Partial classes and platform specific code
Hello. Trying to create platform specific code and only use/compile the code for correct platform upon release. My current issue is that regardless of platform my linux specific code is trying to run on windows. I seen examples of it using MAUI, however i'm just trying to make a simple console app.
In my csproj:
Base class:
used like this:
an identical implementation is done for linux and all code is in the same namespace
and it's built using this command:
dotnet publish wallpaper.csproj -r win-x64 -c Release
20 Replies
Thought partials would be a good use case for this, but cmiiw and if a easier way exists
https://learn.microsoft.com/en-us/visualstudio/msbuild/property-functions?view=vs-2022#msbuild-property-functions:
Specify whether the current OS platform is platformString. platformString must be a member of OSPlatform.https://stackoverflow.com/a/65289488/1086121 suggests the thing to do is to check on
$(RuntimeIdentifier)
? Not sure whether there's anything more specific
Also https://github.com/dotnet/msbuild/issues/5065#issuecomment-2271062217Thanks for the help. Just checked out that github and tried the below and it now works on windows but not linux. Gonna look at some more stuff now that you linked
sorry to expand on it I now get the below error:
Partial method 'Platform.SetWallpaper(string)' must have an implementation part because it has accessibility modifiers.
Yepyep. Remember that not-implemented partial methods are removed from the caller. That can only happen if the caller is in the same class
Hmm. So would that mean that the condition is not met in csproj, therefore the compiler removes the code (implementations) specified using #if? (Expected if csproj is wrong) Or is it now correct and that my actual code is wrong.
If you have a partial method without a body, it must have 0 access modifiers
So your code is wrong
Just looking at this article
it seems like its okay to define it with access modifier? Sorry still learning
https://learn.microsoft.com/en-us/dotnet/maui/platform-integration/invoke-platform-code?view=net-maui-8.0
.NET MAUI invoking platform code - .NET MAUI
Learn how to invoke platform code in a .NET MAUI app by using conditional compilation, or by using partial classes and partial methods.
Ah, maybe I'm wrong
ur good. I think it points back to the csproj being wrong again, but im not sure.
The difference between setting it in the .csproj and setting checking it at runtime is 1 has runtime overhead (though minimal) and the other doesnt.
You could when publishing for Linux or Windows include the constant in there if you want
dotnet publish -p:DefineConstants="LINUX" --os linux
Additionally for code like the platform code you specified, you could create a similar interface then declare a platform specific implementation of that
eg.
then you can write up an implementation for each platform like
For checking at runtime, you could do
or
Thanks for the example code. I ended up doing it at runtime pretty much how you showed here. My only question is that do u think originally that publishing it using the args you provided would have worked? I'd go back and try but didnt use git
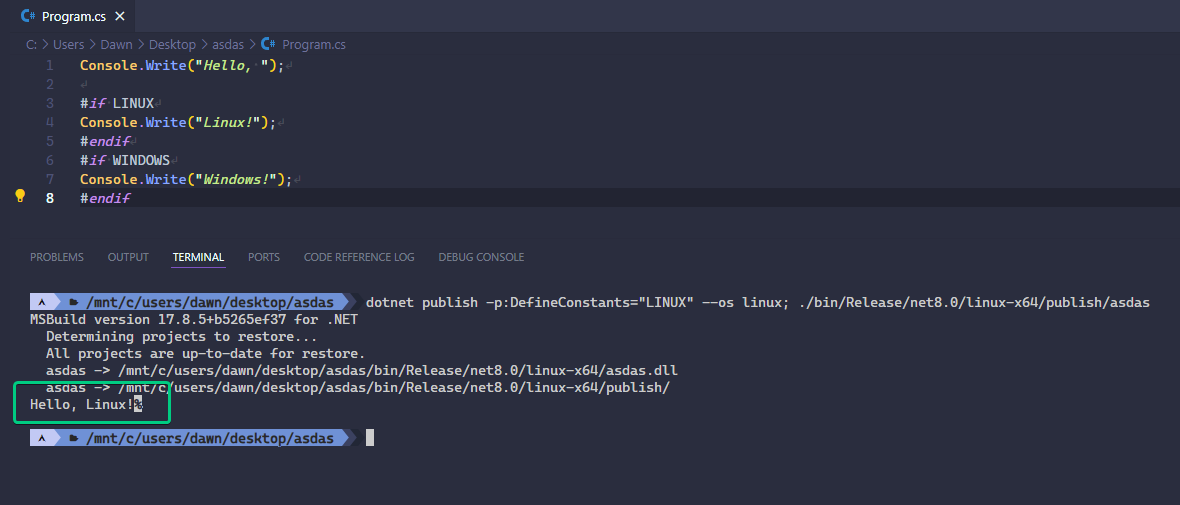
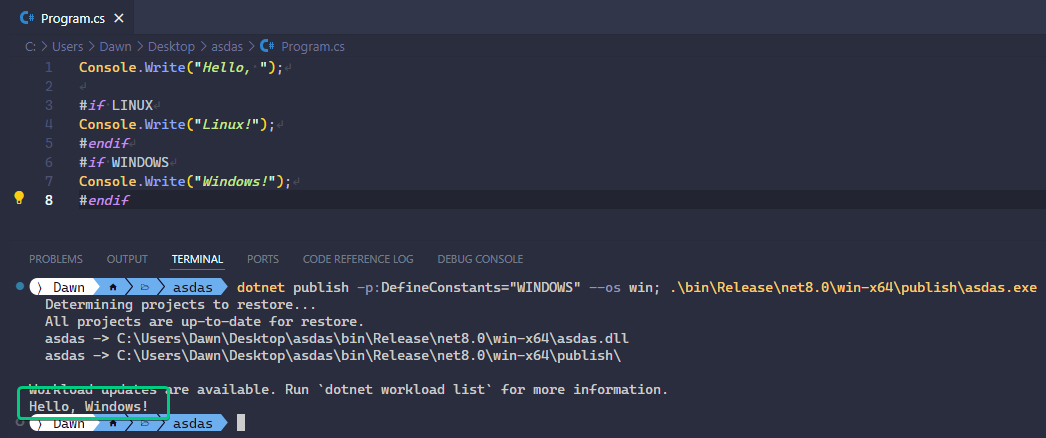
If neither constant is provided, it will just write out
Hello,
Unknown User•7mo ago
Message Not Public
Sign In & Join Server To View
If you can provide an example that would be great, just looking at it seems too complicated for this use case
I was mainly talking about in my specific example with partial classes, thank you for the test though 🙂
Unknown User•7mo ago
Message Not Public
Sign In & Join Server To View
will take a look, thanks
so i took a quick look and this only seems to apply to ide/dev ux and warnings and does not affect compilation
Unknown User•7mo ago
Message Not Public
Sign In & Join Server To View
You are right I should use one of attributes provided, but I believe that is a seperate issue to my original problem. In regards to the bad msbuild, are you saying that it takes the host OS when building and not the target os? So instead of using IsOSPlatform I would use something like Condition="$(RuntimeIdentifier.StartsWith('win'))" instead, like a commenter above pointed out. However this just brings me back to the error of "Partial method 'Platform.SetWallpaper(string)' must have an implementation part because it has accessibility modifiers." correct? My goal was to use partial classes and conditional compilation similair to maui example. I do appreciate everyones help though 🙂
And yes just for something simple I think we have established that conditional calling based on platform is probably the best bet, but I would still like to see why this wasn't working for me.
Unknown User•7mo ago
Message Not Public
Sign In & Join Server To View