ā Making a chess program. Why is this happening?
I don't understand this error and nothing online makes sense to me, so can I get a firsthand explanation of what I've done wrong? Or probably done wrong?

36 Replies
You've got another
public struct Move
somewhere in your code, in that same namespacethis is the only other search result when searching for
public struct Move
in vscode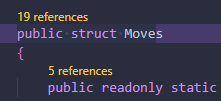
Search for
struct Move
?
Are you using source generators anywhere?same result
whats a source generator?
I'm guessing probably not then. Can we see the whole definition of
Move
?GitHub
chess/MoveGeneration.cs at beta-testing Ā· axololly/chess
A chess bot I made over the summer because my friends didn't want to go out with me. - axololly/chess
Not found: is your project private?
wait
no its public i just got the link wrong
on mobile rn
ok works now
missed the
tree
part of the URL
There we go. That
GeneratedRegex
attribute is a signal to a source generator to generate the other part partial part of that method at compile-time. See https://learn.microsoft.com/en-us/dotnet/standard/base-types/regular-expression-source-generators?pivots=dotnet-8-0
And for Move
to have a partial
method, it needs to be partial
itselfcan i make the method not partial? or does that break stuff?
It has to be partial in order for the
GeneratedRegex
machinery to work, see that link
If you don't use GeneratedRegex
then you don't need partial
ah ok. how do i use regex in C# without
GeneratedRegex
?Just
new Regex(...)
?ah okay
oh yeah thats why i did this in the first place
Use 'GeneratedRegexAttribute' to generate the regular expression implementation at compile-time.
Yeah, it's prompting you to move to the source generator, which does produce a regex which is quicker to run at runtime
(but does require
partial
, because it adds code to your class at compile-time)should i keep it so it happens at compile time instead of runtime?
You can ignore / disable that prompt if you want
It probably doesn't matter a huge amount either way
alright
oops
(since your regex is quite small and simple)
fair enough
Btw, you can do
value[1] - '1'
rather than "12345678".IndexOf(value[1])
. That avoids a linear search through the stringoh wait what
you can subtract numerical versions of strings?
You can subtract
char
soh wow thats awesome
thanks canton
(It's nothing to do with numbers stored as strings. Your
"abcdefgh".IndexOf(value[0])
could also be written value[0] - 'a'
)oh wow thanks man
another question just quickly
im using bitboards for my program. i mention this because i need to use bitwise operators on the struct. i also want to use
&&
on the struct too
i have this example code
and i want result
to be true
if ex1
and ex2
are non-zero values
but this doesnt work
removing the overload for &
and |
makes it work fine tho
wait
oh yeah
if i overload it so that true
(operator) returns ex.Value == 0
and false
(operator) returns ex.Value != 0
that works just fine
wait
nevermind i fucked that upWhat was the solution? I'm failing to spot it ><
if i overload it so that true (operator) returns ex.Value == 0 and false (operator) returns ex.Value != 0 that works just fineIn my reading of https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/true-false-operators, you've defined it correctly though? And if you flip the polarity of your
true
and false
operators, then new Example(1) && new Example(0)
returns true
ohh. if i did it correctly then theres no problem
also
this is part of a print statement
and it prints an empty bitboard
despite that literally being a boolean
Yeah, but I would expect output which is different to what you're getting, so I still don't understand what's going on
Oh, idiot
It's because '5 & 2' is 0.
So yes, your initial code does produce the correct result
Because
&&
is a short-circuiting &
ohhh
š¤¦āāļø
so how do i fix that thne
You're trying to define
&&
and &
to have different semantics, but that's not how it worksTbh, get rid of all the implicit crap and make some methods
It'll be a lot more readable in a week/month, trust me š
unsettling emoji
im too deep in the rabbit hole
so you're saying i can't have
&&
and &
do different things?
alright
i'll do this insteadBut, if you get rid of the implicit stuff, that's
kingMask != 0 && enemyQueenRook != 0
. If you wrap things up a bit, that's !kingMask.IsEmpty && !enemyQueenRook.IsEmpty
And IMO !kingMask.IsEmpty && !enemyQueenRook.IsEmpty
is clearer than (bool)kingMask && (bool)enemyQueenRook
oh yeah i forgot i can do an
.IsEmpty
method. will do that šš½