Need help with fixing my rounded rectangle calculation
Hi! I'm making script for Unity. I'm bad at understanding floating points calculations and geometry, so it might be silly. Help me please
I'm making rounded rectangle. Either pieces are missing or lerp seems ignoring corners. Using unit circle x^2+y^2=r^2. Might be sqrt() or comparison conditions
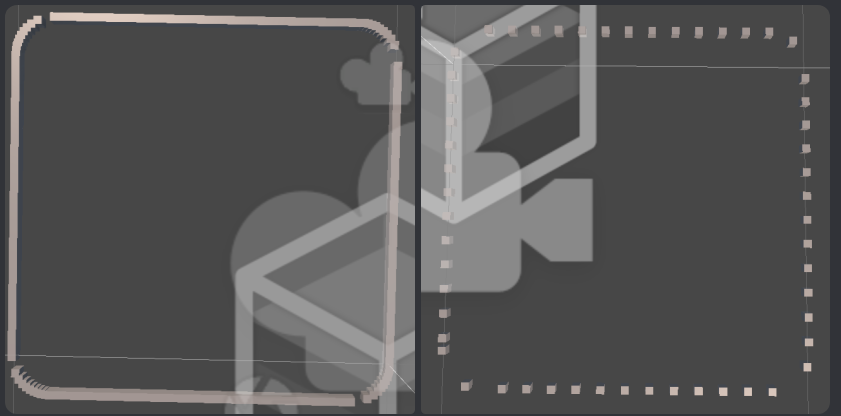
6 Replies
is a } missing somewhere? is RoundRect a constructor?
The code is working properly, the algorithm is not. RoundRect is a constructor, but the code i sent is not full because of the message length limit. The other methods i sent are full
I sent the constructor to show how i define width and height
Which is width without two radiuses
And height is height without two radiuses
I think the code is full enough to debug it if needed
Interpolate
doesn't look like it's ever called
main method seems to be GetPoint
but it's not shown how it's called, is it in a loop?I haven't brought the lines where I call the method, only the class itself
Interpolate runs for every point I want to get
I had a for loop. That goes by 0.01 up to 1
And that it used by GetPoint(Mathf.Lerp(0f, perimeter, t));
Which maps the point from 0 to perimeter
Actually I have my stackoverflow question posted by now and I described that I assume that the method I was trying to implement was wrong inherently https://stackoverflow.com/questions/79080391/im-trying-to-implement-rounded-rectangles-that-can-be-used-with-interpolation
Stack Overflow
I'm trying to implement rounded rectangles that can be used with in...
public class RoundRect {
float width;
float height;
float r;
float fullWidth => width + r * 2;
float fullHeight => height + r * 2;
float radWid...
Density of the points of one end of quarter arc of unit circle is different than density of the same points at the other end of the arc
So i had more gaps
I think it may be solved with angles and a new parameter for angle increment but I'm not sure
I was just using axis coordinate increment, not angles
I assume that was the reason but still not sure
I actually wasn't using
Interpolate
for the first picture.
It is
But i was calling Interpolate
the same but with for (float i = 0f; i < 1f; i += 0.01f) {
and it's the second picture
But the thing is I think the gaps emerge not because of the way I do this for-loop but because it requires more density to cover other end of the quarters rather than equal interpolation. I may be wrong about this though
Also what I want is not to draw a rectangle but to place objects equally on edges of rounded rectangle
I'm rewriting it with a simple rectangle right now completely so it may work now because spreading points equally seems more complicated than just to interpolate perimeter but I'm not sure how to approach arcs yet