need help with this code
Hey, I’m tryna make a bot that checks for a specific keyword within someone’s custom status and if they have the keyword, it gives them a role, and if they don’t, it removes the role. The code is semi working, it’s logging in the console every time someone changes there status but isn’t logging when it is the correct status. and removes the role even if it’s the wrong or right status.
19 Replies
- What are your intents?
GuildVoiceStates
is required to receive voice data!
- Show what dependencies you are using -- generateDependencyReport()
is exported from @discordjs/voice
.
- Try looking at common examples: https://github.com/discordjs/voice-examples.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
That is my code
Also I just noticed I posted this in the wrong area 😭
The newest version, just installed it again today. And all the logs is when someone changes there status, and when it removes the role
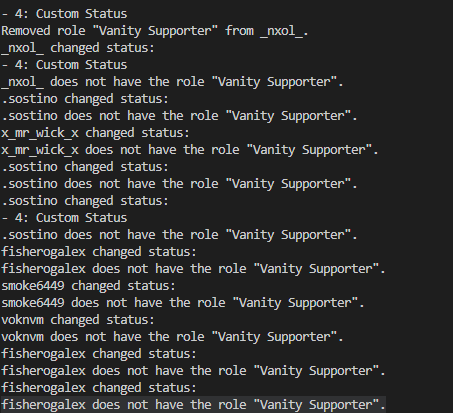
wdym
im still new to this stuff 😭
so how do i fix it
alr
Still getting the same issues, nothing really changes, still also showing “4”
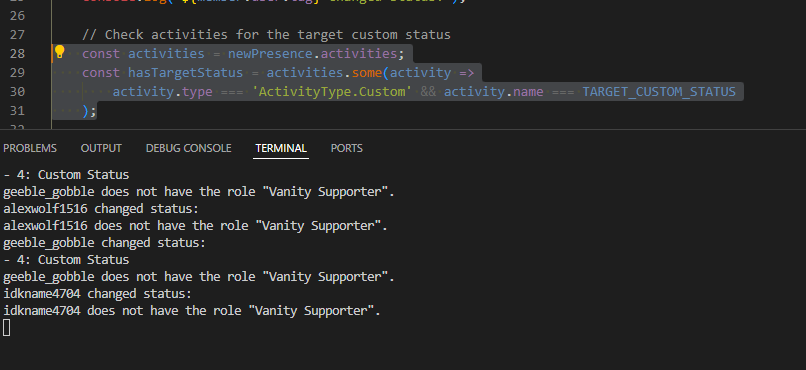
I have the right status set so in theory it should give me the role and log it not say ion got the role
Still no change. 😭
Sorry to bother you
If you aren't getting any errors, try to place
console.log
checkpoints throughout your code to find out where execution stops.
- Once you do, log relevant values and if-conditions
- More sophisticated debugging methods are breakpoints and runtime inspections: learn moreQjuh told you that activity.type is a number but you are still comparing it to a string, you stringified the enum
Yeah but how to fix?
Do not put the enum in strings, use it as is, import the
ActivityType
enum from d.js, you should know by now how to use enums
If not, then #rules 3so a vanity bot checker
Yh
bio or statues
Status
this is legit chat gpt code lol
let me fix it rq 1 sec
@gblazzy do u even know how to code or
Yh I ran it through GPT to try and find the mistake
ohh alr
i remade it works nowo
anyways gl cya