Migration issues -EF core.
I am creating a DDD application with EFcore but I am not able to use the migration because of the following error.
Does anyone know how to solve this? can provide code snippets if needed

13 Replies
Can you show the Order class C# code?
Normally, you should not put related entities (Customer and Location) in the constructor parameters...
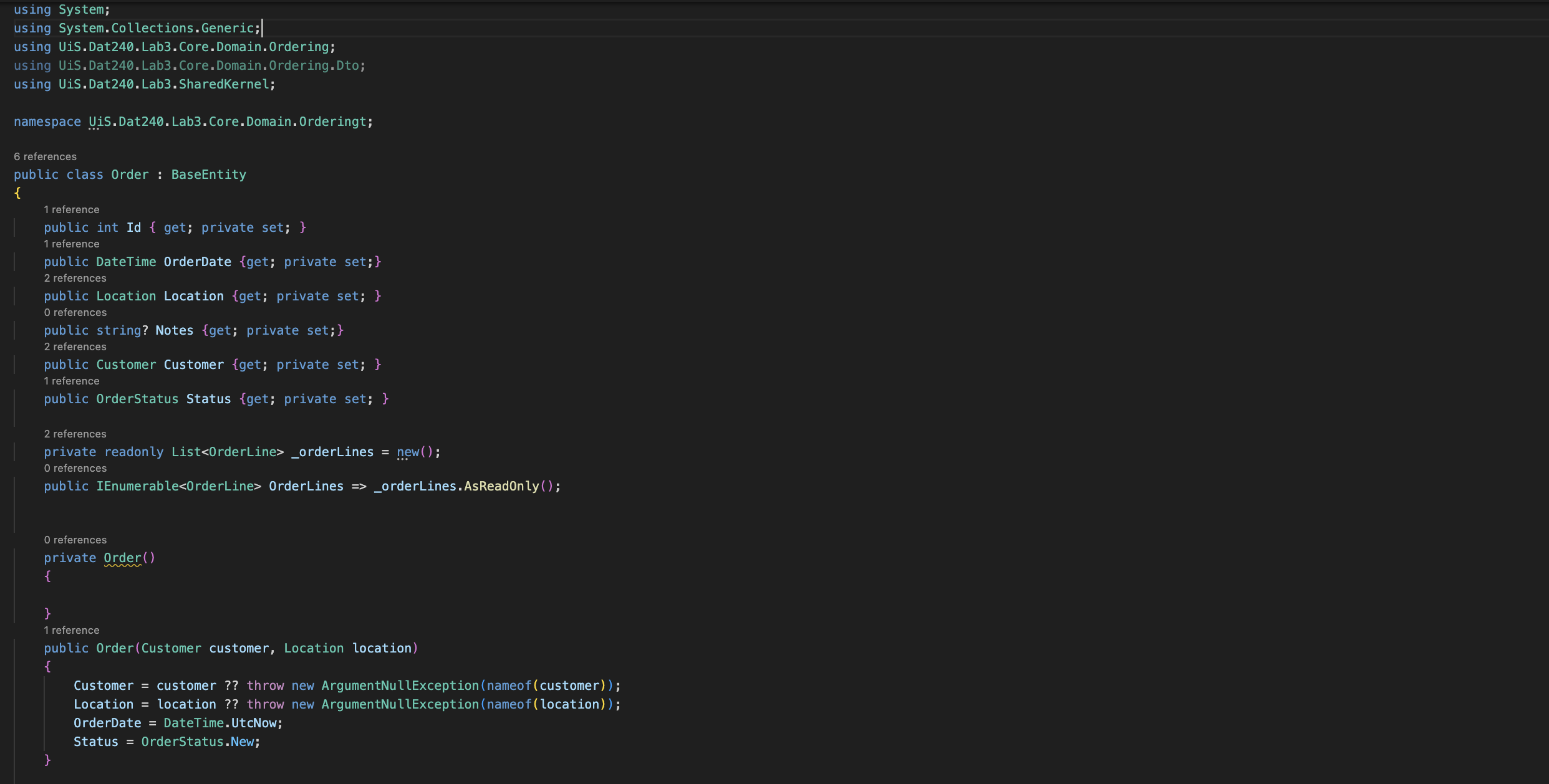
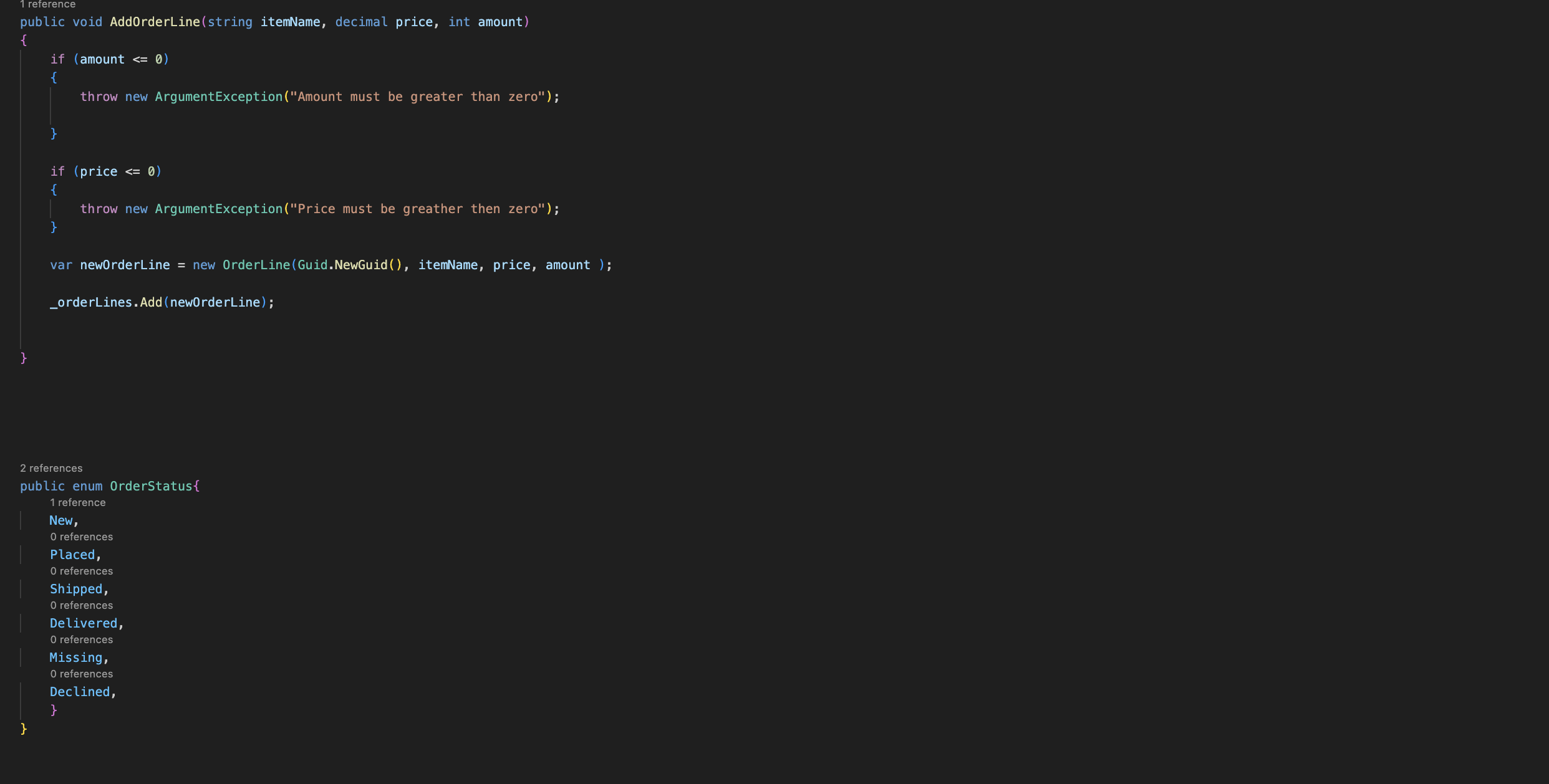
Just remove the constructor with arguments, and set the navigation properties with public getter/setter.
For example:
Because I am using domain driven design shouldnt I use private setters instead?
EF required public setter properties, you can't define the properties with private setters.
Thank you! I really need to read more about EF-core, do you recommend microsoft doc or any other sources?
The MS documentation is enough:
For example, read this page : https://learn.microsoft.com/en-us/ef/core/modeling/constructors and this one https://learn.microsoft.com/en-us/ef/core/modeling/relationships/navigations, you will see the limitation of navigation property.
If you want to do a real domain implementation, I advice you to create :
- Your domain with entities as you really want (with OO style, constructor, value objects,...).
- Create entities for EF which will be used only to query / persist your entity of the domain. You can use manual mapping between the model and EF Entity or a mapper like auto mapper.
We use this approach in my project, because:
- I want to use a real domain that reflect our business rules / entities.
- I don't want that developers deform our domain, because EF have limitations of the design for the entities. We use only EF entities to persist data and query using Linq.
With this approach, of course you will have the fellling to copy/paste entities of your domain to create EF entities.
But with this approach, you can make your domain entities independant of the structure of the SQL tables that you want to query/persist. Specially, it is very interesting when you have complex n-n relationship in your domain and you want to persist it differently in your database (with a JSON column for example,...).
In my projects, my teams make the EF entities as internal and are limited to the infrastructure/persistance layers and we suffix it with the
Db
name to avoid conflict with the domain entities and EF entities class names when doing the mapping.
And last thing, when doing this approach, it is easy to perform unit tests for our domain and our repositories separately.Entity types with constructors - EF Core
Using constructors to bind data with Entity Framework Core model
False, you certainly can and EF will use them. You can also use private constructors for EF to use.
it must however have a setter, or it wont work, but it can be private
For the navigation properties ?
yes
Oh yes sorry, I just read in the MS documentation that the setter can be private for navigation properties.
Yeah its sneaky, and I often forget it and make a read-only property by mistake 🙂
I just look some of my code, and I understand now why I put public setter for navigation properties... If I set them private, sonar will raise a S1144 warning that the private setter is not used...