Message Collector help
Hey all,
Im having some issues with message collectors in DM's.. i cant get the bot to actually collect anything at all when the user replies to the bot's dm?
is it a code error or something else?
18 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPUnknown User•4mo ago
Message Not Public
Sign In & Join Server To View
[email protected]
v22.8.0
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
😢 thank you
So im assuming this should now work
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
Hmm, still nothing, even with the intents and partials update 🤔
ive moved them over to my veriy.js also..
but im not passing client anywhere in the file... guessing thats my issue ?
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
hmm, im confused to why this isnt working then
the collector starts and ends without issue, but doesnt actually collect
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
got a few other commands using it;
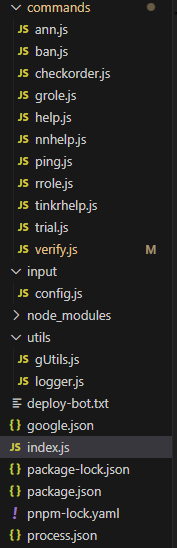
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
command handler is using the message partal;
code is saved and console is logging collector started and collected ended, but nothing in between
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
yep... you are correct, ive deleted that partial and collectors are now working
ive been sat here for 2 hours with this lol T_T
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View
thank you so much
Unknown User•4mo ago
Message Not Public
Sign In & Join Server To View