FindMany Where Confusion
I'm trying to do a simple "find many where" query on the following schema:
TLDR: a course has a 1 to many relationship with a scheduled event.
I have these two queries:
Query 1 returns and empty list.
Query 2 returns a list with many records.
Why is it that I don't get anything back from query 1???
5 Replies
Hi @Matthew
I'm not able to reproduce this as both queries return the
Math
record. Can you confirm that the Math
record exist in scheduledEvent
and is connected to a course in your db?Hey @RaphaelEtim thanks for the help!
Math isn't a record in this case, I'm just hoping to do a do a lookup kind of like "where the title of course (a string) contains the substring of "math""
Here is some example output of the two code blocks
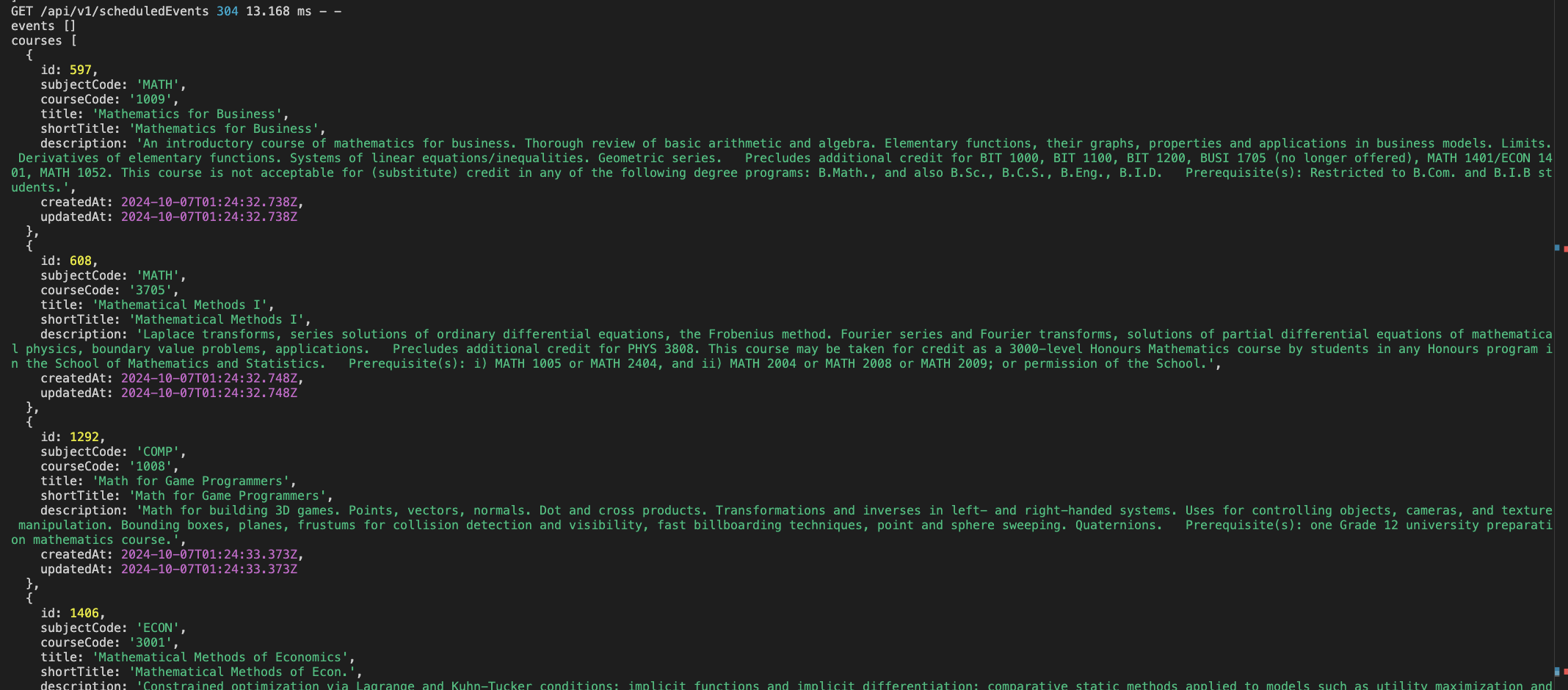
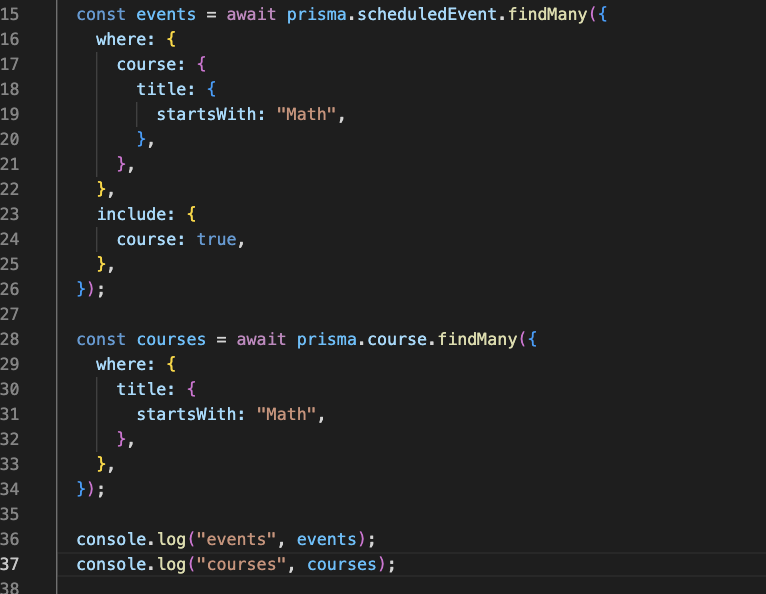
Thanks for clarifying. What does the output of the query below look like?
I'm able to filter on properties directly on the "scheduledEvent" table, but the issue I seem to get is when I want to filter scheduledEvents by data on their associated course
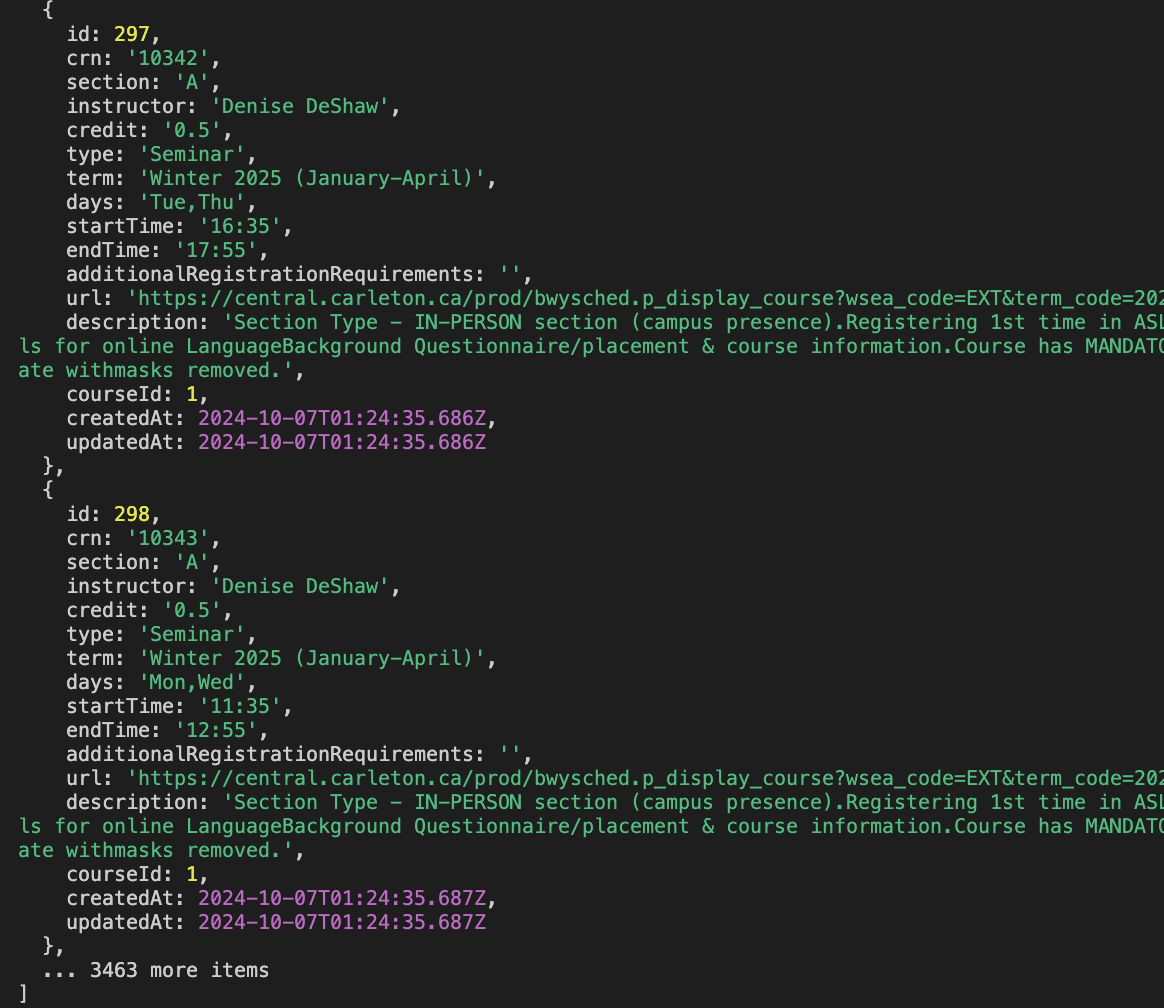
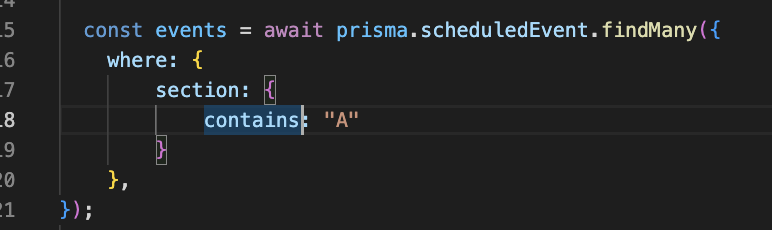
I just implemented the exact query you specified and it works
output