74 Replies
code:
and
errors
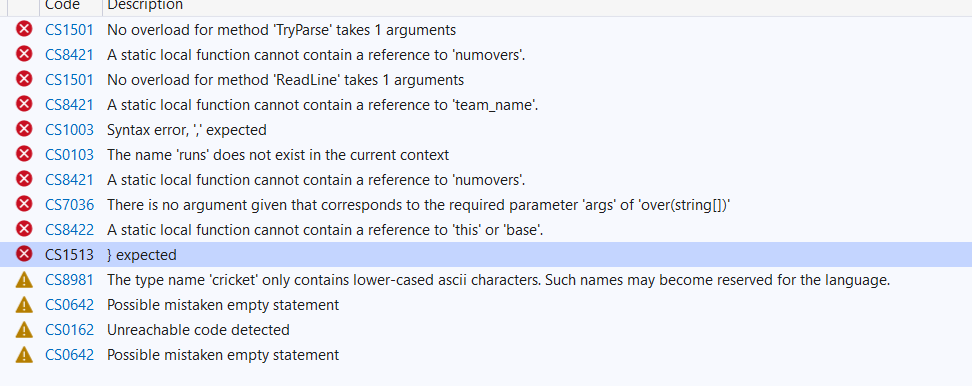
start with the first one
TryParse needs at least 2 arguments, not 1
TryParse takes in a string and an out int variable
so it'd be
if (int.TryParse(inputString, out int parsedNumber) != true) { }
you've also got a static local function referencing variables from the method it's nested within, so it can't be static.
and you're missing a closing curly brace for the over(string[] args) method
numovers doesn't even exist in the scope of the over() method
you're also using Console.ReadLine() to try and print text to the console, use Console.WriteLine() and then get the input with a Console.ReadLine() afterwards
and you'll need to parse that input string as an integer before you can assign it to an integer variable
and remove the semicolons at the end of your if statementsah
i see
sorry for taking a while to respond
wait
i dont have any output though?
i just want to check if its an integer
what am i supposed to use as output
use a discard then
...idk what that is but ill check the documentation
like this
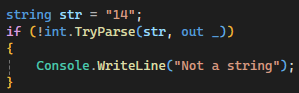
alright
?

the first argument should be a string
oh right
int.Parse and int.TryParse try to parse strings as integers
is there any way to just
check if any value
integer or string
oh wait no thatd be impossible
ok is there a way to check if
anything other than a natural number is entered
so any integer greater than 0
no letters, no decimal numbers, no negative numbers
you could use an unsigned integer
uint
as a parameter because they can't be negative, but casting a negative integer to them will just cause some unexpected behaviour if it was negative
or you could do if (myInteger < 1)
using unsigned types to enforce ranges isn't a great idea, it's better to do an actual range check
so you check if it's an integer first, then check if that integer is greater than 0
ya but then that doesnt check for like characters
i can check if the integer is greater than 0 thats fine
e.g.
i was just wondering if there was something that just auto checks if its anything OTHER than a natural number
ill try this
var just automatically changes the variable to whatever makes the most sense right?
yeah, it's exactly the same as if you put int there instead
it's just obvious that it will be an int already so no need to repeat it
so should i imput numovers
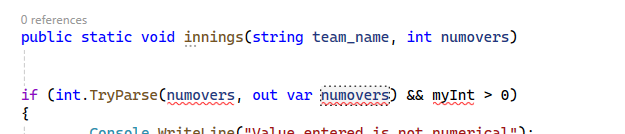
as a string
ykw itd be easier if i showed you what im trying to do
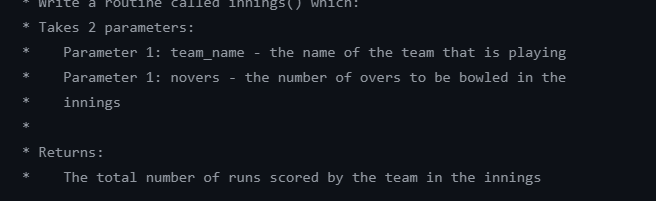
if your method receives an int then you already know it's an integer
there's nothing to parse
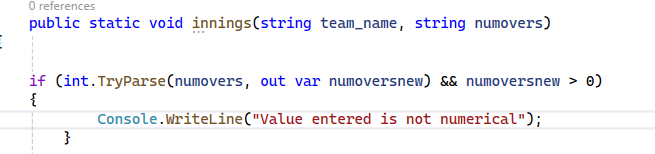
so... this?
that would be good if you're supposed to get a string, yes (the logic is inverted though)
the text you shared doesn't seem to specify what type
numovers
should bei just need a numerical value that i can work with later on
for this
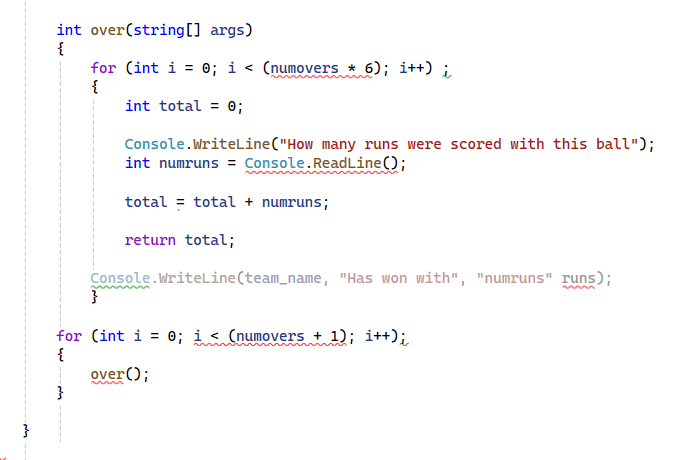
yeah its honestly pretty poor 😠they expect us to write a cricket program but nobody knows how cricket works
and im here trying to decipher my code AND the rules of cricket but whatever
so if it was me, i would separate the user input part and the actual calculation part
so have some other code that reads the input and converts it to an int, then pass it to this method and do all the actual number stuff
but thats not the issue
they kinda expect me to have input validation in the program
unless im just not understanding what youre saying
well nvm that
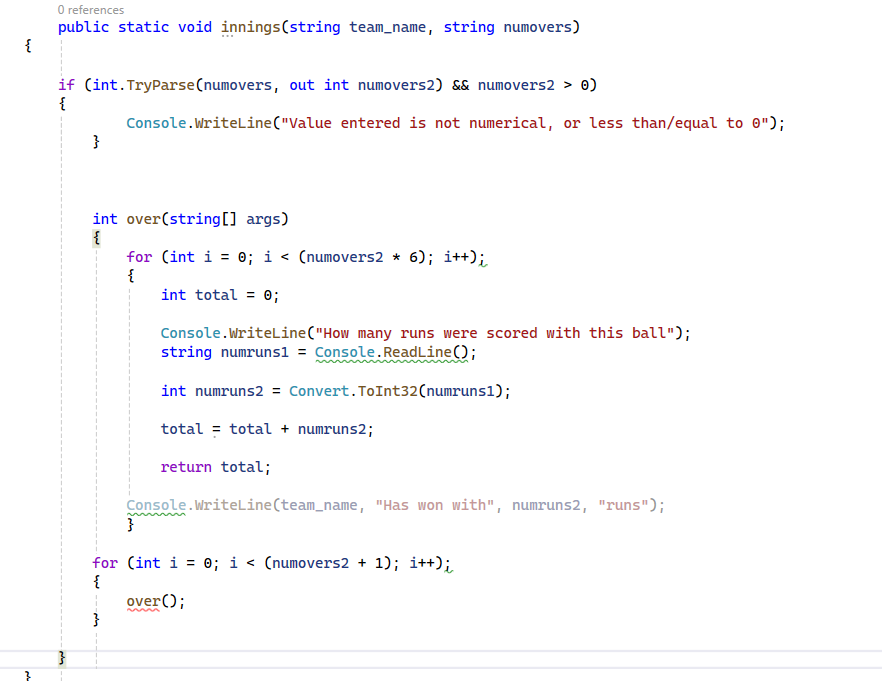
i fixed some of the code but
i cant seem to run my subroutine
actually ig this is better??
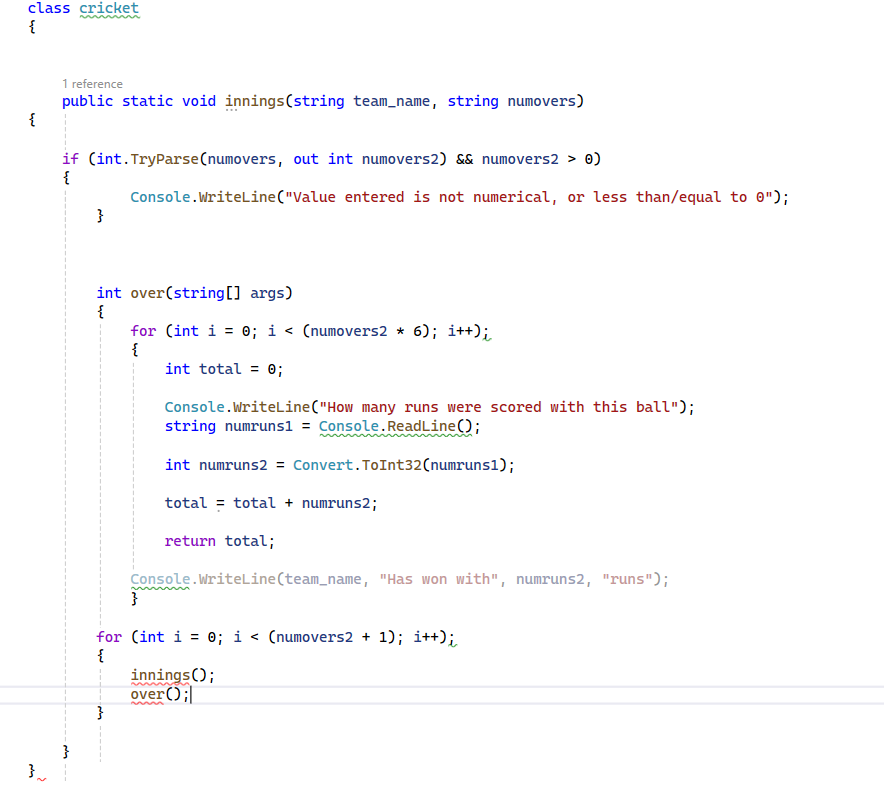
brackets are all over the place. recommend formating and lining up first. Also I dont think you intend to recursively call innings?
what even is string[] args in context of the over function?
i practically rewrote my code hold on
no clue. i just have it there because someone told me to one time
i barely know how c# works ðŸ˜
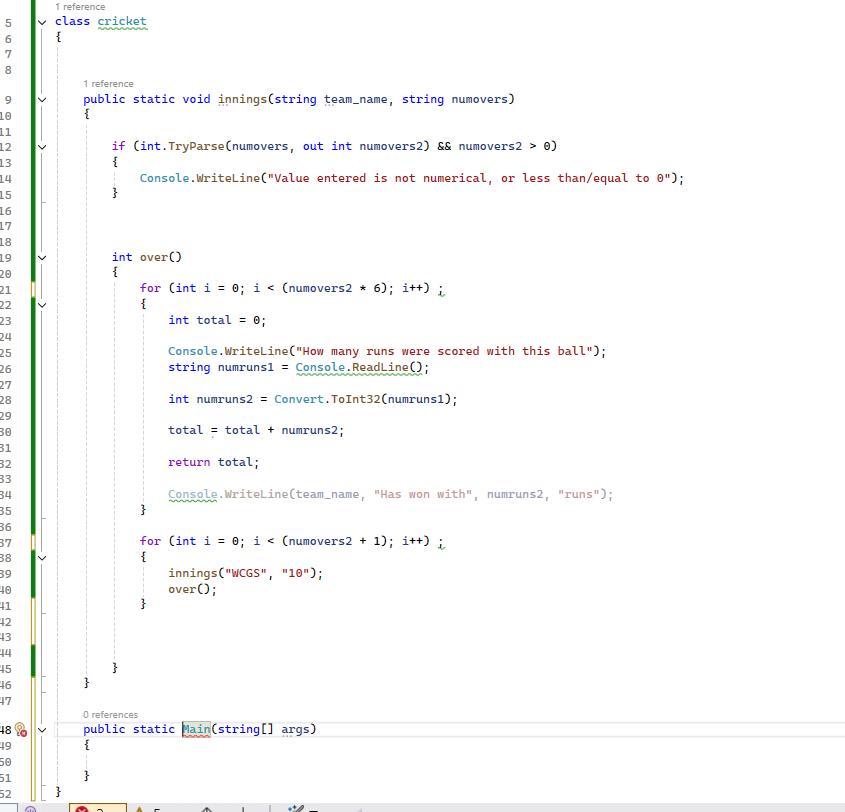
yeah I recommend learning the basics first, learning the syntax etc.
oh i know the syntax
im overexaggerating
i just like
dont know anything other than basic functions
like arithmetic, printing, reading input, calculations, string functions, loops etc
i can do all of that
its just the complicated stuff
also if anyone is still around i kinda realise now i need to input multiple teams and display the winner but idk how to even start that
i would recommend white boarding out your solution before writing any code
expected inputs, logic that needs to be done, and output
i mean if you mean for what i just said yeah i am considering that
i need to loop the functions so that i can enter more than one team name and number of overs
and i need to store it somewhere
so that the highest value can be printed later
its just that like
idk how to go abt that
it's easier to break down the problem into mini problems
first, what are your expected inputs?
oops sorry for disappearing
ok so
team_name
and
numovers
numovers will be used to calculate numruns
which loops to get a total
oh wait
i think i kinda see the issue
see
my school expects me to input the team name and the number of overs like this

but thats just the problem
if i write it like that I can't loop it over
so maybe instead of a for loop, you can have your application running with state machine logic. ie while loop.
so that user can running the logic for firing that inning function based on a certain criteria whenever user wants
and exit the program whenever as well
hm
so if i start like
the entire program
with a while loop
maybe linked to an input
and if the user like
inputs
just as an example
H
the program will stop
and return the largest score value?
so on init, have something like
Hi user. press following button to do stuff.
1. Do Foo
2. Exit
and 1 will direct them to input whatever they need "team name", "number"
and will output that number, then direct them back into the main while loop
ok
that works
small issue though
how do i like
hold the score value
if that makes sense
like lets say they do the inputs
program runs
and returns like
20
how do i take that 20
store it
and when the program stops running
i compare all the input values
and print it out
the easiest way is to put it in memory during application runtime
how would i go about that
a way to do this would be a static integer, something like
alright
ill try and implement this
so like this???
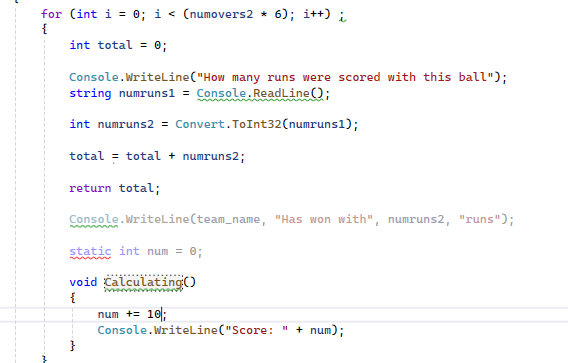
but this would just add 10 each time
i need it to like
make a new variable???
no
to
store a number
compare a newly inputted number
and output it???
idk
man
ill send my entire code so u can see where its at atm
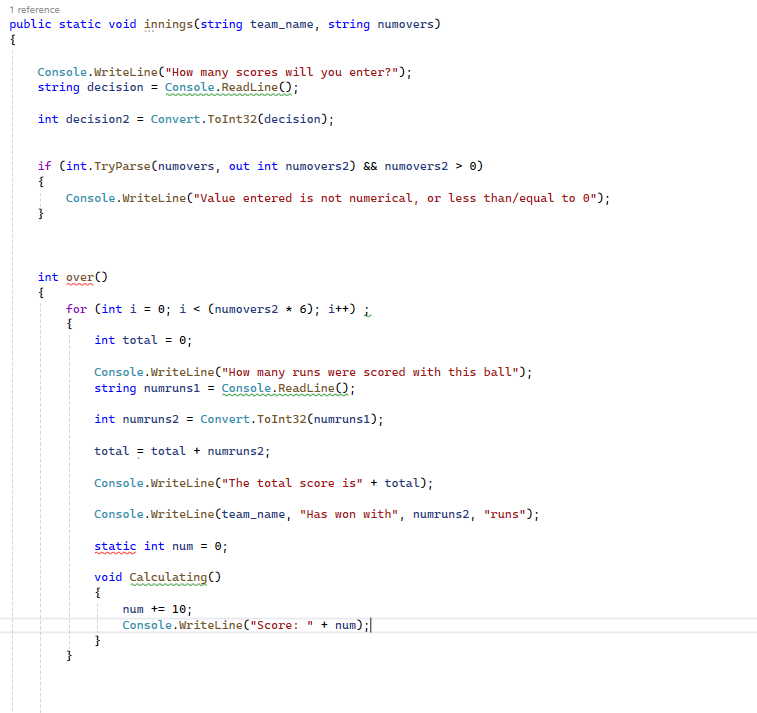
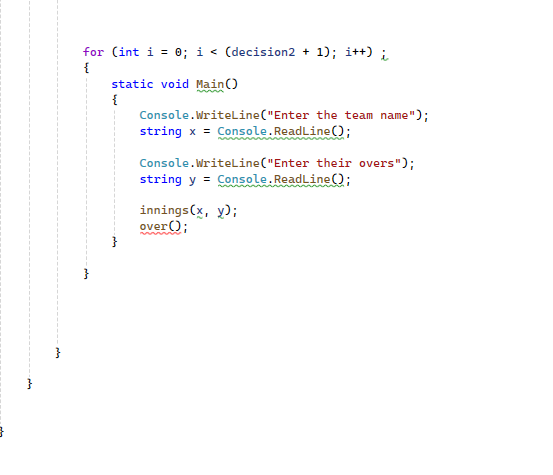
i probably shouldnt put the static integer there
lets work on getting the application flow working first, being able to run the project helps. Personally, i would recommend creating a new console project and getting the while loop going
mk
ill just try an output a single score for now
one small issue
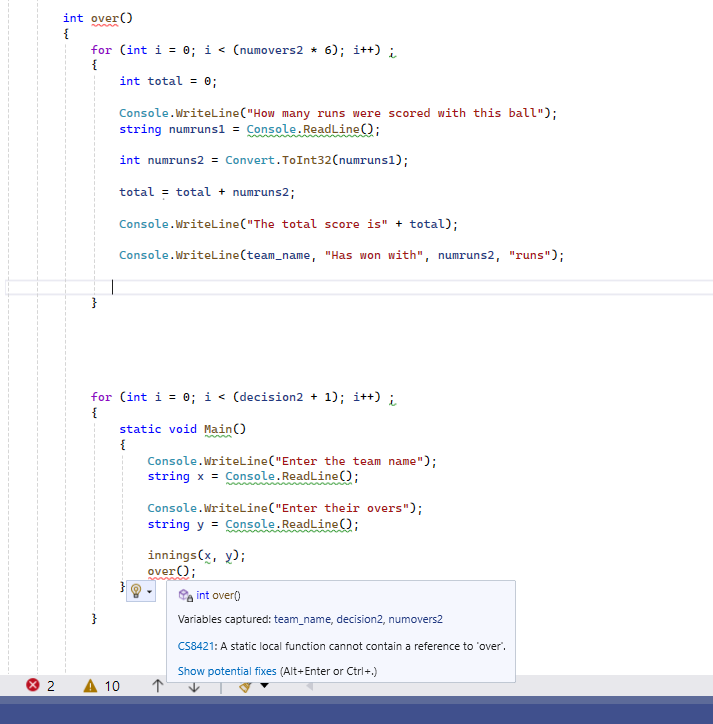
well the flow is all messed up in the first place, which is why i recommended creating a new projet
oh..
its getting late for me though so
do yk how i can fix it? ill just fix that error go to sleep and get back to it later
or just hand it in incomplete because disregarding the programming the task was explained vry badly by my teacher
you can put keyword "static" right before
int over()
but your program might infinitely recurse and crash due to stack overflowoh...
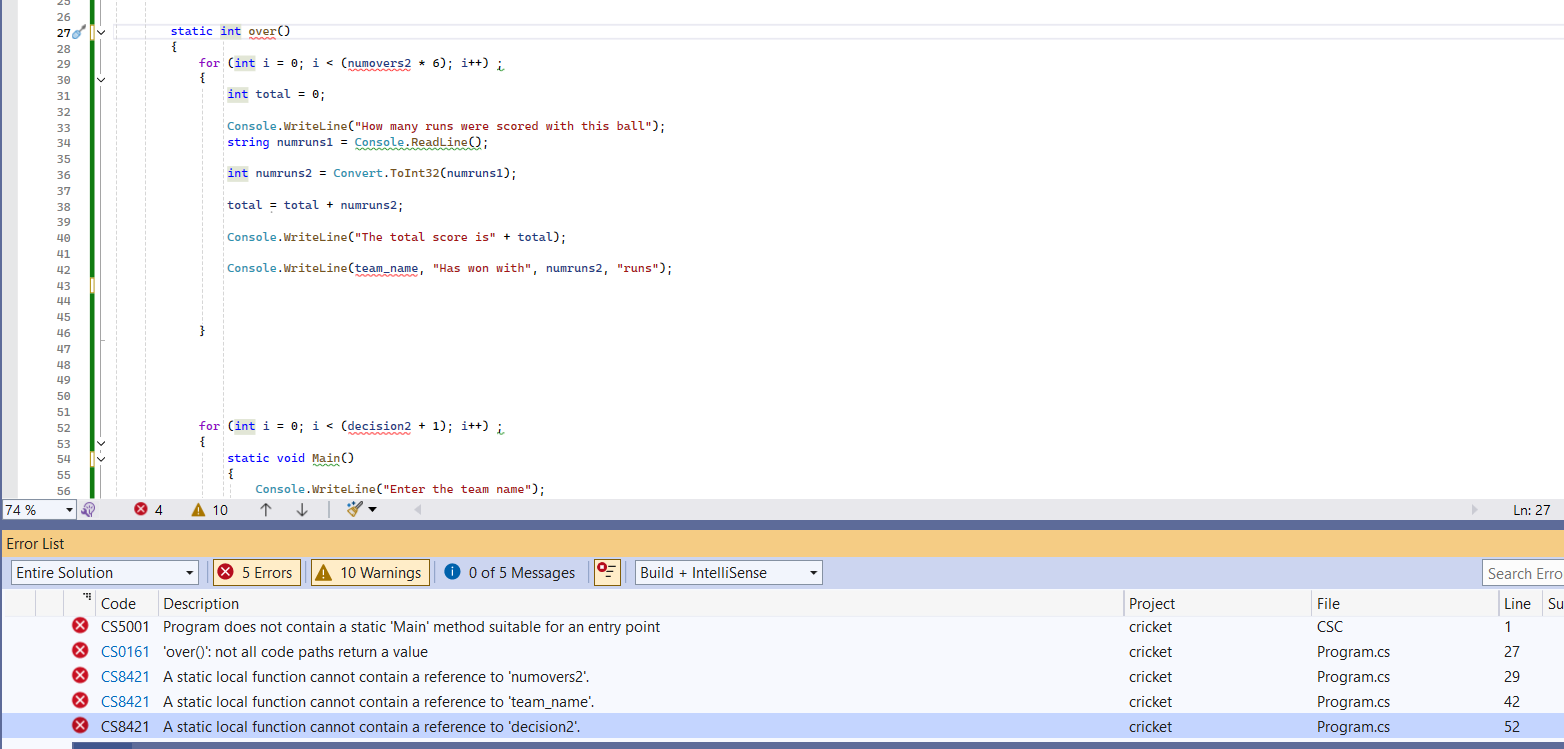
if i do that i get these fun errors
yeah there isnt really an easy fix, just rewrite imo
ah
mk
This would be the skeleton for your application flow, nothing too complicated.
This conversation is very long so I'm just going to comment on what I see
Don't use
Convert
. Use int.Parse
to parse a number. Even better, use int.TryParse
to safely parse the number and check if it's a number to begin with. Your initial post tried to use it so I don't see why you switched. Convert
is legacy and even if you're definitely only passing numbers you should check regardless.
Your methods are also completely misplaced Main
is inside over
which should not be the case. You rarely need nested loops, and your main method should definitely not be nested. Unnest it and it can call over
Other than that the errors relate to variable snot being defined. Where is it supposed to get numover2
and team_name
and such from?oh i was using convert bcs i needed it to be an integer
at that point
that wasnt to check
ah I see
