Context Commands Registering
I try in more than one way to record my commands, but they do not register
my code:
any help plz?
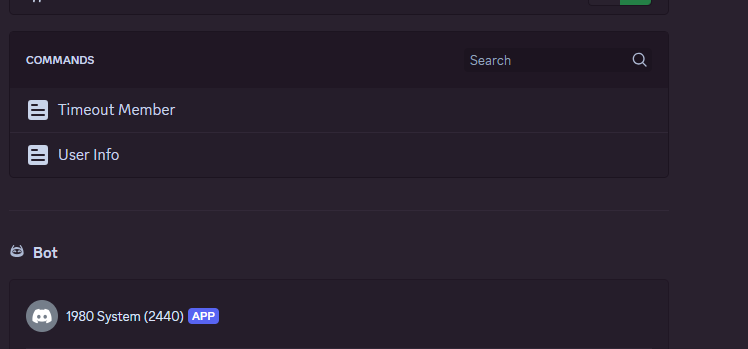

13 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPWhat makes you think they aren't registered?
this

Reload Discord
i do
As in, the app you're using to talk to me right now?
And not your bot
Because your first screenshot suggests they are registering if they're showing up in your integration settings
I don't know, applications appear normally for me, but my application does not appear
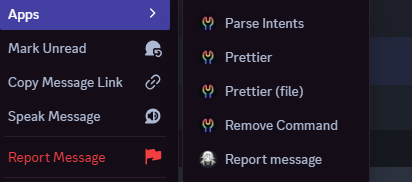
Fine, thank u
ur using ApplicationCommandType.User, which makes user context commands like this
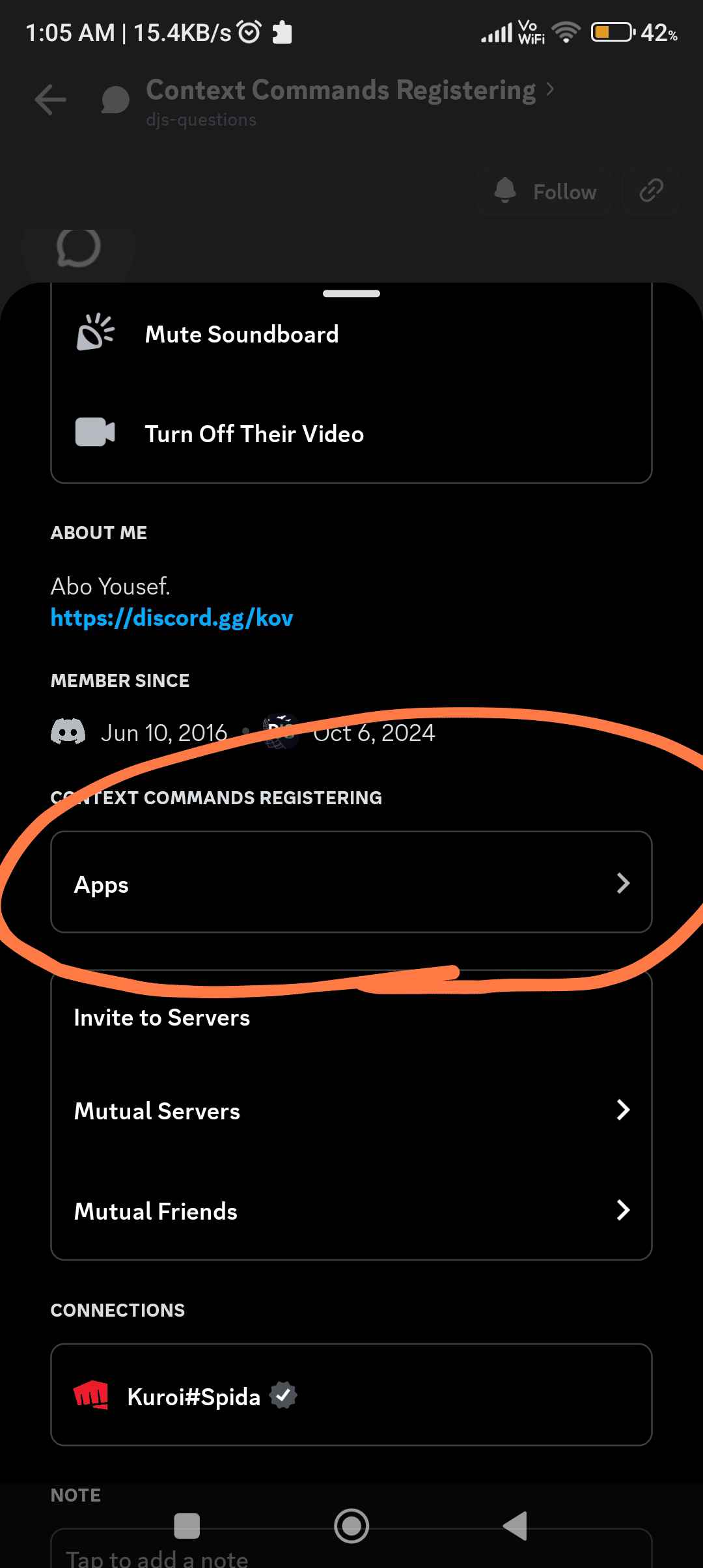
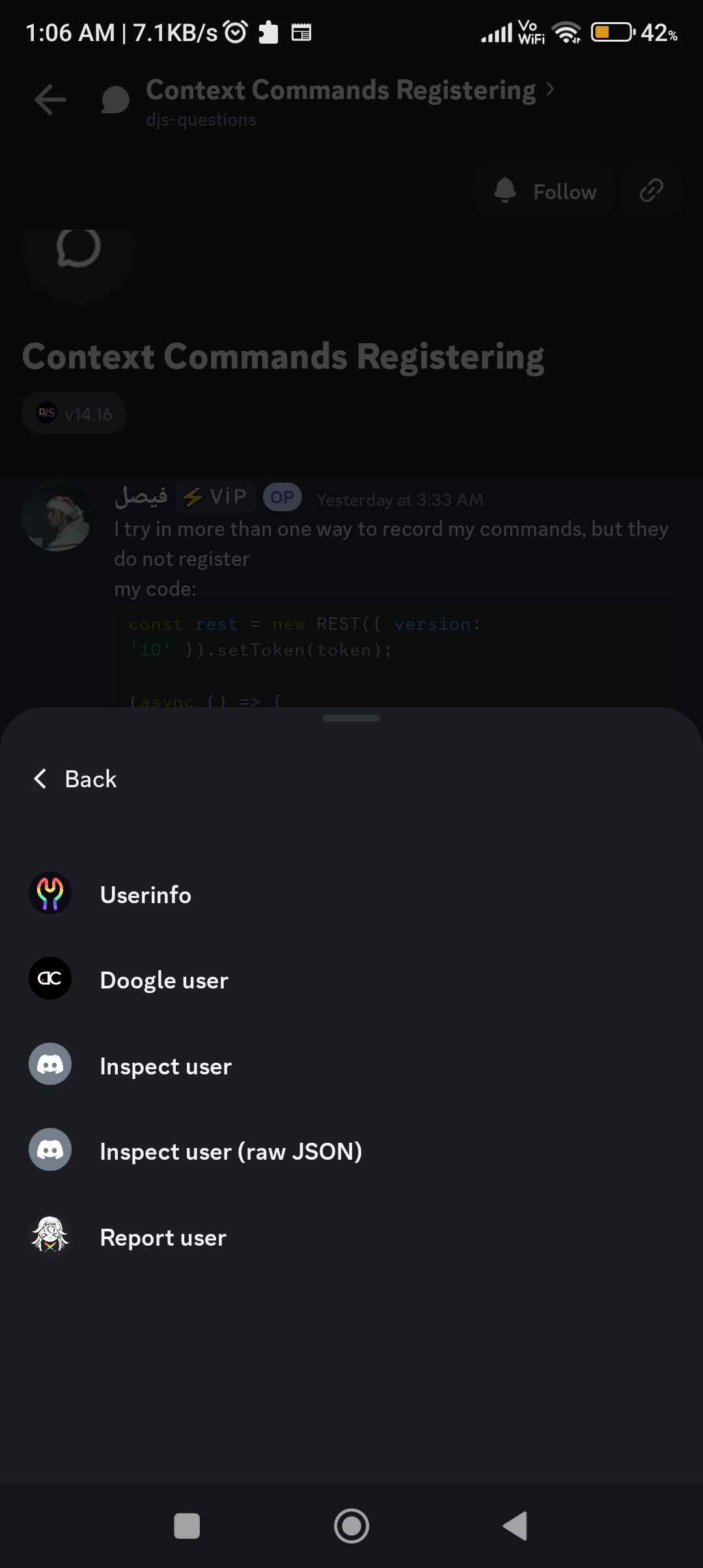
use ApplicationCommandType.Message as the type for msg context commands
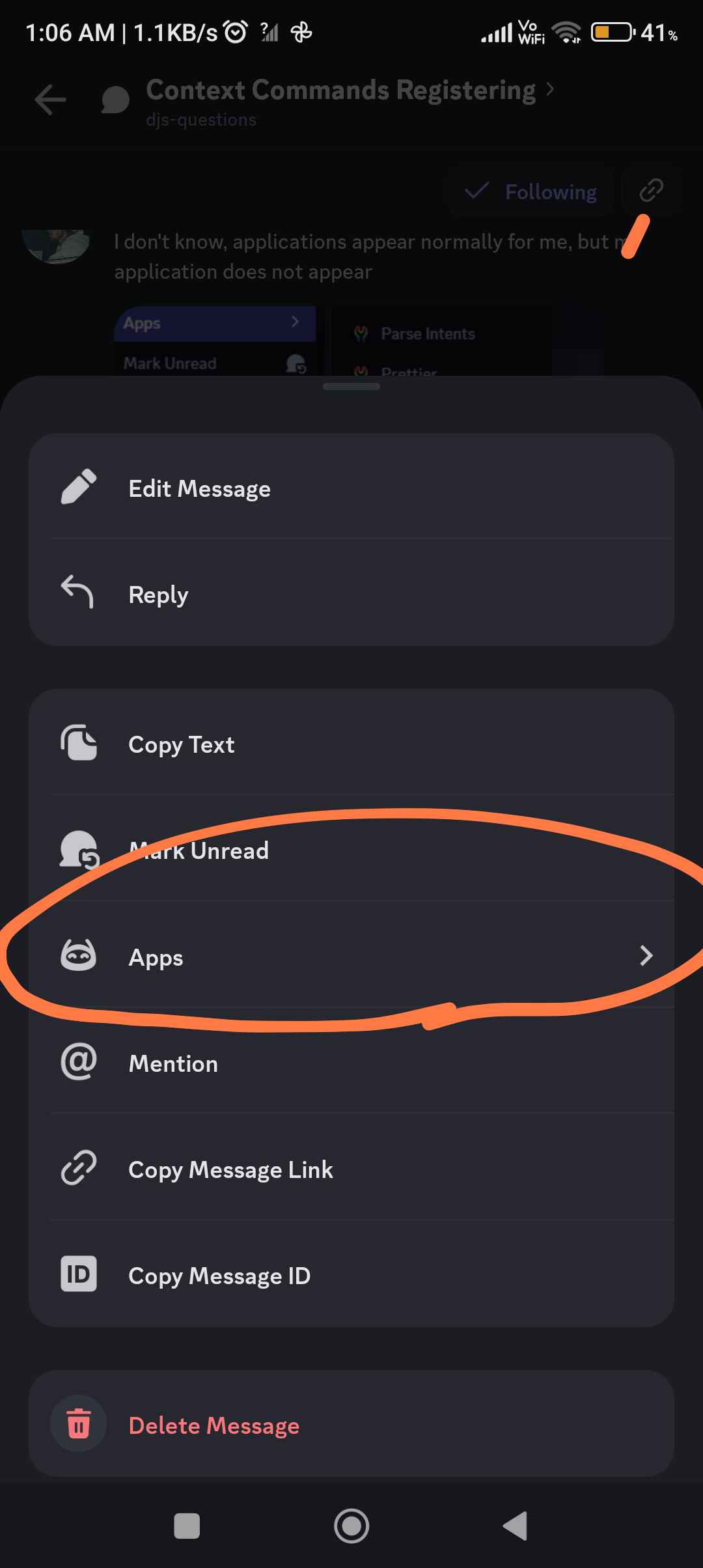
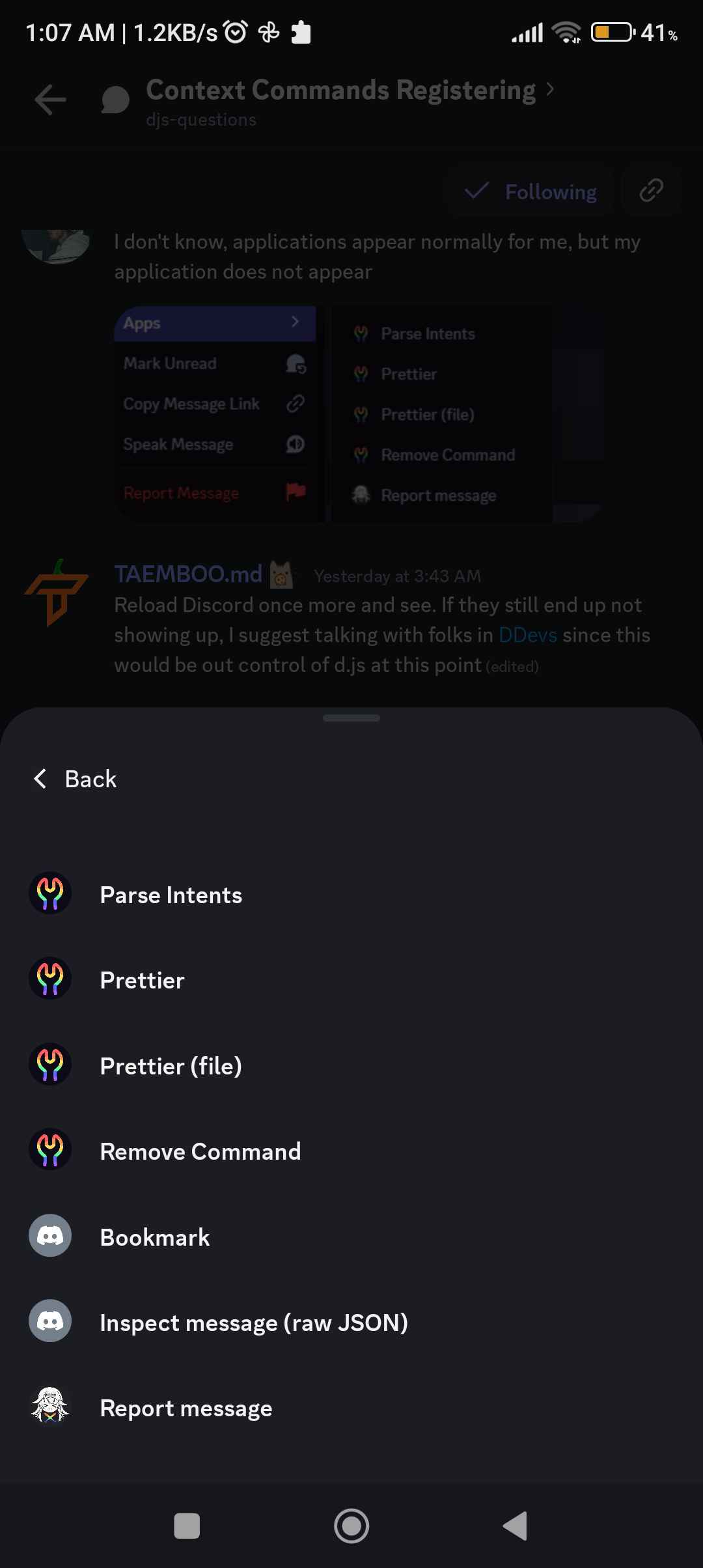
ok lmao ty