Access Violations
I started using SUBSCRIBE_UOBJECT_METHOD() as per Mircea/Rex advice, but I still get an access violation.
Starting a test world with nothing in it / no other mods besides SML, everything works OK until I place a sign. The build animation plays and then there's about 1 frame where the sign tries to display something and then the game crashes.
26 Replies
Originally was following the pattern of Linear Override, but since
virtual float GetAdjustedEmissiveValue(int32 Level) const;
is well, virtual that makes sense. I then used https://github.com/Th3Fanbus/Th3SwiftSwim/blob/master/Source/Th3SwiftSwim/Private/Th3SwiftSwim.cpp as suggested as the overall framework for the mod so farYou didn't need the helper class (I used that for AccessTransformers), but it still shouldn't crash like this
Line 15 is
float NewEmissiveValue = BaseEmissiveValue * 10.0f;
I'm guessing something got inlinedI may want to hook in somewhere else, this just "seemed" easy ... but learning a new modding framework for a new game always is like this 😅
That's the thing, though. This should work
Let me check...
OK, perhaps I've messed something up in .h or build.cs
MoreLightingOptionsModule.h
.h looks fine
MoreLightingOptions.Build.cs
it's a pretty barebones mod for now while I wrap my head around everything, I feel there isn't much that can go wrong
That looks like it's based on Th3SwiftSwim?
That's correct, I restructured it to mirror that general construction
I think pretty much no one else sets
bUseUnity = false;
the one thing that's missing is a proper state check, but there isn't much exposed in that header file
That's why I knew
Okay, I can reproduce
Oh wow I didn't realize the cpp file was available in SML, that may help some - i thought I only had access to the header files
Which CPP file?
FGBuildableWidgetSign.cpp
That's just stubs
I think its just function definitions, but it at least has returns
Courtesy of Ghidra:
setting up a case system rather than a multiplier seems to function
I did something
Problem is that calling the original function didn't seem to work
Solution
is what I did, so basically the same
I like not using mutable variables 😄
cc @Mircea (Area Actions) any idea why hooking
AFGBuildableWidgetSign::GetAdjustedEmissiveValue
and trying to call the original would fail?it's bright
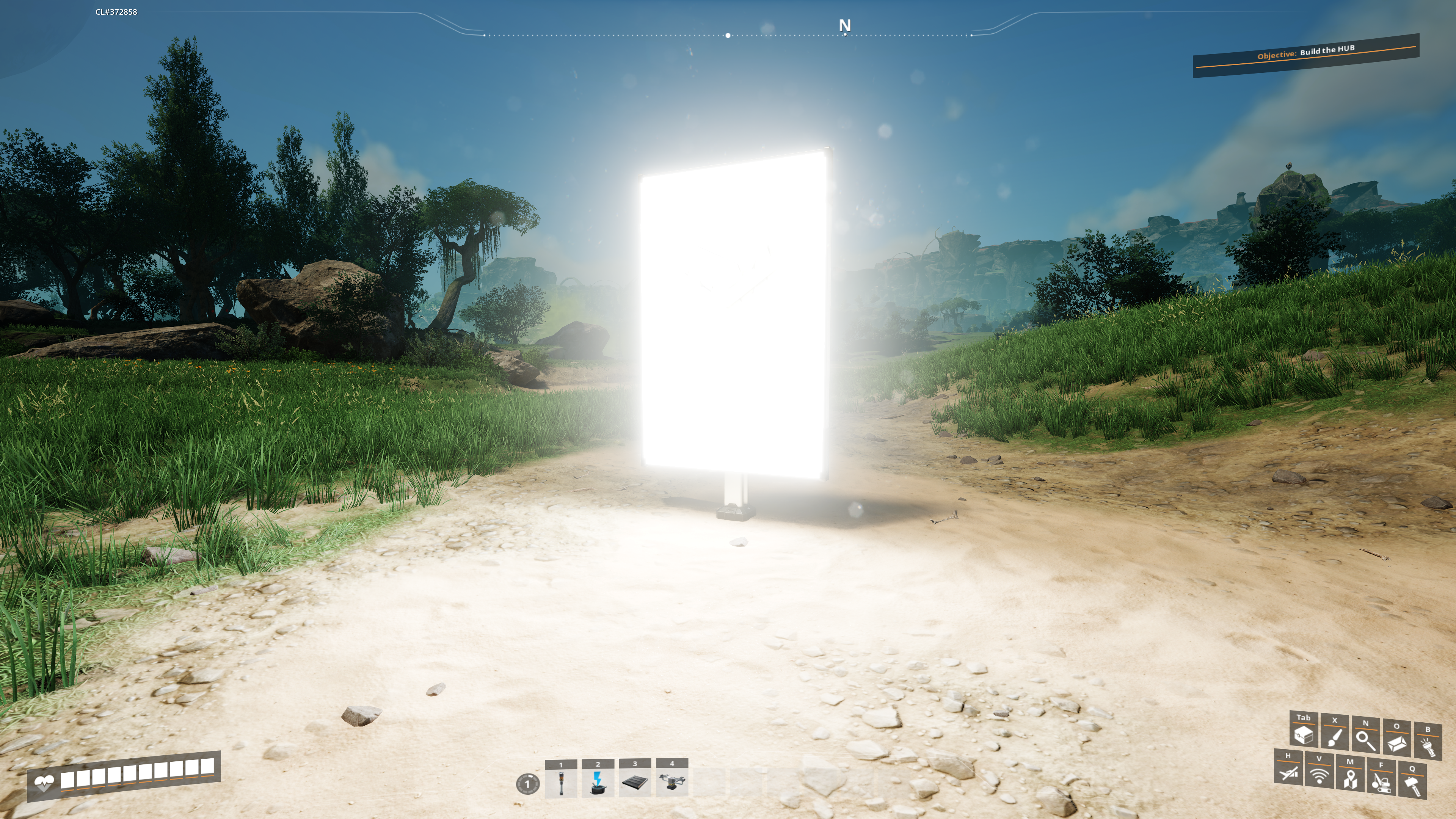
thanks for your help 🙂
Now I'm off to add some mod options, which I think will be easier on the blueprint side.
I just have to figure out how to access those from cpp
Be warned: accessing options from hooks is a pain
Where there's a will there's a way ... eventually