One click image upload form
I want to create a one click image upload button, ideally using next JS's server actions.
i would like to have a form, in which the only element is an input field that the user can upload a file to, as soon as the file is uploaded - I want the form to be submitted, and the server action to run.
Currently - i am doing this like so.
This does not seem to be working as intended, as for one the page is refreshing when the file is uploaded (how do i do something similar to e.preventDefault using the form.submit() method to submit?) and the action is seemingly also not being hit/ran.
Could anyone give any tips on how they've / would go about achieving this? Thanks.
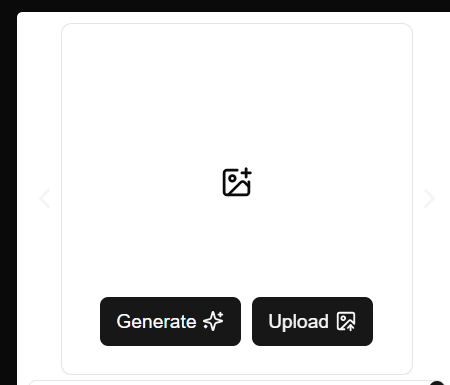
0 Replies