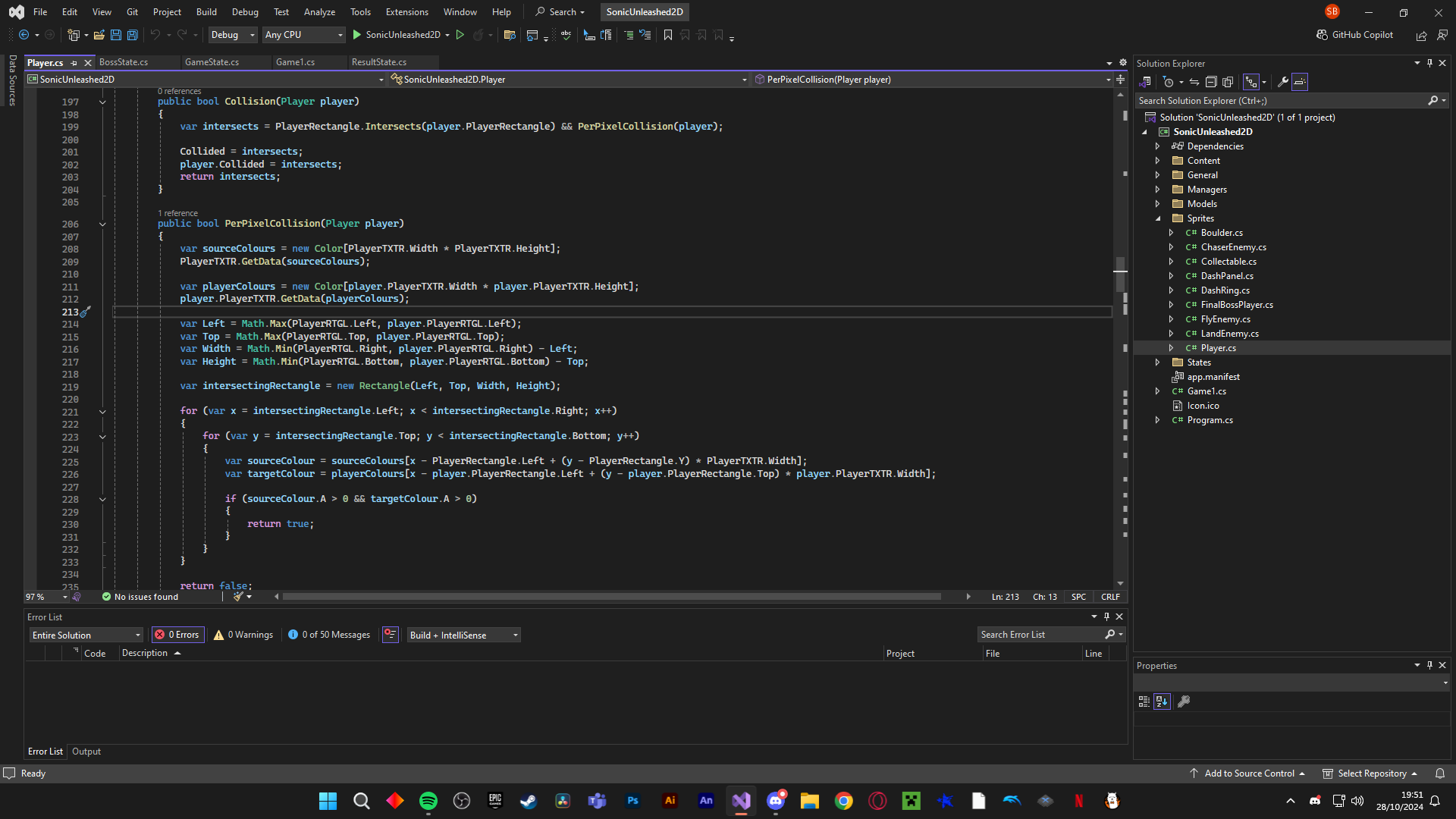
64 Replies
to add to this, I need 3 kinds of collision responses - one for collectables, one to move the player position and one for enemies. I'll attach an image of the game to show what I mean
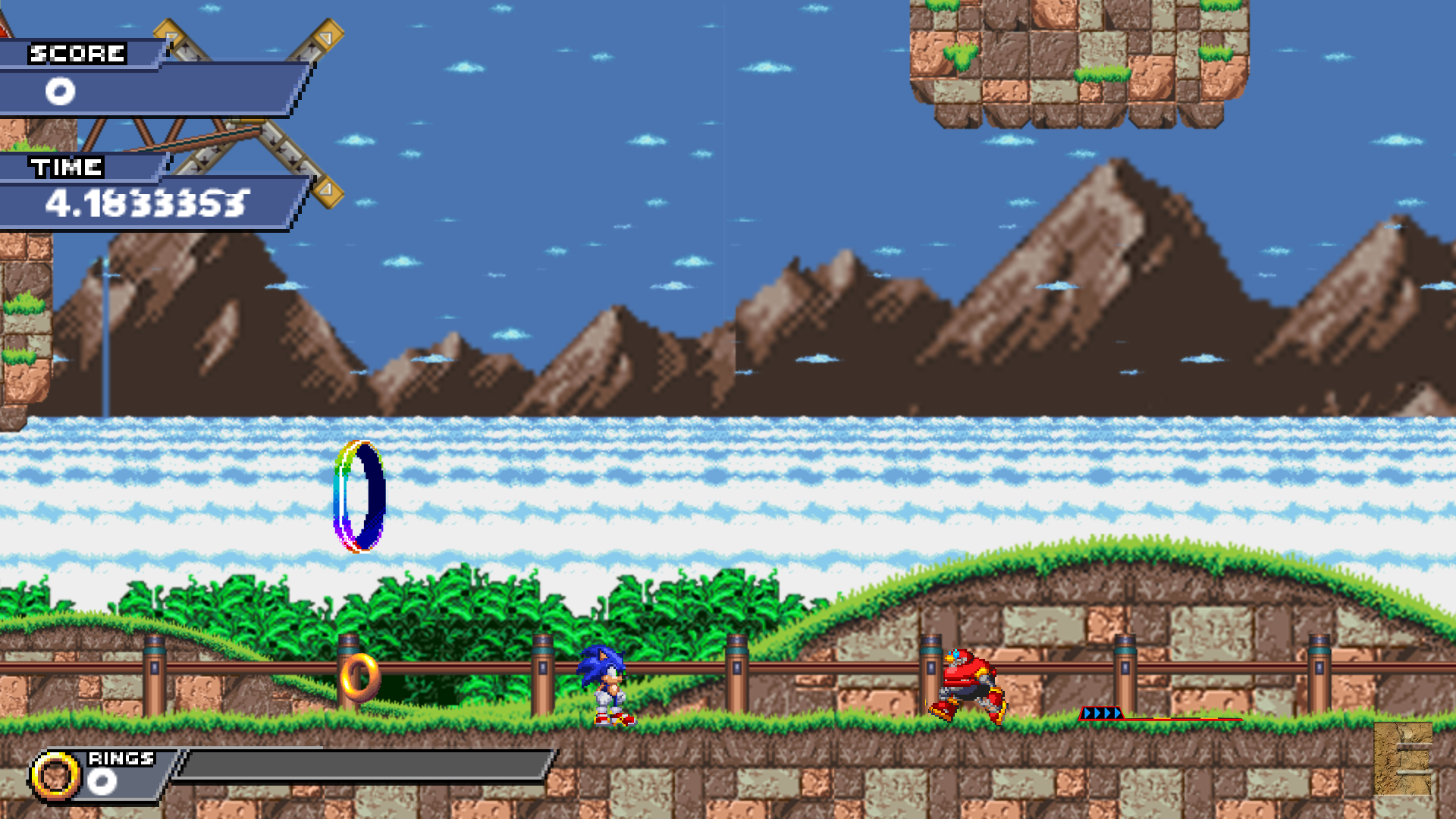
and you want the 3 of them to collide by pixel?
yeah, I need the three collision responses to respond to per-pixel collision detection
well for the enemy, first you have to check if the player's rectangle is intersecting with the enemy's rectangle
in line 199 it seems ur trying to check if the player's rectangle is intersecting with itself
yeah i think i am, i'm a bit confused on the logistics of implimenting it between multiple objects if that makes sense?
yeah i get what u mean
well first lets start with the enemy
okay one second, i
i'll take a screenshot of the enemy collision code in the class itself
i used the exact same code for the enemy as seen here
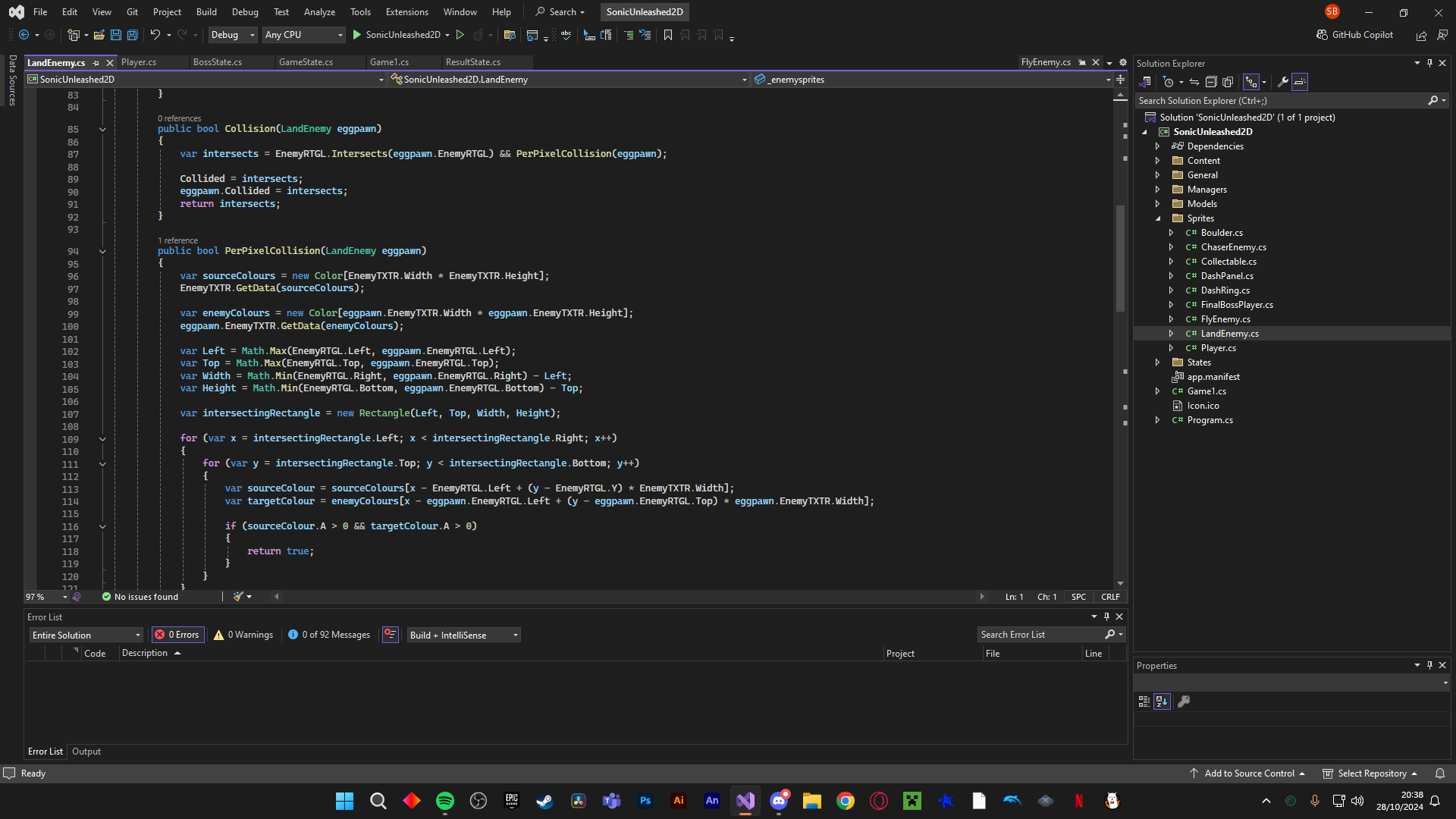
first try not to allocate a new array every time, this will affect the performance of your game
you should only allocate the array once, and add its color data
okay, so should i put the array outside of the bool method and call it in the method?
this is monogame right? the colors of the sprites are already stored when you load them
ohh okay, i didn't know that. for added context all my knowledge has come off tutorials and whatnot, we were given no beforehand knowledge of the engine. this is my first project also. so should i remove the array entirely?
yeah
what is EnemyTXTR?
its the texture for the enemy that the animation is drawn onto
is its type
Texture2D
?yes it is
i hear ya brother!
yeah the colors of ur enemy are already stored in that instance of a class
okay, i'll remove the colour data from the method then and try and rewrite it
u can probably access the colors directly
okay, i'll try that
hmm seems u cant do that in monogame 😒
then have the Color[] as a field
or theres a better way
use a bool array
each Color represents 4 bytes, we dont need to store 4 bytes in every element of the array, we can store only 1 byte for every element
we can use a bool array of trues and falses
you only need to check if there's a pixel in this position of the image or not
with that way you will save alot of memory allocations for your RAM
okay thank you! i'll give that a go and see what I can do 🙂 thanks!
remember, store it as a field instead of a local variable so u can only initialize it once
i will thanks so much for your help!
to greatly enhance maintability its better to encapsulate it in a class
this is really heplful thank you so much! I'll trace it through so i fully understand and use it as reference when i rewrite it!
for the intersection part u only need to loop on the intersecting pixels between the 2 rectangles, no need to loop on the entire pixels of an object
okay so, if i make the class static so i can acess it from anywhere, if i then feed in my objects in the gamestate class and set up what I want to happen if they return true, that should work?
what class? the one i provided?
yes that one
no? each texture has its own pixel collision
sonic has his own texture, egg pawn has its own
so should I just write what I want to happen when the rectangles collide in the classes or in the state they're used in?
what do u mean by state
hold on let me show you
does sonic have multiple textures? ie running textures, jumping texture, etc...
in this project i have states that are swapped between and the gamestates are where the objects will interact
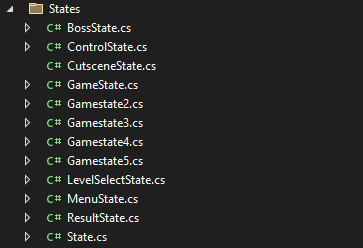
yes he does, all the objects are animated
yeah and each texture has its own color data
i'll show you how i did animations, i created a list for them in the globals class i have, one second
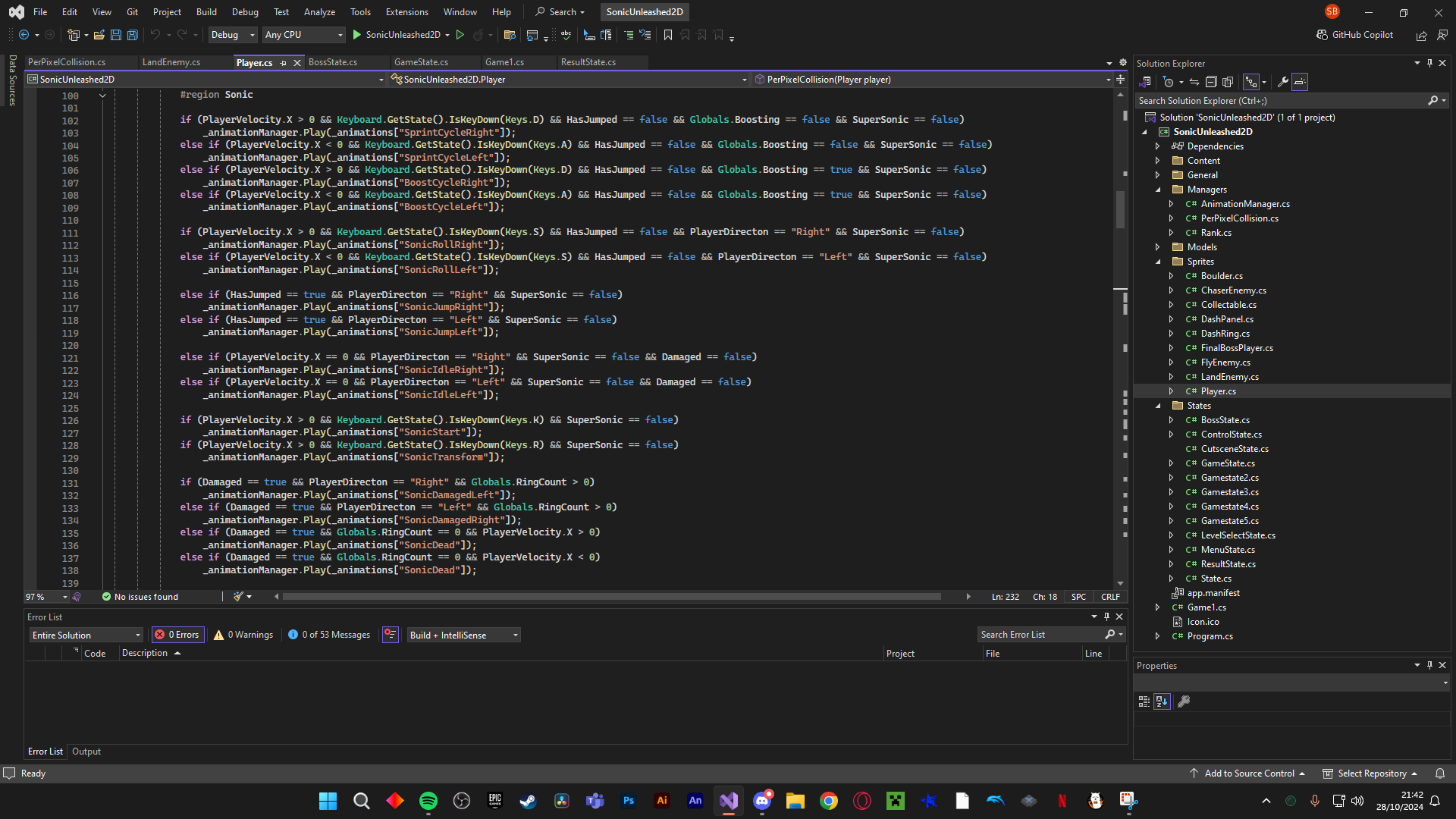
what is the type of _animations
well anyway this is monogame im sure ur using spritebatch
its the pass in of the animation class, the animation class sets the properties like frame width, height, speed etc
yes i use that to draw my objects
u have alot to fix later your code is a bit messy
yeah i know like i said this is the first project im working on so im still learning lol
ur gonna have to initialize this for each sprite
is each frame, say for the running animation, stored in each texture or are all the frames of that animation stored in a single texture?
they're stored in one texture i believe, i used sprite sheets and essentially it cycles to the next x co-ordinate corresponding to the speed of the animation if that makes sense?
ok
show me the class of _animations
okay one second
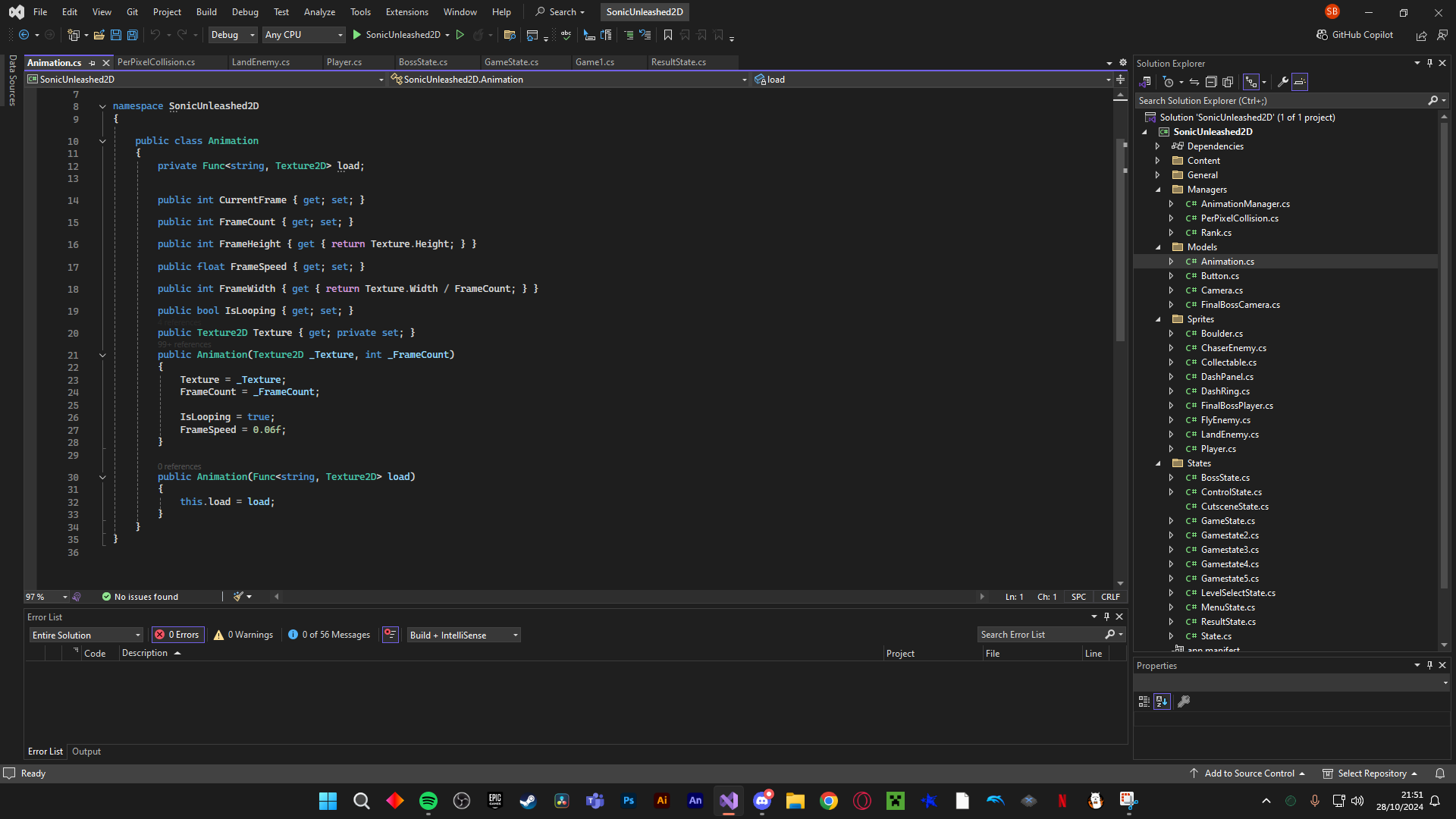
thats the animation class, as i said _animation is the pass in for this class
the type of _animations
is it a dictionary?
i assume its Dictionary<string, Animation>
correct?
yes it is
show me the Play method of _animationManager
so its a sprite sheet ok
then this wont work, we're gonna have to do some changes
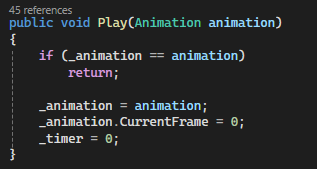
ur gonna have to initialize the bool array for each sprite
okay, i'll try that
i never coded the animation class this way
i just create a class called Sprite and store its position and size :when:
greater maintability, and readability
also judging by your animation it seems all the sprites in the sprite sheet are stored in the same row
its better to create a class called Sprite or Frame
okay, thank you, i'll try and fix it by doing that
it should be easy to fix
and it will be easy to access too
and it should be fine to store the pixel collision array into it
thank you this is really useful to look at, i'll compare this to the one i write myself. Thanks so much for your help!
thank you so much for your help 🙂
i need to go, maybe we continue tomorrow