Problem with the container background
I'm using a ternary operator, and transparent is not working, but "#343964" is working. I was able to hide the content of the container, but not the background.
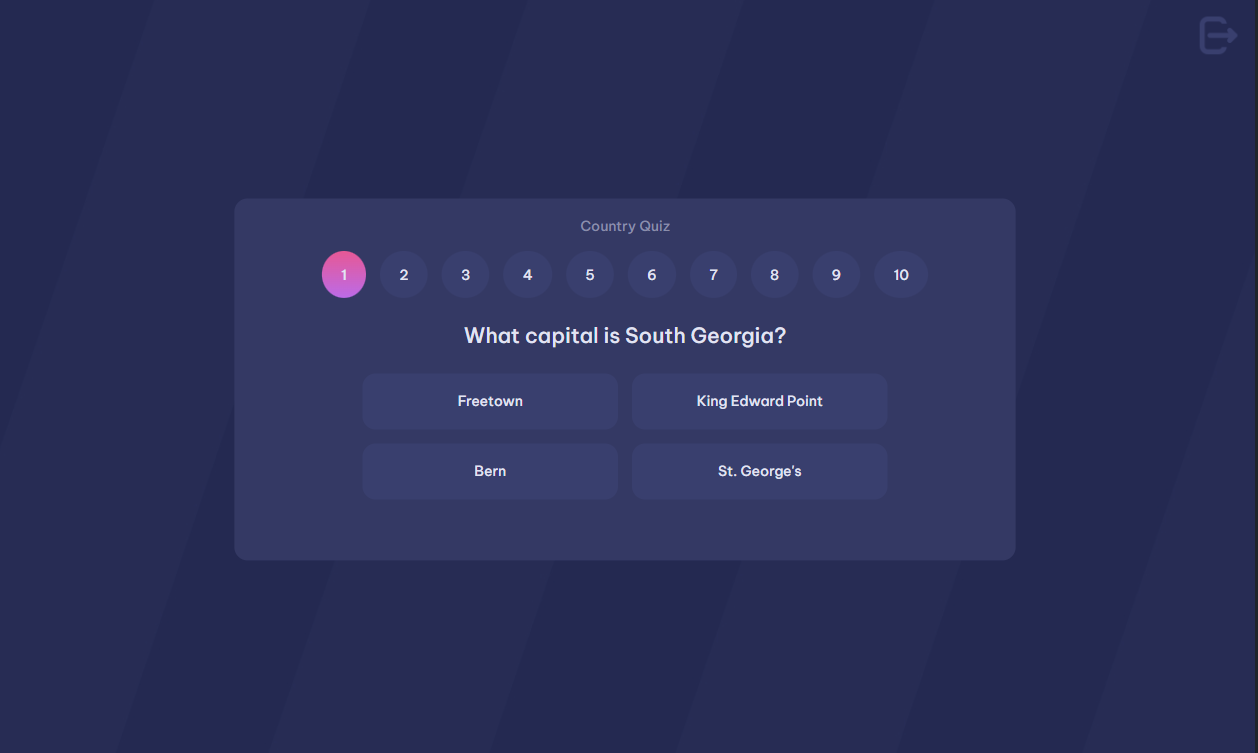
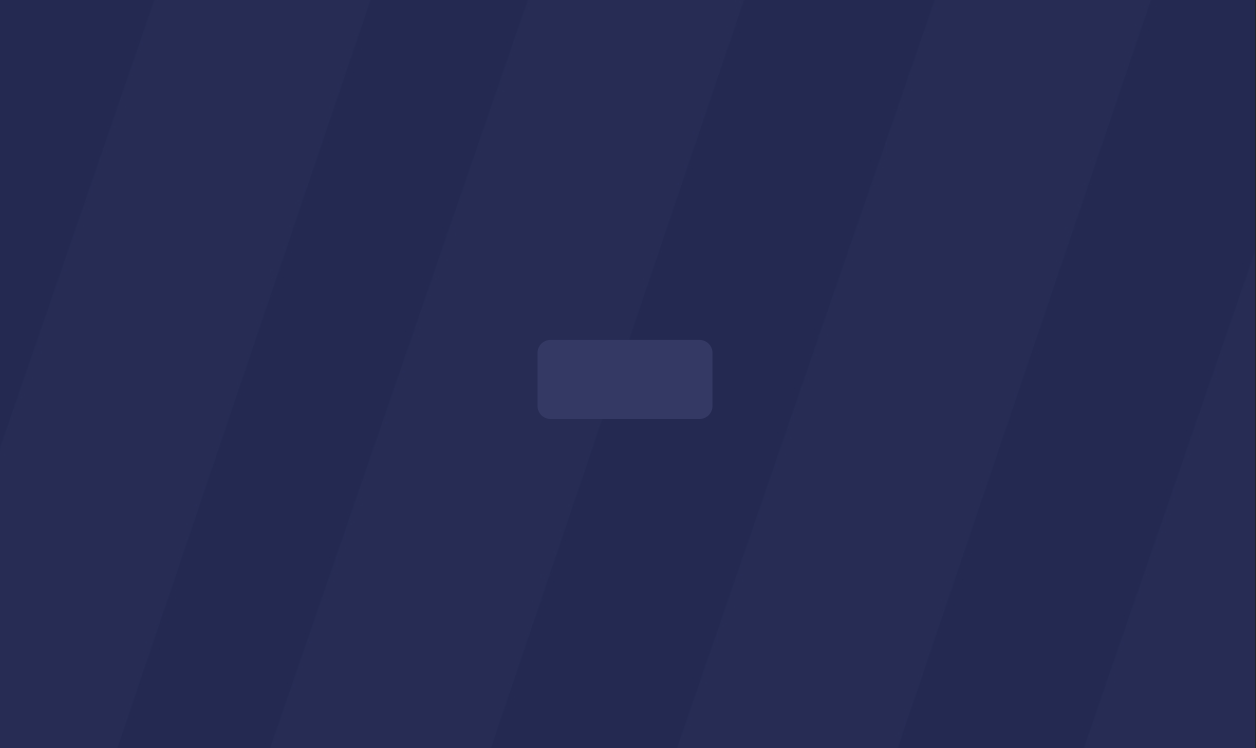
12 Replies
You can conditionally set the display property of the container when the quiz is completed:
import React from "react";
import Questions from "./Questions";
import QuizResults from "./QuizResults";
export default function Main({ isQuizCompleted }) {
return (
<div
className="container"
style={{
backgroundColor: isQuizCompleted ? 'transparent' : '#343964',
display: isQuizCompleted ? 'none' : 'block' // Hide the container when the quiz is completed
}}
>
{/* Only render Questions if the quiz is not completed /}
{!isQuizCompleted && <Questions />}
{/ Render QuizResults when the quiz is completed /}
<QuizResults isQuizCompleted={isQuizCompleted} />
</div>
);
}
You also instead of keeping the div always rendered, you can render the div conditionally based on whether the quiz is completed or not:
import React from "react";
import Questions from "./Questions";
import QuizResults from "./QuizResults";
export default function Main({ isQuizCompleted }) {
return (
<>
{/ Only render Questions and the container if the quiz is not completed */}
{!isQuizCompleted ? (
<div className="container" style={{ backgroundColor: '#343964' }}>
<Questions />
</div>
) : (
<QuizResults isQuizCompleted={isQuizCompleted} />
)}
</>
);
}
Thanks for the reply, I try that and it didn't work. It seems that it's not updating in my styles when quiz is completed.
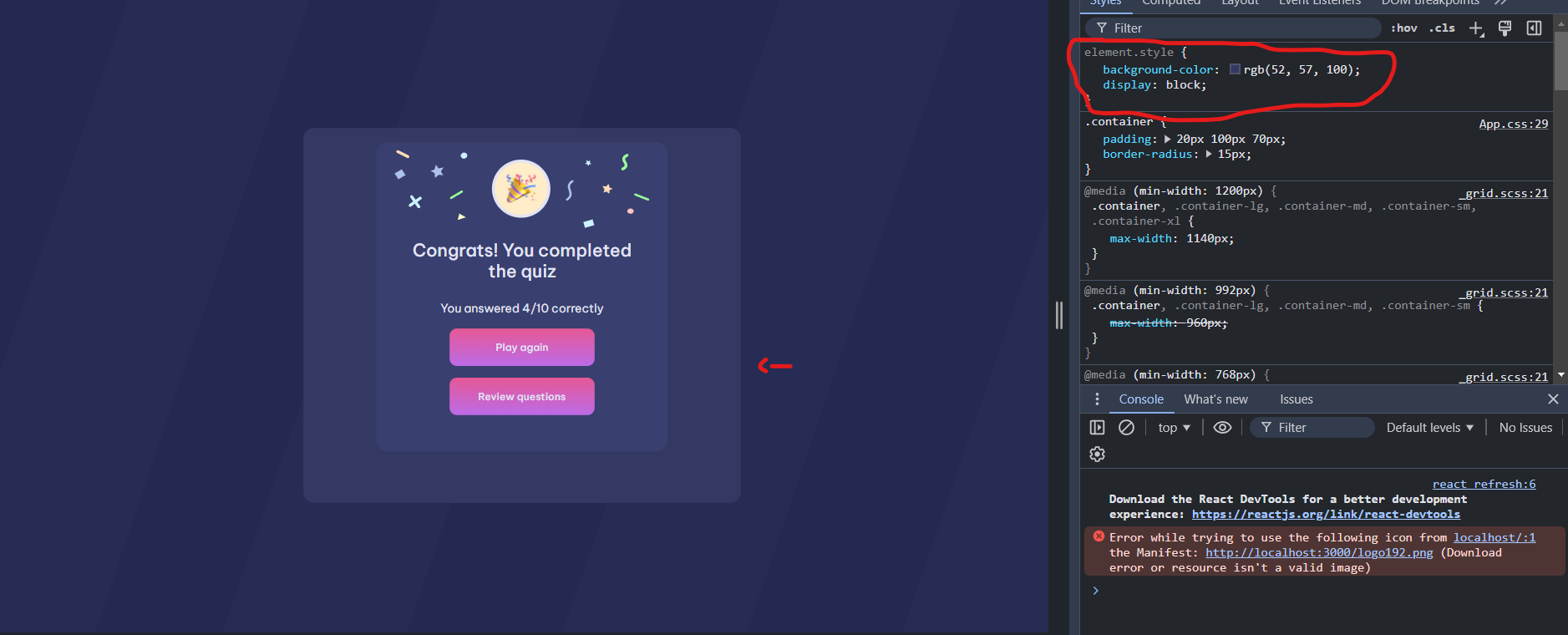
Thanks you for the update!
Have you tried the second method?
Yes, I have tried it.
Here is a link to my code: https://codesandbox.io/p/sandbox/4n6ksl
I cannot load that link, it says I do not have access.
Sorry, try this: https://codesandbox.io/p/sandbox/4n6ksl
You are never passing isQuizCompleted into Main
If the colour is working but not transparency, try changing ‘transparent’ to something else , like black to see if there’s an ancestor bg that makes it look like it’s not working or to help pinpoint where the discrepancy is coming in
But I am also confused because you are not exporting Questions but rather Quiz from Questions.
isQuizCompleted will always be undefined in his example
Thanks for the pointer, I forgot to change it due to renaming the file in the past.
It's now
in the "Questions" component.
you are still trying to pass isQuizCompleted into main but it looks like it should be a use state var, like in my example above
Oh okay, I will try that.
Thanks, it works!
And thank you! @clevermissfox @devF